C# 中的数组切片
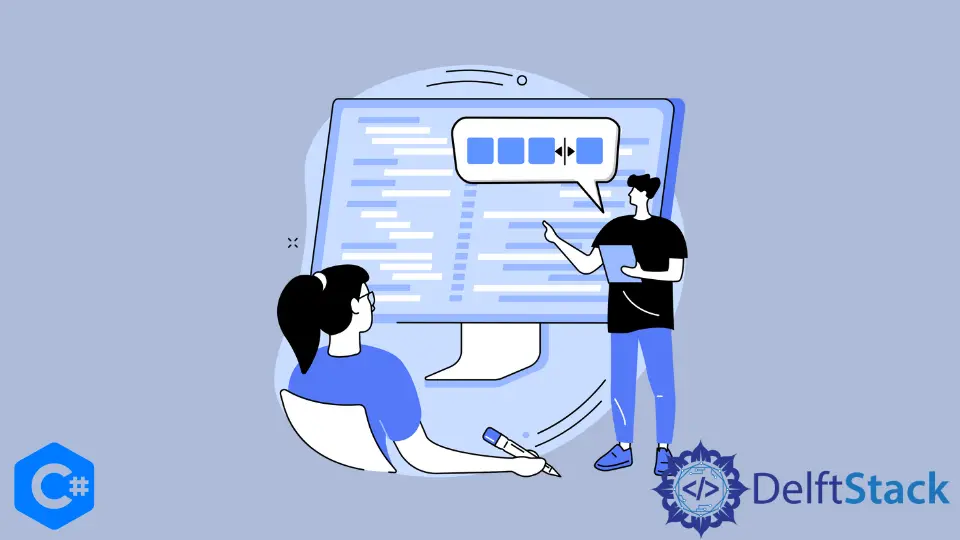
本教程将介绍在 C# 中切片数组的方法。
C# 中使用 Linq 方法进行数组切片
将数组切成更小的子数组的过程称为数组切分。Linq 用于将查询功能与 C# 中的数据结构集成在一起。Linq 的 Take(x)
函数从数据结构的开始复制 x
个元素。以下代码示例向我们展示了如何使用 C# 中的 Linq 切片数组。
using System;
using System.Linq;
namespace array_slicing {
class Program {
static void Main(string[] args) {
string[] foo = { "one", "two", "three", "four", "five" };
string[] bar = foo.Take(2).ToArray();
foreach (var e in bar) {
Console.WriteLine(e);
}
}
}
}
输出:
one
two
我们初始化了字符串 foo
的数组,并将其切成另一个字符串数组 bar
,其中包含 foo
数组的前两个元素。此方法非常有限,因为我们只能使用此方法对数组的起始元素进行切片。
使用 C# 中的 ArraySegment
类进行数组切片
在 C# 中,ArraySegment
类用来将一个数组切成一个子数组。ArraySegment
类的构造方法将要分割的数组,起始索引和要分割的元素数量提供给我们一个子数组。下面的代码示例向我们展示了如何使用 C# 中的 ArraySegment
类对数组进行切片。
using System;
using System.Linq;
namespace array_slicing {
class Program {
static void Main(string[] args) {
string[] a = { "one", "two", "three", "four", "five" };
var segment = new ArraySegment<string>(a, 2, 3);
foreach (var s in segment) {
Console.WriteLine(s);
}
}
}
}
输出:
three
four
five
我们初始化了字符串 a
的数组,并将其切成一个子数组 segment
,该子数组包含 3 个元素,这些元素从 a
数组的索引 2 开始,并带有 ArraySegment
类的构造函数。这是一种更好的方法,因为我们可以使用这种方法从中间对数组进行切片。
用 C# 中的扩展函数对数组进行切分
扩展方法使我们可以在不声明自定义数据类型的情况下向现有数据类型添加函数。我们还可以定义用户定义的扩展方法而不是用在 C# 中切片数组的内置方法。此扩展方法可以获取切片的开始和结束索引,并返回此范围内的子数组。以下代码示例向我们展示了如何定义扩展函数以在 C# 中对数组进行切片。
using System;
using System.Collections.Generic;
namespace iterate_through_a_list {
public static class Extensions {
public static T[] Slice<T>(this T[] source, int start, int end) {
if (end < 0) {
end = source.Length + end;
}
int len = end - start;
T[] res = new T[len];
for (int i = 0; i < len; i++) {
res[i] = source[i + start];
}
return res;
}
}
class Program {
static void Main(string[] args) {
string[] values = { "one", "two", "three", "four", "five" };
string[] slice = values.Slice(2, 4);
foreach (var s in slice) {
Console.WriteLine(s);
}
}
}
}
输出:
three
four
five
我们创建了一个扩展方法 Slice()
,该方法采用切片的开始和结束索引并返回切片的子数组。我们初始化了字符串 values
的数组,并将其切成数组 slice
,其中包含值从 values
数组的索引 2
到索引 4
的值。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn