如何在 C++ 中使用定界符解析字符串
Jinku Hu
2023年10月12日
C++
C++ String
-
使用
find()
和substr()
方法使用定界符来解析字符串 -
使用
stringstream
类和getline
方法使用定界符来解析字符串 -
使用
copy
函数通过单个空格分隔符解析字符串
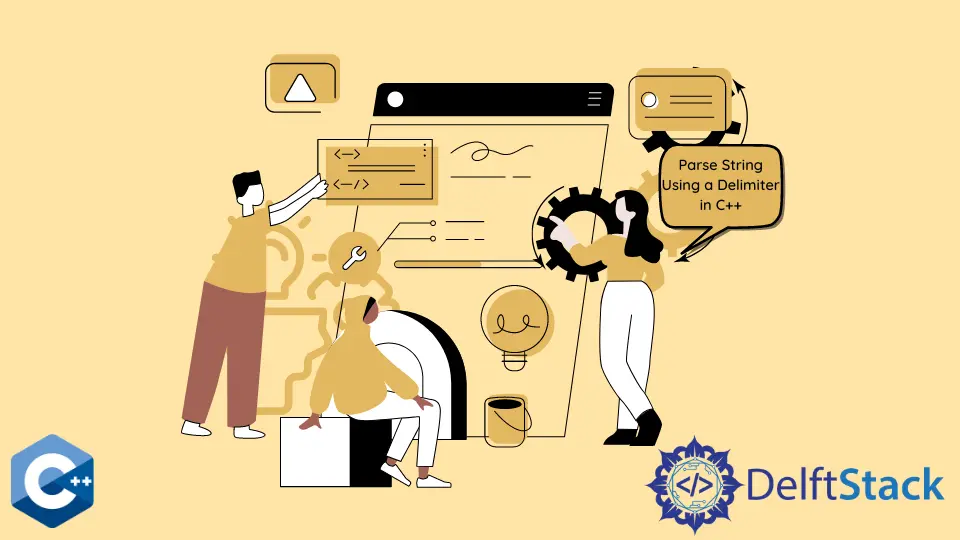
本文将说明如何通过在 C++ 中指定分隔符来解析字符串。
使用 find()
和 substr()
方法使用定界符来解析字符串
本方法使用字符串类的内置 find()
方法。它把一个要寻找的字符序列作为 string
类型,把起始位置作为一个整数参数。如果该方法找到了传递的字符,它返回第一个字符的位置。否则,它返回 npos
。我们将 find
语句放在 while
循环中,对字符串进行迭代,直到找到最后一个定界符。为了提取定界符之间的子字符串,使用 substr
函数,每次迭代时,将 token
推到 words
向量上。在循环的最后一步,我们使用 erase
方法删除字符串的处理部分。
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::vector;
int main() {
string text =
"He said. The challenge Hector heard with joy, "
"Then with his spear restrain'd the youth of Troy ";
string delim = " ";
vector<string> words{};
size_t pos = 0;
while ((pos = text.find(delim)) != string::npos) {
words.push_back(text.substr(0, pos));
text.erase(0, pos + delim.length());
}
for (const auto &w : words) {
cout << w << endl;
}
return EXIT_SUCCESS;
}
输出:
He
said.
The
...
Troy
使用 stringstream
类和 getline
方法使用定界符来解析字符串
在这个方法中,我们把 text
字符串变量放到 stringstream
中,用 getline
方法对其进行操作。getline
提取字符,直到找到给定的 char
,并将标记存储在 string
变量中。请注意,这个方法只能在需要单字符定界符的时候使用。
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text =
"He said. The challenge Hector heard with joy, "
"Then with his spear restrain'd the youth of Troy ";
char del = ' ';
vector<string> words{};
stringstream sstream(text);
string word;
while (std::getline(sstream, word, del)) words.push_back(word);
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
使用 copy
函数通过单个空格分隔符解析字符串
copy
是一个 <algorithm>
库函数,它可以遍历指定的元素范围,并将其复制到目标范围。首先,我们用 text
参数初始化一个 istringstream
变量。之后,我们利用 istream_iterator
来循环处理以空格分隔的子字符串,最后输出到控制台。但请注意,这个解决方案只有在 string
需要在白空格分隔符上进行分割时才有效。
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::vector;
int main() {
string text =
"He said. The challenge Hector heard with joy, "
"Then with his spear restrain'd the youth of Troy ";
istringstream iss(text);
copy(std::istream_iterator<string>(iss), std::istream_iterator<string>(),
std::ostream_iterator<string>(cout, "\n"));
return EXIT_SUCCESS;
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu