How to Convert Int to Bool in C#
-
Convert Integer to Boolean With the
Convert.ToBoolean()
Method in C# -
Convert Integer to Boolean With the
switch()
Statement in C# - Convert Integer to Boolean Using Ternary Operator in C#
- Conclusion
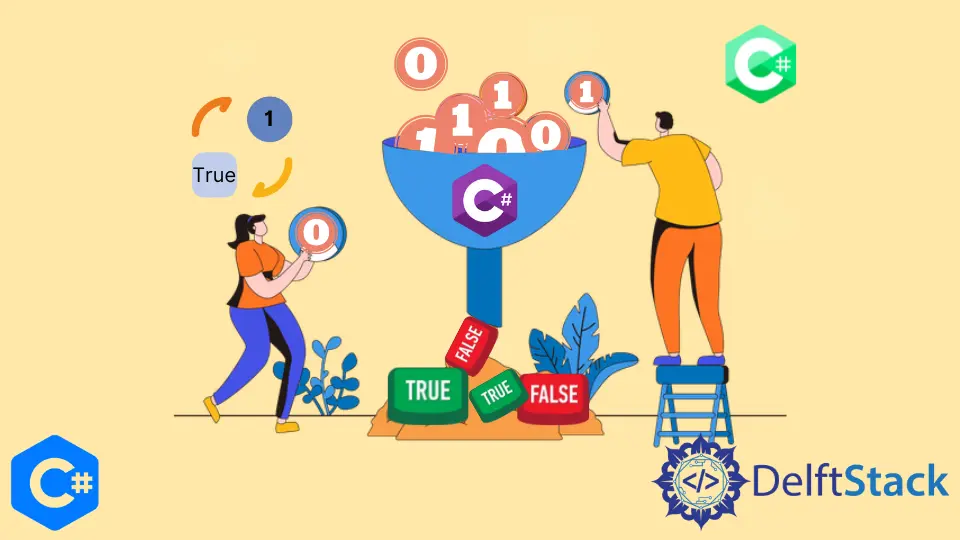
This article focuses on converting integer values to boolean representations in C# using different methods. The primary method discussed is the Convert.ToBoolean()
method, a built-in function in C# that effectively handles the conversion, returning true
for non-zero integers and false
for zero.
Additionally, the switch()
statement and the ternary operator (?:
) are presented as alternative approaches for achieving the same conversion.
Convert Integer to Boolean With the Convert.ToBoolean()
Method in C#
The Convert.ToBoolean()
method is a built-in function in C# that converts the specified value to its equivalent Boolean representation. It handles a wide range of data types and returns true
if the value is true or non-zero and false
if the value is false or zero.
-
To convert an integer to Boolean using the
Convert.ToBoolean()
method, begin by assigning the integer value to a variable of typeint
.int intValue = 1;
-
Then, use the
Convert.ToBoolean()
method to perform the conversion.bool boolValue = Convert.ToBoolean(intValue);
-
The method
Convert.ToBoolean(intValue)
will returntrue
for any non-zero integer andfalse
for zero.
Let’s demonstrate the process with a complete code example:
using System;
class Program {
static void Main(string[] args) {
int intValue = 1;
bool boolValue = Convert.ToBoolean(intValue);
Console.WriteLine("Integer Value: " + intValue);
Console.WriteLine("Boolean Value: " + boolValue);
int zeroValue = 0;
bool zeroBoolValue = Convert.ToBoolean(zeroValue);
Console.WriteLine("Integer Value (zero): " + zeroValue);
Console.WriteLine("Boolean Value (zero): " + zeroBoolValue);
}
}
Output:
Integer Value: 1
Boolean Value: True
Integer Value (zero): 0
Boolean Value (zero): False
In this example:
intValue
is assigned the value1
, which is an integer.boolValue
is assigned the Boolean value obtained by convertingintValue
usingConvert.ToBoolean()
.zeroValue
is assigned the value0
, which is an integer representing zero.zeroBoolValue
is assigned the Boolean value obtained by convertingzeroValue
usingConvert.ToBoolean()
.
We use Console.WriteLine()
to output the values of intValue
, boolValue
, zeroValue
, and zeroBoolValue
to the console.
In the output, we can see that it shows the integer values and their corresponding Boolean representations.
Convert Integer to Boolean With the switch()
Statement in C#
The switch()
statement in C# is designed for efficient decision-making based on the value of a specified expression. It evaluates the expression against various cases and executes the corresponding block of code for the matching case.
This simplifies complex branching logic and enhances the readability of the code.
To convert an integer value to a Boolean using the switch()
statement, follow these steps:
-
Begin by assigning the integer value to a variable of type
int
. -
Incorporate the
switch()
statement and specify the integer variable as the expression to be evaluated. -
Define cases within the
switch()
statement corresponding to different integer values. Assign the desired Boolean value based on the case. -
Optionally, display the Boolean value obtained from the conversion.
Let’s explore a code example illustrating the process of converting an integer to a Boolean using the switch()
statement:
using System;
namespace ConvertIntToBool {
class Program {
static void Main(string[] args) {
int intValue = 1;
bool boolValue;
switch (intValue) {
case 0:
boolValue = false;
break;
case 1:
boolValue = true;
break;
default:
Console.WriteLine("Unhandled integer value.");
return;
}
Console.WriteLine("Integer Value: " + intValue);
Console.WriteLine("Boolean Value: " + boolValue);
}
}
}
Output:
Integer Value: 1
Boolean Value: True
In this example, we first assign an integer value to intValue
(Step 1). Within the switch()
statement (Step 2), we define cases for 0
and 1
, assigning the corresponding Boolean values (Step 3).
If the integer value doesn’t match any case, the default
case handles it gracefully.
Convert Integer to Boolean Using Ternary Operator in C#
The ternary operator (?:
) in C# is a conditional operator in C# that evaluates a Boolean expression and returns one of two possible values based on whether the expression is true or false. It has the following syntax:
condition? valueIfTrue : valueIfFalse;
If the condition
is true, the operator returns valueIfTrue
; otherwise, it returns valueIfFalse
.
To convert an integer value to a Boolean value using the ternary operator, follow these steps:
-
Begin by assigning the integer value to a variable of type
int
. -
Utilize the ternary operator (
?:
) to create a condition based on the integer variable and return a Boolean value. -
Assign the resulting Boolean value from the ternary operator to a Boolean variable.
Let’s illustrate the process with a code example:
using System;
class Program {
static void Main(string[] args) {
int intValue = 1;
bool boolValue = (intValue != 0) ? true : false;
Console.WriteLine("Integer Value: " + intValue);
Console.WriteLine("Boolean Value: " + boolValue);
}
}
Output:
Integer Value: 1
Boolean Value: True
This code starts by declaring an integer variable intValue
and assigns it the value 1.
It then uses the ternary operator to check if intValue
is not equal to zero. If true, it assigns true
to boolValue
; otherwise, it assigns false
.
Finally, it displays the original integer value and the Boolean value after conversion to the console.
Conclusion
Converting integers to Boolean values is a fundamental operation in programming, often required for efficient decision-making and logic implementation. The Convert.ToBoolean()
method in C# serves as a straightforward approach to achieve this conversion, producing the desired Boolean output based on the input integer.
Additionally, utilizing the switch()
statement and the ternary operator provides programmers with alternative techniques to accomplish the same objective, allowing for flexibility in code implementation and enhancing code readability. Understanding these methods can help to handle integer-to-boolean conversions effectively, catering to various programming needs.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn