How to Include a Class Into Another Class in C#
-
Use
using <namespace>
to Include a Class Into Another Class inC#
-
Use Inheritance to Include a Class Into Another Class in
C#
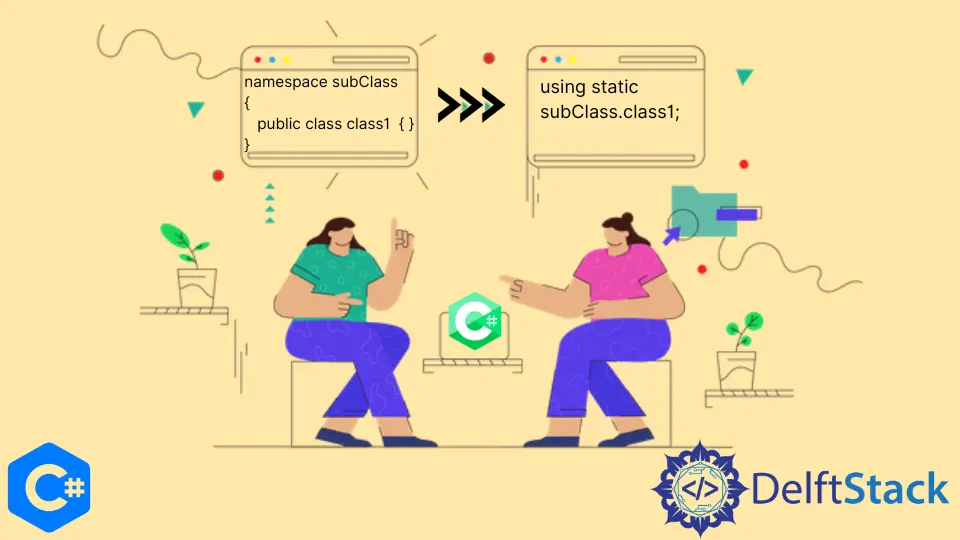
In this tutorial, you will learn the different methods to include a class into another class in C#. A class as a user-defined blueprint or prototype contains objects and methods that can be called within another class.
Including a class in another class allows a class to call methods and objects from another class. This feature enables the user to technically group classes and creates more readable and maintainable code.
Use using <namespace>
to Include a Class Into Another Class in C#
A namespace in C# is a logically arranged class, struct, interface, enum, or delegate. Namespaces in C# can be nested, meaning you can define a class and include it in another class.
A class in a specific namespace and a class from another .cs
file can be called by using <namespace>;
. The members of a class are accessible using the dot(.)
operator when defining it into another class, like using <namespace>.<method>
.
// WelcomeClass.cs
namespace SubClassNamS {
public class WelcomeClass {
public static void Welcome() {
// To show a message box when this method from `WelcomeClass` will be called by another class
MessageBox.Show("Hello World!", "Test Subject", MessageBoxButtons.OK,
MessageBoxIcon.Information);
}
}
}
The WelcomeClass
class in a C# project is a part of the WelcomeClass.cs
file. It contains a Welcome()
method.
The namespace SubClassNamS
is a reference to this class you can use to include it in another class.
The Form1.cs
is a form in C#, which contains only a button1
button. The WelcomeClass.cs
and Form1.cs
are a part of a same C# project and WelcomeClass.cs
classes can be called by classes from Form1.cs
using the namespace.
// Form1.cs
// Use `using` Namespace of another class from a different file
using static SubClassNamS.WelcomeClass;
namespace IncludeClass {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
// Button `Button1` click event
private void button1_Click(object sender, EventArgs e) {
// No need to mention `WelcomeClass` here
Welcome();
}
}
}
Including SubClassNamS.WelcomeClass
enables us to access WelcomeClass
from the SubClassNamS
namespace.
This method helps you import or include a WelcomeClass
class into the Form1
class by introducing its methods and members using namespace in C#.
Use Inheritance to Include a Class Into Another Class in C#
If two classes are from the same .cs
file in C#, we can simply include a class into another class using [class].[method]();
. It is possible to introduce methods and elements from one class to another using Inheritance.
The concept of class inheritance consists of a Derived
or child class that inherits from another class called Base
or parent class. Inheritance makes importing a class tricky but enables us to take advantage of the methods of both classes by calling only the parent class.
using System;
// Parent Class
class Rocket {
public string Type = "Rocket";
public void bang() {
Console.WriteLine("Boom, Boom!");
}
}
// Child Class
class Celebration : Rocket {
public string RocketModel = "HappyJones";
}
// Main program
class Program {
static void Main(string[] args) {
Celebration Air = new Celebration();
Air.bang();
Console.WriteLine(Air.Type + ": " + Air.RocketModel);
}
}
Output:
Boom, Boom!
Rocket: HappyJones
Create Air
as an object of the Celebration
class, which can access both the elements of Rocket
and Celebration
classes. In C#, the Air
object from the derived class helps import two different classes into the Program
class using inheritance.
You don’t need an import
statement or using
namespace if both classes are from the same namespace. The easiest way to include a class into another class is by creating an instance of the TecClass
class and calling its member functions from the MainClass
class.
using System;
public class TecClass {
public static void CallMethod() {
Console.WriteLine("Hello World");
}
}
class MainClass {
public static void Main(string[] args) {
TecClass.CallMethod();
}
}
Output:
Hello World
The most optimized way to include a class into another class is by creating a separate .cs
file for a class and calling it by using <namespace>;
. Use using static [namespace].[class];
to include the static members of a class into the main class.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub