C++에서 구분 기호를 사용하여 문자열을 구문 분석하는 방법
-
구분 기호를 사용하여 문자열을 구문 분석하려면
find()
및substr()
메서드 사용 -
구분 기호를 사용하여 문자열을 구문 분석하려면
stringstream
클래스 및getline
메서드 사용 -
copy()
함수를 사용하여 단일 공백 구분 기호로 문자열 구문 분석
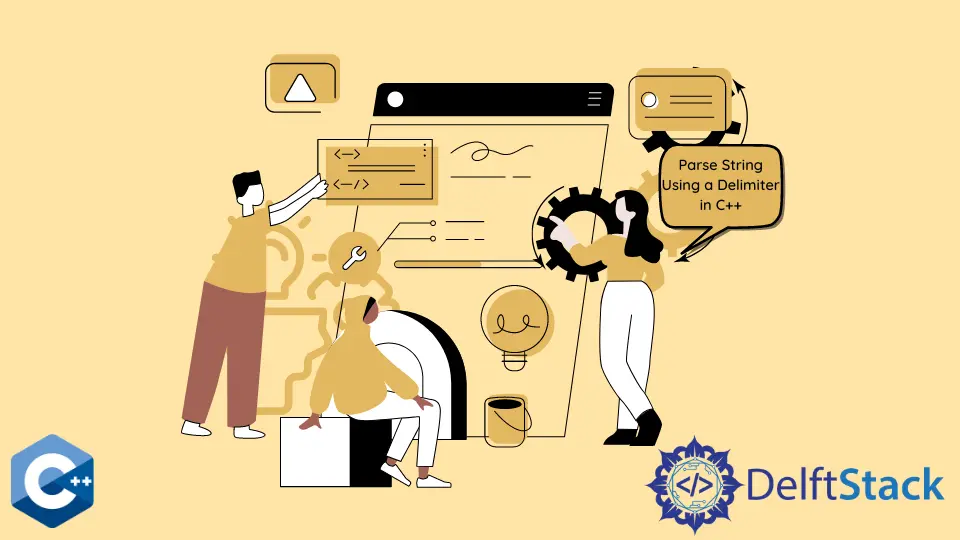
이 기사에서는 C++에서 구분 기호를 지정하여 문자열을 구문 분석하는 방법을 설명합니다.
구분 기호를 사용하여 문자열을 구문 분석하려면find()
및substr()
메서드 사용
이 메소드는string
클래스의 내장find
메소드를 사용합니다. ‘문자열’유형으로 검색되는 일련의 문자와 정수 매개 변수로 시작 위치가 필요합니다. 메서드가 전달 된 문자를 찾으면 첫 번째 문자의 위치를 반환합니다. 그렇지 않으면npos
를 반환합니다. 마지막 구분 기호를 찾을 때까지string
을 반복하기 위해while
루프에find
문을 넣습니다. 구분자 사이의 하위 문자열을 추출하기 위해 substr
함수를 사용하고 각 반복에서 토큰을 ‘단어’에 푸시합니다. 루프의 마지막 단계로 erase
메소드를 사용하여 문자열의 처리 된 부분을 제거합니다.
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::vector;
int main() {
string text =
"He said. The challenge Hector heard with joy, "
"Then with his spear restrain'd the youth of Troy ";
string delim = " ";
vector<string> words{};
size_t pos = 0;
while ((pos = text.find(delim)) != string::npos) {
words.push_back(text.substr(0, pos));
text.erase(0, pos + delim.length());
}
for (const auto &w : words) {
cout << w << endl;
}
return EXIT_SUCCESS;
}
출력:
He
said.
The
...
Troy
구분 기호를 사용하여 문자열을 구문 분석하려면stringstream
클래스 및getline
메서드 사용
이 메서드에서는text
문자열 변수를stringstream
에 넣어getline
메서드로 작업합니다. getline
은 주어진char
를 찾을 때까지 문자를 추출하고string
변수에 토큰을 저장합니다. 이 방법은 단일 문자 구분 기호가 필요한 경우에만 적용 할 수 있습니다.
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text =
"He said. The challenge Hector heard with joy, "
"Then with his spear restrain'd the youth of Troy ";
char del = ' ';
vector<string> words{};
stringstream sstream(text);
string word;
while (std::getline(sstream, word, del)) words.push_back(word);
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
copy()
함수를 사용하여 단일 공백 구분 기호로 문자열 구문 분석
copy()
는<algorithm>
라이브러리 함수로, 지정된 요소 범위를 반복하고 대상 범위에 복사 할 수 있습니다. 처음에는text
인수로istringstream
변수를 초기화합니다. 그런 다음 istream_iterator
를 사용하여 공백으로 구분 된 하위 문자열을 반복하고 마지막으로 콘솔에 출력합니다. 그러나이 솔루션은 문자열을 공백 구분 기호로 분할해야하는 경우에만 작동합니다.
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::vector;
int main() {
string text =
"He said. The challenge Hector heard with joy, "
"Then with his spear restrain'd the youth of Troy ";
istringstream iss(text);
copy(std::istream_iterator<string>(iss), std::istream_iterator<string>(),
std::ostream_iterator<string>(cout, "\n"));
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook