How to Slice An Array in C#
-
Array Slicing With the Linq Method in
C#
-
Array Slicing With the
ArraySegment
Class inC#
-
Array Slicing With an Extension Function in
C#
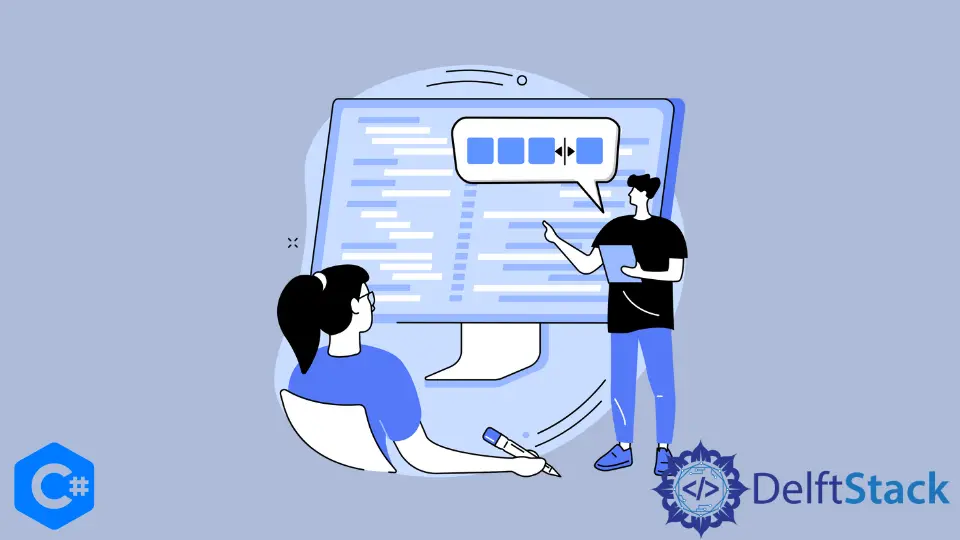
This tutorial will introduce the methods to slice an array in C#.
Array Slicing With the Linq Method in C#
The process of slicing down an array into a smaller sub-array is known as array slicing. The Linq is used to integrate query functionality with the data structures in C#. The Take(x)
function of Linq copies x
number of elements from the start of a data structure. The following code example shows us how we can slice an array with the Linq in C#.
using System;
using System.Linq;
namespace array_slicing {
class Program {
static void Main(string[] args) {
string[] foo = { "one", "two", "three", "four", "five" };
string[] bar = foo.Take(2).ToArray();
foreach (var e in bar) {
Console.WriteLine(e);
}
}
}
}
Output:
one
two
We initialized the array of strings foo
and sliced it into another array of strings - bar
, containing the first two elements of the foo
array. This method is very limited because we can only slice the array’s starting elements with this method.
Array Slicing With the ArraySegment
Class in C#
The ArraySegment
class is used to slice an array into a sub-array in C#. The Constructor of the ArraySegment
class takes the array to be segmented, the starting index, and the number of elements to be segmented and gives us a sub-array. The following code example shows us how we can slice an array with the ArraySegment
class in C#.
using System;
using System.Linq;
namespace array_slicing {
class Program {
static void Main(string[] args) {
string[] a = { "one", "two", "three", "four", "five" };
var segment = new ArraySegment<string>(a, 2, 3);
foreach (var s in segment) {
Console.WriteLine(s);
}
}
}
}
Output:
three
four
five
We initialized the array of strings a
and sliced it into a sub-array segment
containing 3 elements starting from index 2 of the a
array with the constructor of the ArraySegment
class. It is a better approach because we can slice an array from the middle with this approach.
Array Slicing With an Extension Function in C#
The extension methods allow us to add functions to existing data types without declaring a custom data type. We can also define a user-defined extension method instead of a built-in method for slicing an array in C#. This extension method can take the slice’s starting and ending index and return the sub-array within this range. The following code example shows us how to define an extension function to slice an array in C#.
using System;
using System.Collections.Generic;
namespace iterate_through_a_list {
public static class Extensions {
public static T[] Slice<T>(this T[] source, int start, int end) {
if (end < 0) {
end = source.Length + end;
}
int len = end - start;
T[] res = new T[len];
for (int i = 0; i < len; i++) {
res[i] = source[i + start];
}
return res;
}
}
class Program {
static void Main(string[] args) {
string[] values = { "one", "two", "three", "four", "five" };
string[] slice = values.Slice(2, 4);
foreach (var s in slice) {
Console.WriteLine(s);
}
}
}
}
Output:
three
four
five
We created an extension method, Slice()
, that takes the slice’s starting and ending index and returns the sliced sub-array. We initialized the array of strings values
and sliced it into the array slice
containing values from index 2
to index 4
of the values
array.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn