C#에서 정수를 이진으로 변환
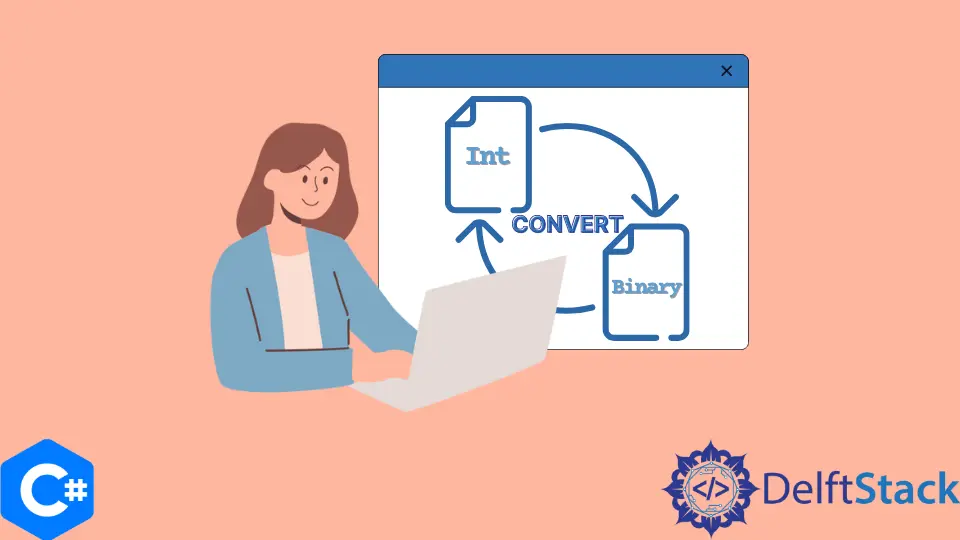
이 자습서에서는 C#에서 10 진수 정수를 이진 문자열로 변환하는 방법에 대해 설명합니다.
C#의 기존 메서드를 사용하여 정수를 이진으로 변환
기존의 방법은 10 진수를 이진수로 변환하려면 숫자를2
로 계속 나누고 숫자가2
보다 작아 질 때까지 각 단계의 나머지를 저장해야한다고 지정합니다. 이 논리를 사용하여 10 진수 정수를 이진 문자열로 변환하고 결과를 문자열 변수에 연결할 수 있습니다. 아래 예를 참조하십시오.
using System;
namespace convert_int_to_binary {
class Program {
static void method1() {
int decimalNumber = 15;
int remainder;
string binary = string.Empty;
while (decimalNumber > 0) {
remainder = decimalNumber % 2;
decimalNumber /= 2;
binary = remainder.ToString() + binary;
}
Console.WriteLine("Binary: {0}", binary);
}
static void Main(string[] args) {
method1();
}
}
}
출력:
1111
10 진수 값15
를 C#의 기존 논리를 사용하여1111
을 포함하는 이진 문자열로 변환했습니다.
C#에서Convert.ToString()
함수를 사용하여 정수를 이진으로 변환
십진 정수 변수를 이진 문자열 변수로 변환하는 또 다른 간단한 방법은 C#에서 Convert.ToString()
function을 사용하는 것입니다. Convert.ToString(dec, 2)
는dec
를 기본2
로 변환하고 결과를 문자열 형식으로 반환합니다. 다음 코드 예제는 C#에서Convert.ToString()
함수를 사용하여 10 진수 정수를 이진 문자열로 변환하는 방법을 보여줍니다.
using System;
namespace convert_int_to_binary {
class Program {
static void method2() {
int value = 15;
string binary = Convert.ToString(value, 2);
Console.WriteLine("Binary: {0}", binary);
}
static void Main(string[] args) {
method2();
}
}
}
출력:
1111
이 코드는 이전 접근 방식보다 훨씬 간단하고 이해하기 쉽습니다. C#의Convert.ToString()
함수를 사용하여 10 진수 값15
를1111
을 포함하는 이진 문자열로 변환했습니다.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn