C++ でデリミタを使用して文字列を解析する方法
胡金庫
2023年10月12日
C++
C++ String
-
区切り文字を用いて文字列を解析するには
find()
とsubstr()
メソッドを用いる -
stringstream
クラスとgetline
メソッドを用いてデリミタを用いて文字列を解析する -
文字列を単一の空白区切り文字で解析するには
copy()
関数を使用する
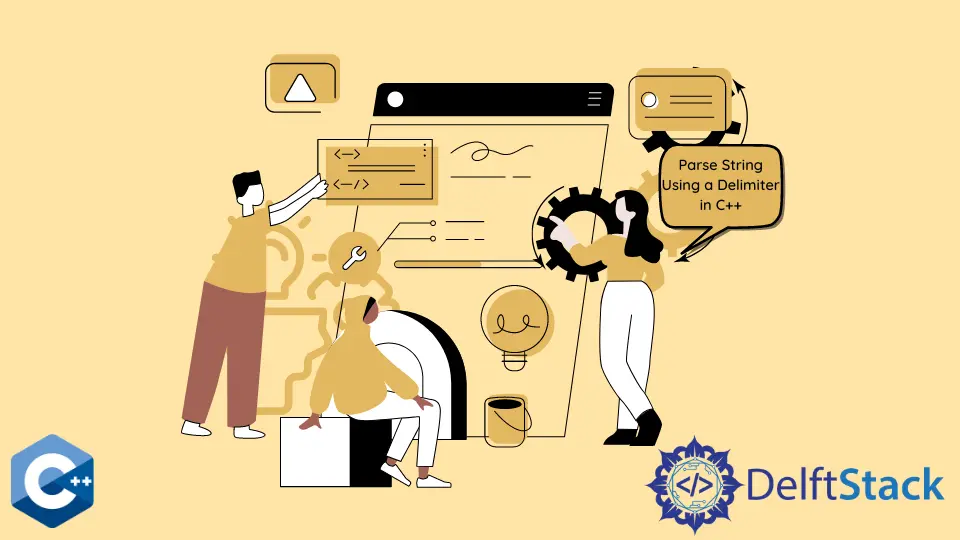
この記事では、C++ でデリミタを指定して文字列を解析する方法を説明します。
区切り文字を用いて文字列を解析するには find()
と substr()
メソッドを用いる
このメソッドは string
クラスの組み込みの find
メソッドを用います。このメソッドは検索対象の文字列を string
型で受け取り、開始位置を整数値のパラメータとします。メソッドは渡された文字を見つけた場合、最初の文字の位置を返します。そうでなければ npos
を返します。最後の区切り文字が見つかるまで string
を繰り返し処理するために、while
ループの中に find
文を入れる。区切り文字の間の部分文字列を抽出するには、substr
関数を用い、繰り返しのたびにトークンを words
ベクトルにプッシュします。ループの最後のステップとして、erase
メソッドを用いて文字列の処理部分を削除します。
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::vector;
int main() {
string text =
"He said. The challenge Hector heard with joy, "
"Then with his spear restrain'd the youth of Troy ";
string delim = " ";
vector<string> words{};
size_t pos = 0;
while ((pos = text.find(delim)) != string::npos) {
words.push_back(text.substr(0, pos));
text.erase(0, pos + delim.length());
}
for (const auto &w : words) {
cout << w << endl;
}
return EXIT_SUCCESS;
}
出力:
He
said.
The
...
Troy
stringstream
クラスと getline
メソッドを用いてデリミタを用いて文字列を解析する
このメソッドでは、文字列変数 text
を stringstream
に入れて getline
メソッドで操作します。getline
は与えられた char
が見つかるまで文字を抽出し、そのトークンを string
変数に格納します。このメソッドは 1 文字の区切り文字が必要な場合にのみ適用できることに注意してください。
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text =
"He said. The challenge Hector heard with joy, "
"Then with his spear restrain'd the youth of Troy ";
char del = ' ';
vector<string> words{};
stringstream sstream(text);
string word;
while (std::getline(sstream, word, del)) words.push_back(word);
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
文字列を単一の空白区切り文字で解析するには copy()
関数を使用する
copy()
は <algorithm>
ライブラリ関数であり、指定された範囲の要素を繰り返し処理してコピー先の範囲にコピーすることができます。まず、引数 text
で istringstream
変数を初期化します。その後、istream_iterator
を利用して、空白で区切られた部分文字列をループさせ、最終的にコンソールに出力します。しかし、この解決策は string
が空白区切り文字で分割される必要がある場合にのみ動作することに注意してください。
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::vector;
int main() {
string text =
"He said. The challenge Hector heard with joy, "
"Then with his spear restrain'd the youth of Troy ";
istringstream iss(text);
copy(std::istream_iterator<string>(iss), std::istream_iterator<string>(),
std::ostream_iterator<string>(cout, "\n"));
return EXIT_SUCCESS;
}
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: 胡金庫