Interface vs Abstract Classes in C#
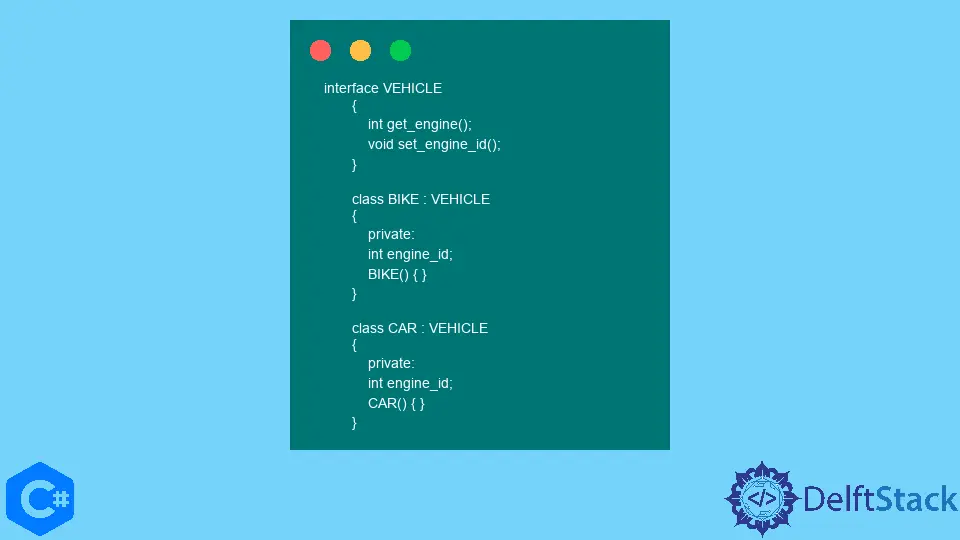
Classes have different specifiers in C#. Today we will be talking about two classes; Interface and Abstract classes.
Difference Between Abstract and Interface Classes in C#
An interface is something that defines how something should work. It is required to provide a guideline or a basic setup on stuff and communicate between objects.
An interface only implements methods or tells which functions are to be made or are required. It will only provide the basic setup; the rest is on the class that inherits it to implement and extend these functions as needed.
So if we had a CAR
class and a BIKE
class, they could inherit the interface VEHICLE
that could contain common functions to both of these classes (CAR and BIKE)
such as; get_engine_vid(), set_color()
etc. The VEHICLE
here would act as an interface.
You see how an interface helps maintain a standard definition protocol within classes. All methods declared inside the interface are and should be extended.
Example Code:
interface VEHICLE {
int get_engine();
void set_engine_id();
}
class BIKE : VEHICLE {
private : int engine_id;
BIKE() {}
}
class CAR : VEHICLE {
private : int engine_id;
CAR() {}
}
This code will show an error.
To fix it, we’ll implement methods in each inherited class in the following code.
Fixed Code:
interface VEHICLE {
public int get_engine();
public void set_engine_id(int id);
}
class BIKE : VEHICLE {
private int engine_id;
BIKE() {}
public
int get_engine() {
return engine_id;
}
public void set_engine_id(int id) {
engine_id = id;
}
}
class CAR : VEHICLE {
private int engine_id;
CAR() {}
public
int get_engine() {
return engine_id;
}
public void set_engine_id(int id) {
engine_id = id;
}
}
Now that you are clear on interfaces let us look at abstract classes in C#.
Example Code:
abstract class VEHICLE {
public abstract int get_engine();
public abstract void set_engine_id(int id);
}
class BIKE : VEHICLE {
private int engine_id;
public BIKE() {}
public
override int get_engine() {
return engine_id;
}
public override void set_engine_id(int id) {
engine_id = id;
}
}
class CAR : VEHICLE {
private int engine_id;
public CAR() {}
public
override int get_engine() {
return engine_id;
}
public override void set_engine_id(int id) {
engine_id = id;
}
}
static void Main() {
CAR n = new CAR();
n.set_engine_id(2);
Console.WriteLine("The engine id is: " + n.get_engine());
}
You can see how override
tends to be set here to extend the functions declared in the abstract class. So what is the difference?
Abstract classes are used for multiple classes with the same base structure, but an interface may just be used to define a method that can be implemented. Using it for multiple classes that may or may not inherit such a structure is unnecessary.
Abstract classes are also able to define default implementations of methods. However, this is not the case with interfaces.
You need to remove override
for it to work perfectly.
Fixed Code:
abstract class VEHICLE {
public int get_engine() {
Console.Write("I am here");
return -1;
}
public abstract void set_engine_id(int id);
}
// and then in the classes:
// code c#
class BIKE : VEHICLE {
private int engine_id;
public BIKE() {}
public
int get_engine() {
return engine_id;
}
public override void set_engine_id(int id) {
engine_id = id;
}
}
class CAR : VEHICLE {
private int engine_id;
public CAR() {}
public
int get_engine() {
return engine_id;
}
public override void set_engine_id(int id) {
engine_id = id;
}
}
That is it for abstract classes and interfaces. We hope that you understand the differences and can use it efficiently as you desire.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub