A Declaration Shadows a Parameter Error in C++
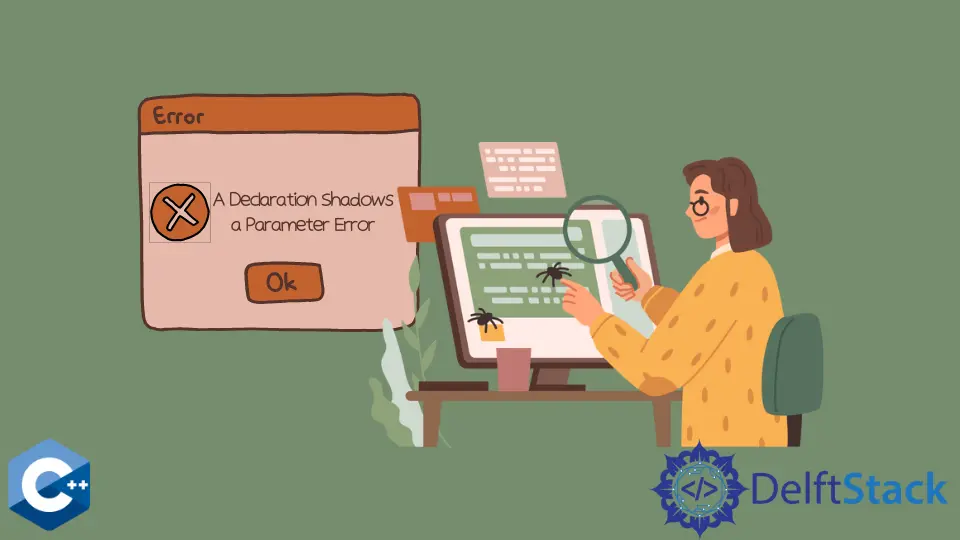
There are always some boundaries, ranges, or scope of every object or variable to access other class members like data members or member functions defined by access specifiers in C++ as public
, private
, or protected
.
When we define a variable more than once with the same names in the specific scope or block of the program, the compilers confused and throw’s an error saying a declaration shadows a parameter
.
Access Specifiers in C++
Before going into the details of the shadow parameter, it’s important to understand access specifiers. Generally, three access specifiers are public
, private
, and protected
.
Public Access Specifier in C++
A Public
specifier makes all the members (Data members or member functions) of a class public, which can be accessed throughout the program from other classes; you can directly access those member functions using the dot .
operator with the object of that same class.
#include <iostream>
using namespace std;
// class definition
class Cal_Circle {
public: // Public access specifier
double radius;
double compute_area() { return 3.14 * radius * radius; }
};
// main function
int main() {
Cal_Circle obj;
// accessing public datamember outside class
obj.radius = 3.4;
cout << "Radius of the circle is: " << obj.radius << "\n";
cout << "Area of the circle is: " << obj.compute_area();
return 0;
}
Output:
Radius of the circle is: 3.4
Area of the circle is: 36.2984
Private Access Specifier in C++
Private
access specifier keeps every member, either data member or member function, private within the boundaries of a class. They cannot be accessed outside of the class directly by the object of that class.
They are hidden from access outside the class to ensure the program’s security.
#include <iostream>
using namespace std;
// class definition
class Cal_Circle {
private: // private access specifier
double radius;
double compute_area() { return 3.14 * radius * radius; }
};
// main function
int main() {
Cal_Circle obj;
// accessing public datamember outside class
obj.radius = 3.4;
cout << "Radius of the circle is: " << obj.radius << "\n";
cout << "Area of the circle is: " << obj.compute_area();
return 0;
}
Output:
error: 'double Cal_Circle::radius' is private within this context
obj.radius = 3.4;
note: declared private here
double radius;
note: declared private here
double compute_area(){
See in this example, we have used the accessed specifier as private
and accessed it through the object of the class from the main function. And it’s throwing an error for each class member that is declared protected here.
Protected Access Specifier in C++
Similarly, the Protected
access specifier cannot be accessed outside of its class directly through the object of that class, but the difference is that the Protected
class or members can be accessed through derive class.
Using the object of the derived class, you can access the protected members of a class.
#include <iostream>
using namespace std;
class Radius { // Base class
protected: // protected access specifier
double radius;
};
class Cal_Circle : public Radius { // Derive class
public: // private access specifier
double compute_area(int a) {
radius = a;
return 3.14 * radius * radius;
}
};
// main function
int main() {
Cal_Circle obj;
// accessing public datamember outside class
cout << "Area of the circle is: " << obj.compute_area(3);
return 0;
}
Output:
Area of the circle is: 28.26
Our base class is protected and has one data member radius
. We have inherited that base class to Cal_Circle
, and with the object of the derived class, we are accessing the protected data member radius
.
We are indirectly accessing the protected members of a protected class.
Declaration Shadows a Parameter
Error in C++
In computer programming, there are certain boundaries known as scope. This could be an if-else
block, a function, or a class.
A variable defined inside an if-else
block, function, or a class cannot be used unless and until you have defined it as public
. And a public variable, function, or class can be accessed throughout the program.
Also, it is prohibited in C++ to define one variable two times or more within a specific scope. And when you do it, an error is thrown as a declaration shadows a parameter
.
Let’s understand it through an example.
#include <iostream>
int doublenumber();
using namespace std;
int doublenumber(int x) {
int x; // We are redefining the same variable as the parameter of the
// function
return 2 * x;
cout << endl;
}
int main() { // Main function
int a;
cout << "Enter the number that you want to double it : " << endl;
cin >> a;
int d = doublenumber(a);
cout << "Double : " << d << endl;
return 0;
}
Output:
Test.cpp: In function 'int doublenumber(int)':
Test.cpp:6:9: error: declaration of 'int x' shadows a parameter
int x;
As we have seen, we cannot define one variable more than once in a specific block of code like function, class, and an if-else
block.
In the doublenumber()
function, we have a parameter int x
, and in the scope of this function, we are also defining another local int x
, which is shadowing the parameter and throwing an error.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn