C# 中的深拷贝
Muhammad Maisam Abbas
2024年2月16日
Csharp
Csharp Class
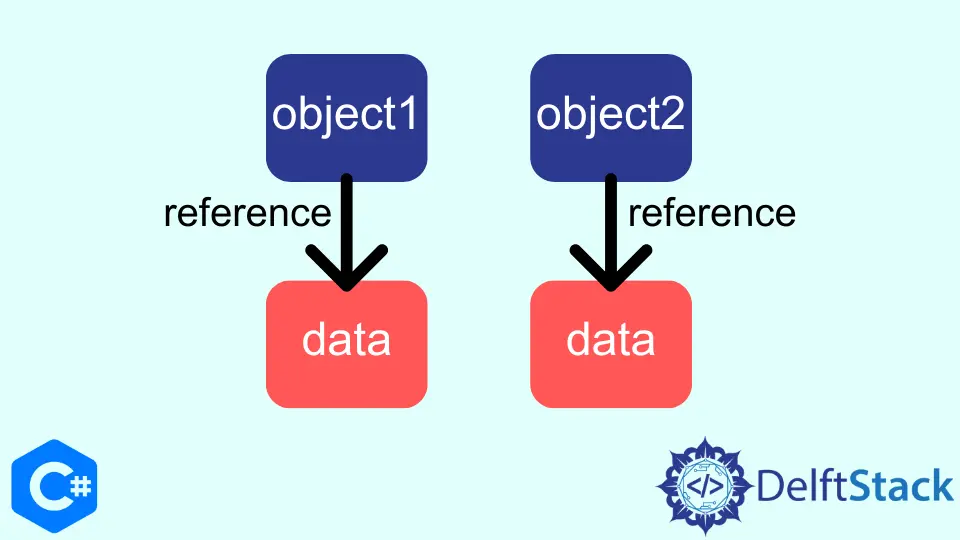
本教程将介绍在 C# 中创建类对象的深拷贝的方法。
在 C# 中使用 BinaryFormatter
类进行深拷贝对象
深拷贝意味着将一个对象的每个字段复制到另一个对象,而浅层复制意味着创建一个新的类实例并将其指向先前的类实例的值。我们可以使用 BinaryFormatter
在 C# 中创建类对象的深拷贝。BinaryFormatter
类以二进制格式读取和写入类对象到流中。我们可以使用 BinaryFormatter.Serialize()
方法将类对象写入 C# 中的内存流。然后,我们可以使用 BinaryFormatter.Deserialize()
方法将相同的内存流写入对象并返回它。我们需要首先将我们的类标记为 [Serializable]
,以便这种方法能够发挥作用。下面的代码例子向我们展示了如何用 C# 中的 BinaryFormatter
类创建一个对象的深度拷贝。
using System;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
namespace deep_copy {
[Serializable]
public class Sample {
public string sampleName { get; set; }
}
static class ext {
public static Sample deepCopy<Sample>(this Sample obj) {
using (var memStream = new MemoryStream()) {
var bFormatter = new BinaryFormatter();
bFormatter.Serialize(memStream, obj);
memStream.Position = 0;
return (Sample)bFormatter.Deserialize(memStream);
}
}
}
class Program {
static void Main(string[] args) {
Sample s1 = new Sample();
s1.sampleName = "Sample number 1";
Sample s2 = s1.deepCopy();
Console.WriteLine("Sample 1 = {0}", s1.sampleName);
Console.WriteLine("Sample 2 = {0}", s2.sampleName);
}
}
}
输出:
Sample 1 = Sample number 1
Sample 2 = Sample number 1
在上面的代码中,我们创建了 Sample
类的对象 s1
的深拷贝,并使用 C# 中的 BinarySerializer
类将其保存在同一类的对象 s2
中。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn