C#의 여러 클래스에서 상속
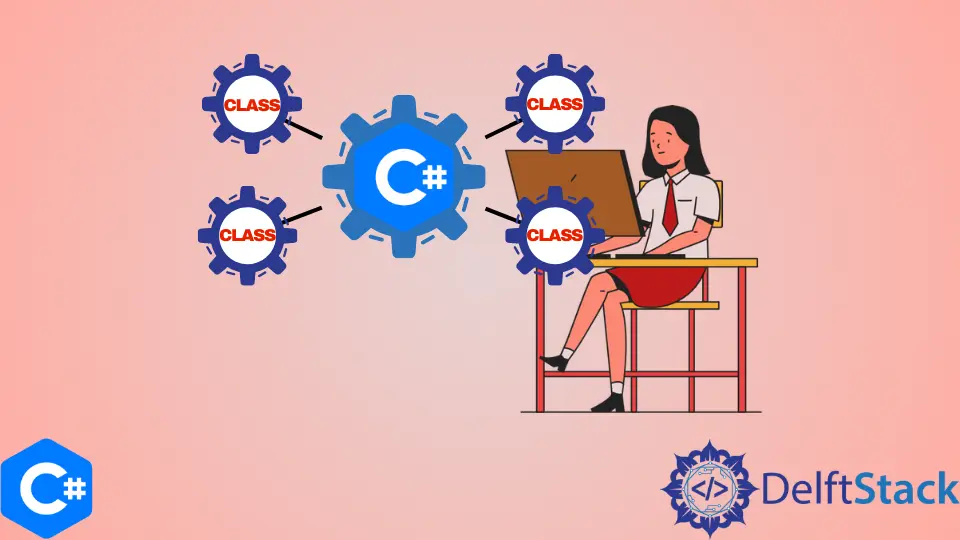
클래스 또는 개체는 OOP 언어의 하나 이상의 부모 개체 또는 클래스에서 기능과 특성을 상속할 수 있습니다. 하위 클래스가 상위 클래스의 속성 중 일부 또는 전체에 액세스해야 하는 경우 상속이 활용됩니다.
자식 클래스가 많은 기본 클래스 생성자를 병합해야 하는 경우에도 유용합니다. 이 자습서에서는 C#에서 다중 상속을 구현하는 방법을 다룰 것입니다.
C#
에서 다중 상속 구현
C# 언어에서 가장 잘 알려져 있고 간단한 기술은 인터페이스를 활용하는 것입니다. 여기에 BMI를 계산하기 위해 여러 클래스에서 상속을 구현하는 예가 있습니다.
우리는 이것을 단계별로 배울 것입니다. 먼저 C#에서 사용되는 메서드에 액세스하려면 System
라이브러리를 가져와야 합니다.
using System;
다른 클래스에서 값을 검색하는 데 사용할 setter 메서드를 사용하여 Attributes
라는 클래스를 생성합니다. 그 안에 weight
와 height
라는 두 개의 이중 유형 변수를 선언합니다.
우리는 그것들을 보호된
상태로 유지할 것입니다. 즉, 우리 수업 이외의 사람은 사용할 수 없습니다. 다음으로, 제공된 값을 weight
에 할당하는 이중 유형 인수 w
를 사용하여 setWeight()
메서드를 만들고 동일한 값에서 height
값을 설정하는 setHeight()
메서드를 만듭니다. 이대로.
class Attributes {
protected double weight;
protected double height;
public void setWeight(double w) {
weight = w;
}
public void setHeight(double h) {
height = h;
}
}
그런 다음 이중 데이터 유형의 age
인수를 사용하는 retrieveAge
이중 유형 메소드를 포함하는 RetrieveAge
인터페이스를 만듭니다.
public interface RetrieveAge {
double retrieveAge(double age);
}
속성
및 RetrieveAge
라는 두 개의 클래스를 상속할 BMI
클래스를 작성하십시오. 이 클래스 내의 부모 클래스에서 값을 검색하는 4개의 메서드를 만들 것입니다.
첫 번째 방법은 retrieveBMI()
라는 이중 데이터 유형으로, 부모 클래스 Attributes
에서 무게와 키를 가져와 무게를 높이의 제곱으로 나눈 결과를 반환합니다. 다음 두 메서드 retrieveHeight()
및 retrieveWeight()
는 키와 몸무게 값을 가져와 반환합니다.
마지막 메소드인 retrieveAge()
는 이중 유형의 age
인수를 가져와 반환하는 RetrieveAge
에서 메소드를 확장합니다.
class BMI : Attributes, RetrieveAge {
public double retrieveBMI() {
return (weight / (height * height));
}
public double retrieveHeight() {
return height;
}
public double retrieveWeight() {
return weight;
}
public double retrieveAge(double age) {
return age;
}
}
Main()
함수가 있는 최종 클래스를 만듭니다. 메인 함수 내에서 BMI
클래스 인스턴스를 만듭니다.
setHeight()
및 setWeight()
메서드를 사용하여 키와 체중을 할당합니다. 마지막으로 키, 몸무게, 계산된 BMI, 나이를 출력해야 합니다.
전체 코드:
using System;
class Attributes {
protected double weight;
protected double height;
public void setWeight(double w) {
weight = w;
}
public void setHeight(double h) {
height = h;
}
}
public interface RetrieveAge {
double retrieveAge(double age);
}
class BMI : Attributes, RetrieveAge {
public double retrieveBMI() {
return (weight / (height * height));
}
public double retrieveHeight() {
return height;
}
public double retrieveWeight() {
return weight;
}
public double retrieveAge(double age) {
return age;
}
}
class TotalBMI {
static void Main() {
BMI bmi = new BMI();
bmi.setWeight(80);
bmi.setHeight(2.07);
Console.WriteLine("Your Height: {0} m", bmi.retrieveHeight());
Console.WriteLine("Your Weight: {0} kg", bmi.retrieveWeight());
Console.WriteLine("BMI: {0}", bmi.retrieveBMI());
Console.WriteLine("Age: {0}", bmi.retrieveAge(40));
}
}
출력:
Your Height: 2.07 m
Your Weight: 80 kg
BMI: 18.6702140073281
Age: 40
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn