在 C# 中复制一个对象
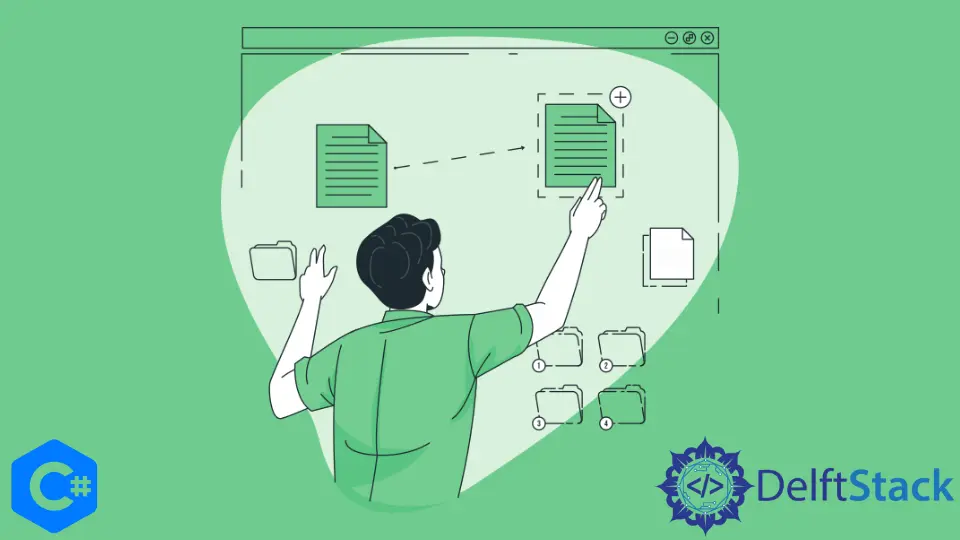
本教程将介绍在 C# 中复制对象的方法。
在 C# 中使用 MemberWiseClone()
方法复制对象
不幸的是,没有内置的方法可以在 C# 中创建对象的单独副本。在下面的代码示例中证明了这种现象。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace copy_an_object {
class myClass {
public String test;
}
class Program {
static void Main(string[] args) {
myClass a = new myClass();
a.test = "This is a test";
myClass b = a;
b.test = "This is not a test";
Console.WriteLine(a.test);
Console.WriteLine(b.test);
}
}
}
输出:
This is not a test
This is not a test
我们两次都得到相同的输出,因为 C# 中的类的实例是指向特定内存位置的引用类型变量。由于 C# 的面向对象特性,新指针 b
也指向 a
的存储位置。
如果要为 b
创建一个单独的存储位置,则必须依靠某些用户定义的方法。MemberWiseClone()
方法用于在 C# 中创建调用对象的值的单独副本。MemberWiseClone()
函数的返回类型是 object。以下代码示例向我们展示了如何使用 C# 中的 MemberWiseClone()
函数创建类对象的单独副本。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace copy_an_object {
class myClass {
public String test;
public object Clone() {
return this.MemberwiseClone();
}
}
class Program {
static void Main(string[] args) {
myClass a = new myClass();
a.test = "This is a test";
myClass b = (myClass)a.Clone();
b.test = "This is not a test";
Console.WriteLine(a.test);
Console.WriteLine(b.test);
}
}
}
输出:
This is a test
This is not a test
这次从输出中可以清楚地看到每个类对象都有一个单独的副本。我们在 myClass
类的 Clone()
函数内部实现了 MemberWiseClone()
函数。Clone()
函数返回类对象的单独副本。Clone()
函数的返回值被类型转换为 myClass
,并由 b
类对象指向。
使用 C# 中的参数化构造方法复制对象
我们可以创建一个参数化的类构造函数以在 C# 中实现相同的目标。我们可以将先前的类对象传递给新类对象的构造函数,并从中复制所有值。以下代码示例向我们展示了如何使用 C# 中的参数化构造函数方法创建类对象的单独副本。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace copy_an_object {
class MyClass {
public String test;
public MyClass() {}
public MyClass(MyClass other) {
test = other.test;
}
}
class Program {
static void Main(string[] args) {
MyClass a = new myClass();
a.test = "This is a test";
MyClass b = new MyClass(a);
b.test = "This is not a test";
Console.WriteLine(a.test);
Console.WriteLine(b.test);
}
}
}
输出:
This is a test
This is not a test
我们为 MyClass
类创建了一个参数化的构造函数,该构造函数接受 MyClass
类的对象并从中复制 test
字符串。在主函数中,我们创建了 MyClass
类的对象 a
,并将其传递给了 b
的构造函数。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn