在 C# 中複製一個物件
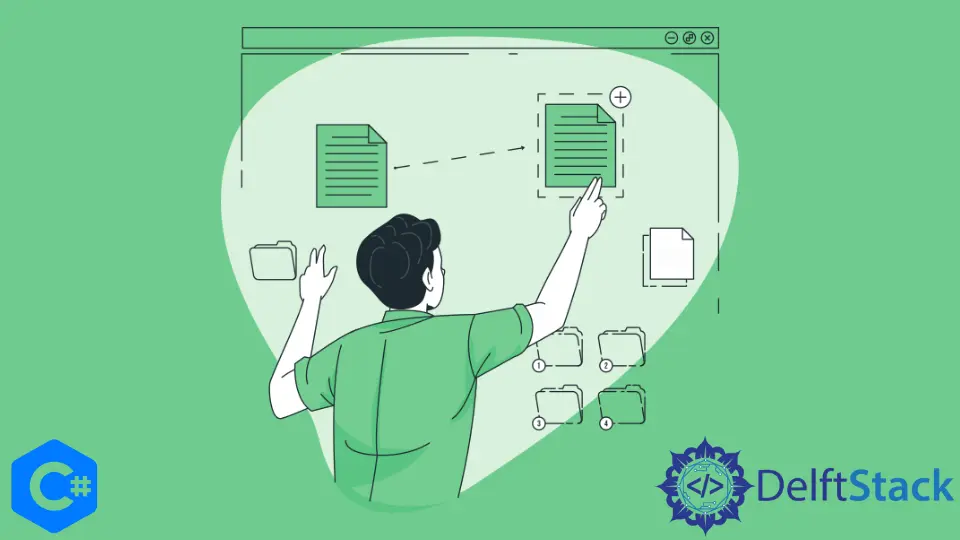
本教程將介紹在 C# 中複製物件的方法。
在 C# 中使用 MemberWiseClone()
方法複製物件
不幸的是,沒有內建的方法可以在 C# 中建立物件的單獨副本。在下面的程式碼示例中證明了這種現象。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace copy_an_object {
class myClass {
public String test;
}
class Program {
static void Main(string[] args) {
myClass a = new myClass();
a.test = "This is a test";
myClass b = a;
b.test = "This is not a test";
Console.WriteLine(a.test);
Console.WriteLine(b.test);
}
}
}
輸出:
This is not a test
This is not a test
我們兩次都得到相同的輸出,因為 C# 中的類的例項是指向特定記憶體位置的引用型別變數。由於 C# 的物件導向特性,新指標 b
也指向 a
的儲存位置。
如果要為 b
建立一個單獨的儲存位置,則必須依靠某些使用者定義的方法。MemberWiseClone()
方法用於在 C# 中建立呼叫物件的值的單獨副本。MemberWiseClone()
函式的返回型別是 object。以下程式碼示例向我們展示瞭如何使用 C# 中的 MemberWiseClone()
函式建立類物件的單獨副本。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace copy_an_object {
class myClass {
public String test;
public object Clone() {
return this.MemberwiseClone();
}
}
class Program {
static void Main(string[] args) {
myClass a = new myClass();
a.test = "This is a test";
myClass b = (myClass)a.Clone();
b.test = "This is not a test";
Console.WriteLine(a.test);
Console.WriteLine(b.test);
}
}
}
輸出:
This is a test
This is not a test
這次從輸出中可以清楚地看到每個類物件都有一個單獨的副本。我們在 myClass
類的 Clone()
函式內部實現了 MemberWiseClone()
函式。Clone()
函式返回類物件的單獨副本。Clone()
函式的返回值被型別轉換為 myClass
,並由 b
類物件指向。
使用 C# 中的引數化構造方法複製物件
我們可以建立一個引數化的類建構函式以在 C# 中實現相同的目標。我們可以將先前的類物件傳遞給新類物件的建構函式,並從中複製所有值。以下程式碼示例向我們展示瞭如何使用 C# 中的引數化建構函式方法建立類物件的單獨副本。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace copy_an_object {
class MyClass {
public String test;
public MyClass() {}
public MyClass(MyClass other) {
test = other.test;
}
}
class Program {
static void Main(string[] args) {
MyClass a = new myClass();
a.test = "This is a test";
MyClass b = new MyClass(a);
b.test = "This is not a test";
Console.WriteLine(a.test);
Console.WriteLine(b.test);
}
}
}
輸出:
This is a test
This is not a test
我們為 MyClass
類建立了一個引數化的建構函式,該建構函式接受 MyClass
類的物件並從中複製 test
字串。在主函式中,我們建立了 MyClass
類的物件 a
,並將其傳遞給了 b
的建構函式。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn