在 C# 中从多个类继承
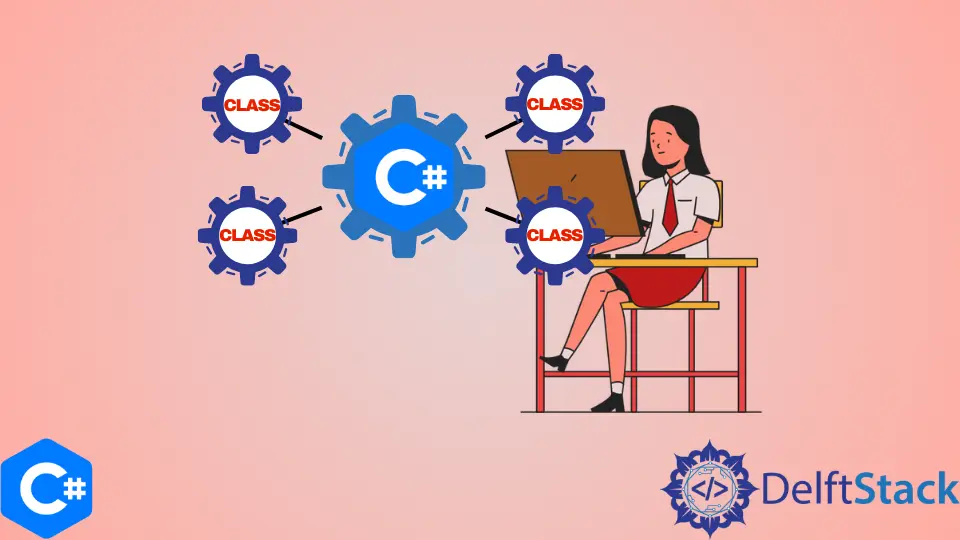
一个类或对象可以从 OOP 语言中的一个或多个父对象或类继承特征和特性。当子类需要访问任何或所有父类的属性时,就会使用继承。
当一个子类需要合并许多基类构造体时,它也很方便。在本教程中,我们将讨论如何在 C# 中实现多重继承。
在 C#
中实现多重继承
在 C# 语言中,最著名和最直接的技术是使用接口。这里我们有一个例子,我们将在其中实现从多个类的继承来计算 BMI。
我们将逐步学习这一点。首先,我们需要导入 System
库来访问 C# 中使用的方法。
using System;
我们将使用 setter 方法创建一个名为 Attributes
的类,我们将使用它从其他类中检索值。在其中声明两个双精度型变量,名为 weight
和 height
。
我们将保持它们 protected
,这意味着我们类以外的任何人都无法使用它们。接下来,创建一个带有双类型参数 w
的方法 setWeight()
,将提供的值分配给 weight
,并创建 setHeight()
方法,将 height
的值设置为相同就像这个一样。
class Attributes {
protected double weight;
protected double height;
public void setWeight(double w) {
weight = w;
}
public void setHeight(double h) {
height = h;
}
}
然后,创建一个接口 RetrieveAge
,其中包含一个双类型方法 retrieveAge
,该方法采用双数据类型的参数 age
。
public interface RetrieveAge {
double retrieveAge(double age);
}
创建一个将继承两个类 Attributes
和 RetrieveAge
的 BMI
类。我们将创建 4 个方法来从此类中的父类中检索值。
第一个方法是一个名为 retrieveBMI()
的双精度数据类型,它从父类 Attributes
获取体重和身高,然后将体重除以身高的平方,然后返回结果。接下来的两个方法 retrieveHeight()
和 retrieveWeight()
将获取身高和体重值并返回它们。
最后一个方法 retrieveAge()
扩展了 RetrieveAge
的方法,该方法接受一个双精度类型的参数 age
并返回它。
class BMI : Attributes, RetrieveAge {
public double retrieveBMI() {
return (weight / (height * height));
}
public double retrieveHeight() {
return height;
}
public double retrieveWeight() {
return weight;
}
public double retrieveAge(double age) {
return age;
}
}
我们创建最终类,该类将具有 Main()
函数。在 main 函数中,创建一个 BMI
类实例。
使用 setHeight()
和 setWeight()
方法来分配身高和体重。最后,我们需要输出身高、体重、计算出的 BMI 和年龄。
完整代码:
using System;
class Attributes {
protected double weight;
protected double height;
public void setWeight(double w) {
weight = w;
}
public void setHeight(double h) {
height = h;
}
}
public interface RetrieveAge {
double retrieveAge(double age);
}
class BMI : Attributes, RetrieveAge {
public double retrieveBMI() {
return (weight / (height * height));
}
public double retrieveHeight() {
return height;
}
public double retrieveWeight() {
return weight;
}
public double retrieveAge(double age) {
return age;
}
}
class TotalBMI {
static void Main() {
BMI bmi = new BMI();
bmi.setWeight(80);
bmi.setHeight(2.07);
Console.WriteLine("Your Height: {0} m", bmi.retrieveHeight());
Console.WriteLine("Your Weight: {0} kg", bmi.retrieveWeight());
Console.WriteLine("BMI: {0}", bmi.retrieveBMI());
Console.WriteLine("Age: {0}", bmi.retrieveAge(40));
}
}
输出:
Your Height: 2.07 m
Your Weight: 80 kg
BMI: 18.6702140073281
Age: 40
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn