在 C# 中将整数转换为布尔值
Muhammad Maisam Abbas
2024年2月16日
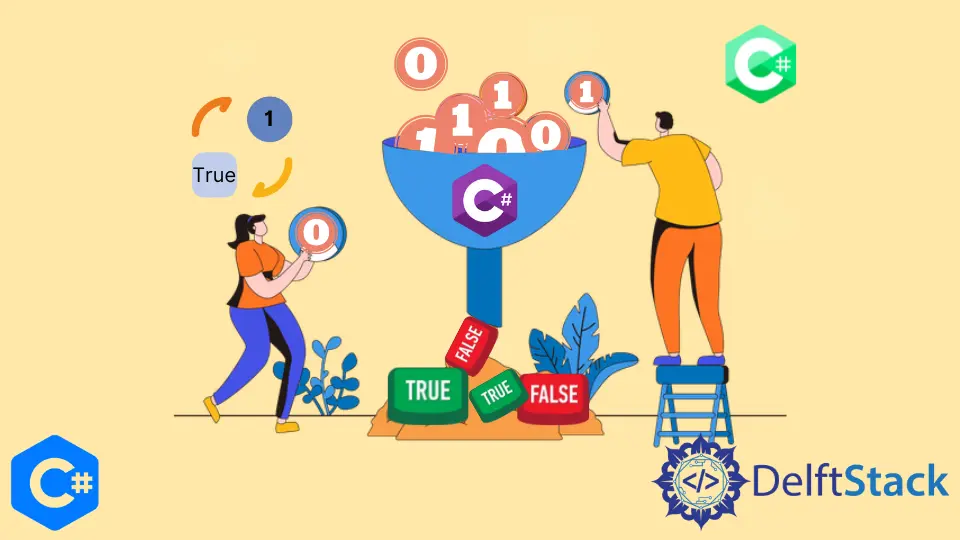
本教程将讨论在 C# 中将整数值转换为布尔值的方法。
在 C# 中使用 Convert.ToBoolean()
方法将整数转换为布尔值
由于整数和布尔值都是基本数据类型,因此我们可以使用 Convert
类将整数值转换为布尔值。Convert.ToBoolean()
方法在 C# 中将整数值转换为布尔值。在 C# 中,整数值 0
等于布尔值中的 false
,而整数值 1
等于布尔值中的 true
。
using System;
namespace convert_int_to_bool {
class Program {
static void Main(string[] args) {
int i = 1;
bool b = Convert.ToBoolean(i);
Console.WriteLine(b);
}
}
}
输出:
True
在上面的代码中,我们使用 C# 中的 Convert.ToBoolean(i)
函数将值为 1
的整数变量 i
转换为值为 true
的布尔变量 b
。
使用 C# 中的 switch()
语句将整数转换为布尔值
我们还可以使用 switch()
语句实现与上一个示例相同的目标。switch()
语句测试变量在 C# 中不同值列表之间的相等性。我们可以在 switch()
语句中使用整数变量,在 0
整数值的情况下将 false
分配给布尔变量,或者在 1
整数值的情况下将 true
分配给布尔值。下面的代码示例向我们展示了如何使用 C# 中的 switch()
语句将整数变量转换为布尔变量。
using System;
namespace convert_int_to_bool {
class Program {
static void Main(string[] args) {
int i = 1;
bool b;
switch (i) {
case 0:
b = false;
Console.WriteLine(b);
break;
case 1:
b = true;
Console.WriteLine(b);
break;
}
}
}
}
输出:
True
在上面的代码中,我们使用 C# 中的 switch(i)
语句将值为 1
的整数变量 i
转换为值为 true
的布尔变量 b
。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn