How to Convert Integer to Binary in C#
- Manually Convert Integer to Binary Using a Loop in C#
-
Convert Integer to Binary With the
Convert.ToString()
Function in C# - Conclusion
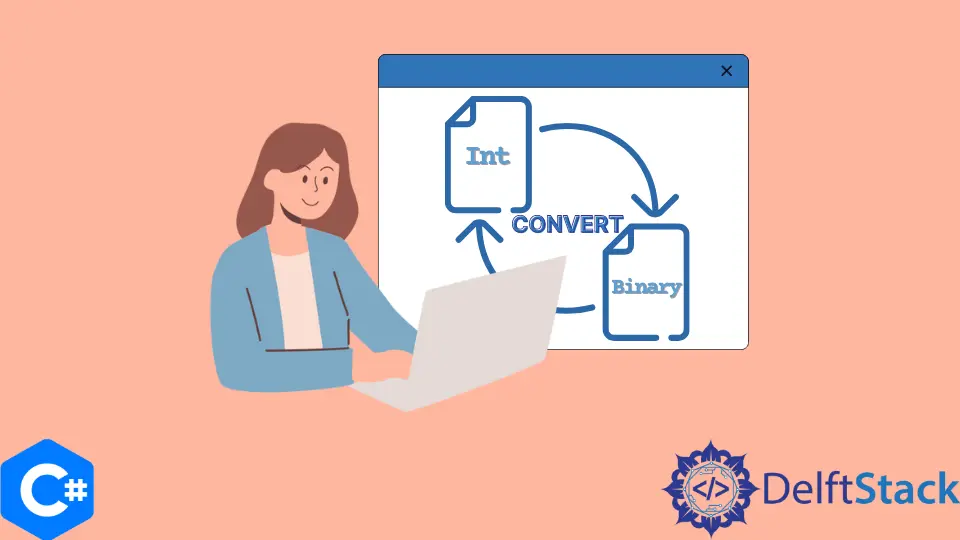
Binary representation is a fundamental concept in computer science, often employed to manipulate and understand the underlying bit patterns of data. In C#, converting a decimal integer to a binary string can be achieved through various methods.
In this article, we’ll explore the methods to convert a decimal integer to a binary string in C#.
Manually Convert Integer to Binary Using a Loop in C#
Before diving into the conversion process, it’s essential to understand the basic concepts of decimal and binary representations.
Decimal is a base-10 numeral system, using digits from 0
to 9
. On the other hand, binary is a base-2 system, utilizing only 0
s and 1
s.
Converting a decimal number to binary involves expressing the number as a sum of powers of 2.
The manual conversion method involves iteratively dividing the decimal number by 2 and recording the remainder until the quotient becomes zero. The remainder, read in reverse order, form the binary representation.
Let’s break down the steps:
-
First, you have to initialize a variable
decimalNumber
with the decimal integer you want to convert to binary. Then, initialize an empty stringbinaryString
to store the binary representation.int decimalNumber = 42; // Replace with your desired decimal number string binaryString = "";
-
Next, define a loop that continues until
decimalNumber
becomes zero.while (decimalNumber > 0) { binaryString = (decimalNumber % 2) + binaryString; decimalNumber /= 2; }
- Inside the loop:
- `(decimalNumber % 2)`: Get the remainder of the division of `decimalNumber` by 2.
- `(decimalNumber % 2) + binaryString;`: Concatenate the remainder to the beginning of `binaryString`.
- `decimalNumber /= 2;`: Update `decimalNumber` by performing integer division by 2, discarding the remainder.
-
Lastly, print the final binary representation of the original decimal number.
Console.WriteLine($"Binary representation: {binaryString}");
The complete C# code looks like this:
using System;
class Program
{
static void Main()
{
int decimalNumber = 15;
string binaryString = "";
while (decimalNumber > 0)
{
binaryString = (decimalNumber % 2) + binaryString;
decimalNumber /= 2;
}
Console.WriteLine($"Binary representation: {binaryString}");
}
}
Output:
Binary representation: 1111
The code iteratively divides the decimal number by 2, recording the remainder in reverse order. The binary representation is constructed by appending these remainders to the beginning of the binaryString
.
The process continues until the decimal number becomes zero, and the resulting binary string is then displayed.
Convert Integer to Binary With the Convert.ToString()
Function in C#
The Convert.ToString
method in C# provides another convenient way to convert numerical values to strings. When using this method for decimal to binary conversion, we can specify the base as an argument.
The Convert.ToString
method in C# has several overloads, but in our case, for converting a decimal integer to a binary string with a specified base, the relevant syntax is:
string binaryString = Convert.ToString(decimalNumber, 2);
Here:
decimalNumber
is the decimal integer you want to convert.2
specifies the base for the conversion, in this case, indicating binary representation.
The method returns a string that represents the binary equivalent of the decimal number.
Let’s see the steps to convert a decimal integer to a binary string using Convert.ToString
:
-
Begin by initializing an integer variable. Here, we have a variable named
decimalNumber
with the value42
.int decimalNumber = 42; // Replace with your desired decimal number
You can replace `42` with any decimal number you want to convert to binary.
-
Next, use the
Convert.ToString
method to convert the decimal number (decimalNumber
) to a binary string. The second parameter,2
, specifies the base for the conversion, indicating that the result should be in binary.string binaryString = Convert.ToString(decimalNumber, 2);
The result is a binary representation stored in the `binaryString` variable.
-
Finally, we print the binary representation using
Console.WriteLine
.Console.WriteLine($"Binary representation: {binaryString}");
When all of the above steps are put together, the complete C# code looks like this:
using System;
class Program
{
static void Main()
{
int decimalNumber = 30;
string binaryString = Convert.ToString(decimalNumber, 2);
Console.WriteLine($"Binary representation: {binaryString}");
}
}
Output:
Binary representation: 11110
One advantage of using this method is that the code is concise and easy to understand, making it accessible for programmers of all levels. Using built-in methods like Convert.ToString
is generally optimized and efficient.
Conclusion
The manual conversion method with a loop and the use of the Convert.ToString
function offer distinct approaches to converting a decimal integer to a binary string in C#. The manual method provides an educational and hands-on experience, allowing developers to understand the fundamental principles involved in binary conversion.
On the other hand, the Convert.ToString
function provides a more concise and efficient solution suitable for scenarios where simplicity and built-in functionality are prioritized. The choice between these methods depends on your preference, coding style, and the specific requirements of the task at hand.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp Integer
- How to Convert Int to String in C#
- How to Convert Int to Enum in C#
- Random Int in C#
- Random Number in a Range in C#
- How to Convert String to Int in C#
- Integer Division in C#