How to Compare Two Strings by Ignoring Case in C#
-
Case-Insensitive String Comparison Using the
String.ToUpper()
Method in C# -
Case-Insensitive String Comparison Using the
String.ToLower()
Function in C# -
Case-Insensitive String Comparison Using the
String.Equals()
Function in C# -
Case-Insensitive String Comparison Using
String.Compare
WithStringComparison
in C# -
Case-Insensitive String Comparison Using
String.Compare
WithCultureInfo
in C# - Conclusion
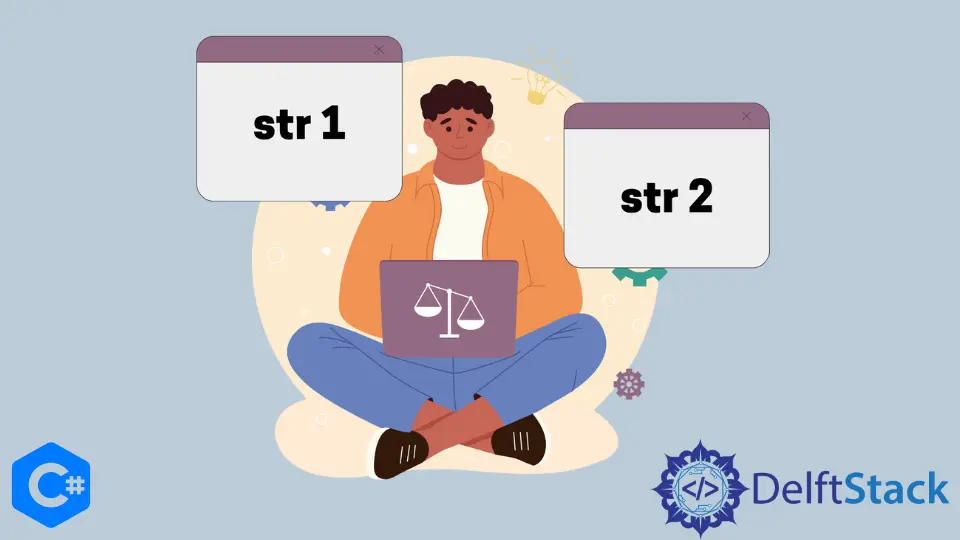
String comparisons lie at the heart of many programming tasks, and in the realm of C#, there are occasions when we need to compare strings without considering the case of the characters. This becomes crucial in scenarios where user input validation or data processing demands a case-insensitive approach.
Fortunately, C# equips developers with multiple methods to seamlessly handle such comparisons, providing flexibility and adaptability to diverse coding scenarios. In this article, we’ll explore various techniques to perform case-insensitive string comparisons in C#.
Case-Insensitive String Comparison Using the String.ToUpper()
Method in C#
One straightforward approach to perform case-insensitive comparisons is by using the String.ToUpper
method. This method converts all the characters in a string to uppercase, allowing for a consistent and case-insensitive basis for comparison.
The syntax of String.ToUpper
is simple:
string resultString = originalString.ToUpper();
Here, originalString
is the string you want to convert to uppercase, and the resulting resultString
will contain the uppercase version of the original string.
Let’s consider a scenario where we have two strings, str1
and str2
, and we want to check if they are equal in a case-insensitive manner using String.ToUpper
:
using System;
class Program {
static void Main() {
string str1 = "Hello";
string str2 = "hello";
bool isEqual = str1.ToUpper() == str2.ToUpper();
Console.WriteLine($"Are str1 and str2 equal (case-insensitive)? {isEqual}");
}
}
In the example above, we have two strings, str1
and str2
. To perform a case-insensitive comparison, we use the ToUpper
method on both strings.
This method returns a new string with all characters converted to uppercase.
bool isEqual = str1.ToUpper() == str2.ToUpper();
The equality check is then done between the uppercase versions of the two strings. If they are equal, isEqual
will be true
, indicating that the original strings are equal in a case-insensitive manner.
Output:
Are str1 and str2 equal (case-insensitive)? True
This approach is concise and easy to understand. It works by normalizing the case of both strings before comparison, ensuring that case differences do not affect the result.
Keep in mind that this method creates new strings, so it may not be the most efficient choice for large strings or in performance-critical scenarios. In such cases, other methods, like using String.Compare
with StringComparison.OrdinalIgnoreCase
, might be more suitable.
Case-Insensitive String Comparison Using the String.ToLower()
Function in C#
Another effective method for achieving case-insensitive string comparisons in C# is by using the String.ToLower
method. This method, similar to String.ToUpper
, transforms all characters in a string to lowercase, providing a consistent basis for comparison.
The syntax of String.ToLower
is straightforward:
string resultString = originalString.ToLower();
Here, originalString
is the string you want to convert to lowercase, and the resulting resultString
will contain the lowercase version of the original string.
Let’s explore a scenario where we compare two strings, text1
and text2
, for equality in a case-insensitive manner using String.ToLower
:
using System;
class Program {
static void Main() {
string text1 = "World";
string text2 = "WORLD";
bool isEqual = text1.ToLower() == text2.ToLower();
Console.WriteLine($"Are text1 and text2 equal (case-insensitive)? {isEqual}");
}
}
In the example above, we have two strings, text1
and text2
.
To perform a case-insensitive comparison, we utilize the ToLower
method on both strings. This method returns a new string with all characters converted to lowercase.
bool isEqual = text1.ToLower() == text2.ToLower();
The comparison is then done between the lowercase versions of the two strings. If they are equal, isEqual
will be true
, indicating that the original strings are equal in a case-insensitive manner.
Output:
Are text1 and text2 equal (case-insensitive)? True
This approach is clear and concise, providing a simple way to handle case-insensitive comparisons. Similar to String.ToUpper
, keep in mind that using String.ToLower
creates new strings, so it may not be the most efficient choice for large strings or performance-critical scenarios.
For such cases, alternatives like String.Compare
with StringComparison.OrdinalIgnoreCase
may be more appropriate.
Case-Insensitive String Comparison Using the String.Equals()
Function in C#
Both methods discussed above work fine, but there is a big disadvantage of using them. Some letters are changed to completely different letters in the English language if we use the String.ToUpper()
or the String.ToLower()
functions in C#.
In order to avoid this problem, C# offers the String.Equals
method, which provides a flexible way to perform case-insensitive string comparisons. The key to achieving this is by utilizing the StringComparison
enumeration, specifically StringComparison.OrdinalIgnoreCase
.
The basic syntax of String.Equals
with case-insensitive comparison is as follows:
bool isEqual = string.Equals(str1, str2, StringComparison.OrdinalIgnoreCase);
Here, str1
and str2
are the strings to be compared, and the third parameter, StringComparison.OrdinalIgnoreCase
, ensures that the comparison is case-insensitive.
Consider a scenario where we compare two strings, input1
and input2
, for equality in a case-insensitive manner using String.Equals
:
using System;
class Program
{
static void Main()
{
string input1 = "apple";
string input2 = "Apple";
bool isEqual = string.Equals(input1, input2, StringComparison.OrdinalIgnoreCase);
Console.WriteLine($"Are input1 and input2 equal (case-insensitive)? {isEqual}");
}
}
In the provided example, we have two strings, input1
and input2
. To perform a case-insensitive comparison, the String.Equals
method is employed with the StringComparison.OrdinalIgnoreCase
parameter.
bool isEqual = string.Equals(input1, input2, StringComparison.OrdinalIgnoreCase);
This comparison method directly checks for equality between the two strings while ignoring case differences. If the strings are equal in a case-insensitive manner, the isEqual
variable will be true
.
Output:
Are input1 and input2 equal (case-insensitive)? True
String.Equals
offers a readable and expressive way to conduct case-insensitive comparisons. The use of StringComparison.OrdinalIgnoreCase
ensures that the comparison is performed without considering the character case, providing a reliable mechanism for case-insensitive string equality checks.
Depending on your specific use case, choose the method that aligns best with your code style and requirements.
Case-Insensitive String Comparison Using String.Compare
With StringComparison
in C#
In C#, the String.Compare
method provides another versatile way to perform case-insensitive string comparisons. By incorporating the StringComparison.OrdinalIgnoreCase
parameter, we can ensure that the comparison ignores differences in character cases.
The syntax of String.Compare
with case-insensitive comparison is as follows:
int result = string.Compare(str1, str2, StringComparison.OrdinalIgnoreCase);
bool isEqual = (result == 0);
Here, str1
and str2
are the strings to be compared, and the third parameter, StringComparison.OrdinalIgnoreCase
, indicates a case-insensitive comparison. The result is an integer that represents the relationship between the two strings, and isEqual
is true
if the strings are equal.
Let’s explore a scenario where we use String.Compare
to compare two strings, word1
and word2
, for equality in a case-insensitive manner:
using System;
class Program {
static void Main() {
string word1 = "DotNET";
string word2 = "dotnet";
int result = string.Compare(word1, word2, StringComparison.OrdinalIgnoreCase);
bool isEqual = (result == 0);
Console.WriteLine($"Are word1 and word2 equal (case-insensitive)? {isEqual}");
}
}
In the provided example, we have two strings, word1
and word2
. To perform a case-insensitive comparison, the String.Compare
method is used with the StringComparison.OrdinalIgnoreCase
parameter.
int result = string.Compare(word1, word2, StringComparison.OrdinalIgnoreCase);
bool isEqual = (result == 0);
The result
variable holds the outcome of the comparison. If result
is equal to 0
, it signifies that the strings are equal in a case-insensitive manner, and therefore, isEqual
is set to true
.
Output:
Are word1 and word2 equal (case-insensitive)? True
Using String.Compare
provides a more detailed result than a simple boolean outcome. The method returns a numeric value that indicates whether one string is less than, equal to, or greater than the other.
This can be useful if you need to sort strings or determine their relative order. However, for simple equality checks, comparing the result to 0
suffices, as demonstrated in the example.
Case-Insensitive String Comparison Using String.Compare
With CultureInfo
in C#
In certain scenarios, considering cultural and linguistic differences in string comparisons is crucial. The String.Compare
method allows for such nuanced comparisons by incorporating the CultureInfo
parameter.
This enables developers to perform case-insensitive string comparisons that respect cultural conventions.
The syntax of String.Compare
with CultureInfo
for case-insensitive comparison is as follows:
CultureInfo culture = CultureInfo.InvariantCulture;
int result = string.Compare(str1, str2, culture, CompareOptions.IgnoreCase);
bool isEqual = (result == 0);
Here, str1
and str2
are the strings to be compared, and CultureInfo
provides cultural information for the comparison. The CompareOptions.IgnoreCase
parameter ensures that the comparison is case-insensitive.
Let’s explore a scenario where we use String.Compare
with CultureInfo
to compare two strings, text1
and text2
, for equality in a case-insensitive manner:
using System;
using System.Globalization;
class Program {
static void Main() {
string text1 = "cliché";
string text2 = "CLICHÉ";
CultureInfo culture = CultureInfo.InvariantCulture;
int result = string.Compare(text1, text2, culture, CompareOptions.IgnoreCase);
bool isEqual = (result == 0);
Console.WriteLine($"Are text1 and text2 equal (case-insensitive and culture-aware)? {isEqual}");
}
}
In the provided example, we have two strings, text1
and text2
. In order to perform a case-insensitive comparison with cultural awareness, the String.Compare
method is employed with the CultureInfo
and CompareOptions.IgnoreCase
parameters.
CultureInfo culture = CultureInfo.InvariantCulture;
int result = string.Compare(text1, text2, culture, CompareOptions.IgnoreCase);
bool isEqual = (result == 0);
Here, if result
is equal to 0
, it indicates that the strings are equal in a case-insensitive and culture-aware manner, and therefore, isEqual
is set to true
.
Output:
Are text1 and text2 equal (case-insensitive and culture-aware)? True
Considerations for cultural differences in string comparisons are essential when developing globalized applications. By utilizing String.Compare
with CultureInfo
, you ensure that your application behaves appropriately across various cultures, accounting for language-specific rules and conventions.
This method is particularly valuable in scenarios where language-specific characters or collation orders impact string equality comparisons.
Conclusion
Mastering case-insensitive string comparisons in C# is a valuable skill for developers, enhancing the robustness and adaptability of their code. The choice between methods often depends on the specific requirements of the task at hand, considering factors such as readability, performance, and cultural considerations.
By exploring and understanding these techniques, developers can navigate the complexities of string handling with confidence, ensuring their applications perform accurate and consistent comparisons regardless of character case.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn