How to Parse String Using a Delimiter in C++
- Using std::string and std::stringstream
- Using std::string::find and std::string::substr
-
Use the
copy()
Function to Parse String by a Single Whitespace Delimiter - Using Regular Expressions
- Conclusion
- FAQ
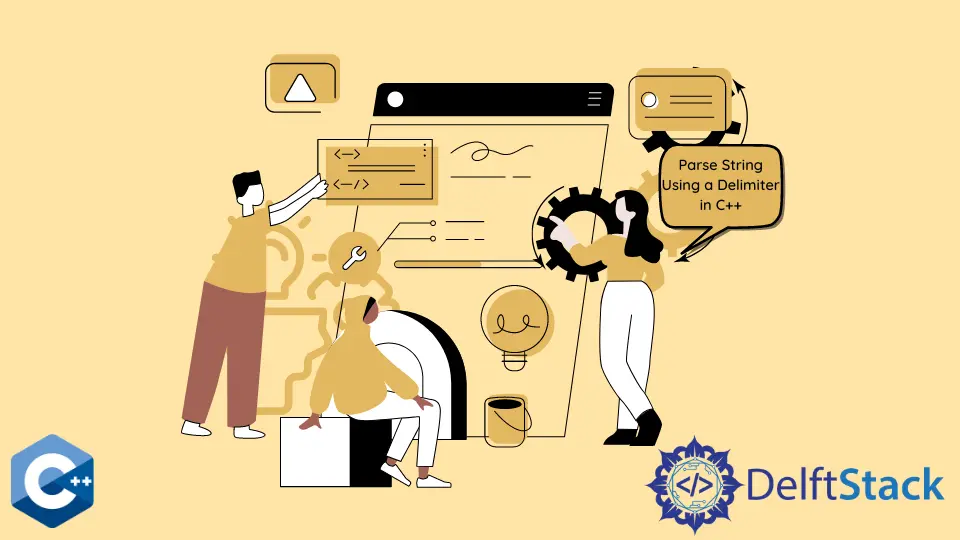
Parsing strings is a fundamental task in programming, and in C++, it can be accomplished effectively using delimiters. Whether you are dealing with CSV files, user input, or any other string data, knowing how to split strings based on specific characters is crucial.
In this article, we will explore various methods to parse strings using a delimiter in C++. We will cover techniques that leverage the Standard Library, as well as manual parsing methods. By the end, you will have a solid understanding of how to manipulate strings in C++ effectively. Let’s dive in!
Using std::string and std::stringstream
One of the most straightforward methods to parse a string in C++ is by using the std::string
class along with std::stringstream
. This approach allows you to read tokens from a string based on a specified delimiter. The std::stringstream
class makes it easy to treat a string like a stream, which can be very useful for parsing.
Here’s a simple example to demonstrate this:
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
std::vector<std::string> parseString(const std::string& str, char delimiter) {
std::vector<std::string> tokens;
std::stringstream ss(str);
std::string token;
while (std::getline(ss, token, delimiter)) {
tokens.push_back(token);
}
return tokens;
}
int main() {
std::string data = "apple,banana,cherry";
char delimiter = ',';
std::vector<std::string> result = parseString(data, delimiter);
for (const auto& fruit : result) {
std::cout << fruit << std::endl;
}
return 0;
}
Output:
apple
banana
cherry
In this code, we define a function parseString
that takes a string and a delimiter as arguments. We create a std::stringstream
object initialized with the input string. The std::getline
function reads each token separated by the specified delimiter and stores it in the tokens
vector. Finally, we print the parsed tokens in the main
function. This method is efficient and leverages the power of C++ Standard Library components.
Using std::string::find and std::string::substr
Another effective method for parsing strings is to use the std::string::find
and std::string::substr
functions. This approach gives you more control over how you handle the string, making it suitable for more complex parsing scenarios.
Here’s how you can implement this method:
#include <iostream>
#include <string>
#include <vector>
std::vector<std::string> parseString(const std::string& str, char delimiter) {
std::vector<std::string> tokens;
size_t start = 0;
size_t end = str.find(delimiter);
while (end != std::string::npos) {
tokens.push_back(str.substr(start, end - start));
start = end + 1;
end = str.find(delimiter, start);
}
tokens.push_back(str.substr(start));
return tokens;
}
int main() {
std::string data = "apple;banana;cherry";
char delimiter = ';';
std::vector<std::string> result = parseString(data, delimiter);
for (const auto& fruit : result) {
std::cout << fruit << std::endl;
}
return 0;
}
Output:
apple
banana
cherry
In this example, we define the parseString
function, which uses std::string::find
to locate the delimiter in the string. We then use std::string::substr
to extract the tokens between the delimiters. This method is particularly useful when you need to handle strings that may contain multiple delimiters or when you want to customize how tokens are extracted. The loop continues until no more delimiters are found, ensuring all tokens are captured.
Use the copy()
Function to Parse String by a Single Whitespace Delimiter
copy()
is a <algorithm>
library function, which can iterate through the specified range of elements and copy them to the destination range. At first, we initialize a istringstream
variable with the text
argument. After this, we utilize istream_iterator
to loop over whitespace-separated substrings and finally output them to the console. Notice, though, this solution only works if the string
needs to be split on whitespace delimiter.
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::vector;
int main() {
string text =
"He said. The challenge Hector heard with joy, "
"Then with his spear restrain'd the youth of Troy ";
istringstream iss(text);
copy(std::istream_iterator<string>(iss), std::istream_iterator<string>(),
std::ostream_iterator<string>(cout, "\n"));
return EXIT_SUCCESS;
}
The above code demonstrates an elegant way to split a string by whitespace using C++ standard library functions. It utilizes std::istringstream
to treat the input string as a stream of words, and std::copy
along with stream iterators to process and output each word. The std::istream_iterator<string>
is used to read words from the string stream, while std::ostream_iterator<string>
is used to write each word to the console, followed by a newline.
This approach is particularly useful for splitting strings on whitespace without explicitly managing loops or temporary containers. It showcases the power of combining stream operations with STL algorithms for concise and efficient string processing. However, it’s important to note that this method is limited to splitting on whitespace and doesn’t handle more complex delimiter scenarios.
Using Regular Expressions
For more complex string parsing, C++ offers the <regex>
library, which allows you to utilize regular expressions. This method is powerful and flexible, enabling you to define complex patterns for token extraction.
Here’s an example of using regular expressions to parse a string:
#include <iostream>
#include <regex>
#include <string>
#include <vector>
std::vector<std::string> parseString(const std::string& str, const std::string& regexPattern) {
std::vector<std::string> tokens;
std::regex regex(regexPattern);
std::sregex_token_iterator it(str.begin(), str.end(), regex, -1);
std::sregex_token_iterator reg_end;
while (it != reg_end) {
tokens.push_back(*it++);
}
return tokens;
}
int main() {
std::string data = "apple|banana|cherry";
std::string pattern = "\\|";
std::vector<std::string> result = parseString(data, pattern);
for (const auto& fruit : result) {
std::cout << fruit << std::endl;
}
return 0;
}
Output:
apple
banana
cherry
In this code, we define a function parseString
that takes a string and a regex pattern. The std::sregex_token_iterator
is used to iterate through the string, splitting it based on the provided regular expression. This method is particularly useful when dealing with complex delimiters or patterns, as it allows for greater flexibility in string parsing. Regular expressions can seem daunting at first, but they are incredibly powerful tools for string manipulation.
Conclusion
In summary, parsing strings using a delimiter in C++ can be accomplished through various methods, each with its advantages. Whether you choose to use std::stringstream
, std::string::find
and std::string::substr
, or regular expressions, understanding these techniques will enhance your ability to manipulate strings effectively. The choice of method often depends on the complexity of the data you are working with and your specific requirements. By mastering these techniques, you’ll be well-equipped to handle string parsing in your C++ projects.
FAQ
-
What is string parsing in C++?
String parsing in C++ refers to the process of breaking down a string into smaller, manageable parts based on specific delimiters or patterns. -
Why should I use std::stringstream for parsing?
Using std::stringstream is efficient and allows you to treat strings like streams, making it easier to read and extract tokens based on delimiters. -
Can I parse strings with multiple delimiters?
Yes, you can parse strings with multiple delimiters using techniques like regular expressions or by modifying the logic in your parsing function. -
Are regular expressions difficult to learn?
Regular expressions can be complex initially, but they are powerful tools for string manipulation once you understand the syntax and patterns. -
What is the best method for parsing strings in C++?
The best method depends on your specific use case. For simple tasks, std::stringstream is great, while regular expressions offer flexibility for more complex parsing scenarios.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++