How to Fix the C++ Error: Redefinition of Default Parameter
-
Understanding the
"redefinition of default parameter"
Error in C++ - Specify Arguments in the Function Declaration to Handle Redefinition of Default Parameter Error in C++
- Specify Arguments in the Function Definition to Handle the Redefinition of Default Parameter Error in C++
- Use Function Overloading to Resolve Redefinition of Default Parameter Error in C++
- Use Function Prototypes and Definitions Carefully to Resolve Redefinition of Default Parameter Error in C++
- Conclusion
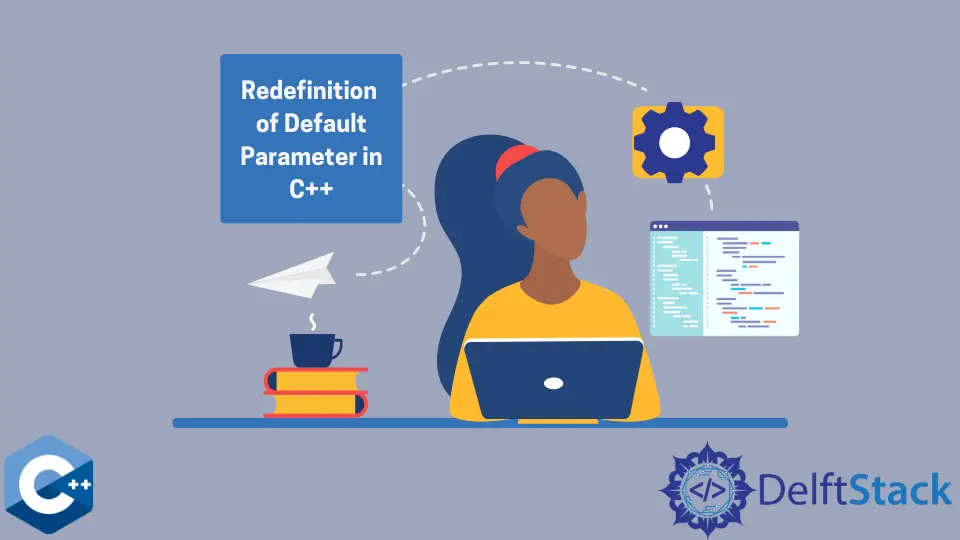
The "redefinition of default parameter"
error in C++ occurs when you attempt to provide a default value for a function parameter in multiple places, such as within both the function declaration and its definition. This conflict leads to ambiguity for the compiler and results in the error.
Resolving this issue involves understanding several methods that can be employed to rectify or work around this problem.
In this tutorial, you’ll learn how to handle the redefinition of default parameter errors in C++. The default arguments in C++ must be specified in either the method or function’s declaration or definition but not in both because of duplication.
Understanding the "redefinition of default parameter"
Error in C++
C++ offers powerful features like default parameters in function declarations, enabling flexibility and simplicity in function calls. However, encountering the "redefinition of default parameter"
error can be puzzling for developers.
In C++, default parameters allow function declarations to specify default values for parameters. However, redefining default parameters in function declarations or definitions causes the compiler to raise an error.
This error occurs when a function’s default parameters are specified in both its declaration and definition, leading to ambiguity.
Specify Arguments in the Function Declaration to Handle Redefinition of Default Parameter Error in C++
The most straightforward approach is to have a single declaration of the default parameter. Ensure that the default value is only provided in one place, typically in the function declaration or definition.
// Function Declaration
void exampleFunction(int a, int b = 10); // Default parameter declared here
// Function Definition
void exampleFunction(int a, int b) {
// Function body
// The default value is not redeclared here
}
What causes the redefinition of default parameter
error is its redefinition in the header file, which will be invoked each time from a parent file and lead to redefining the function or method multiple times.
However, you can add default arguments in later declarations of a function (or method) in the same scope for non-template functions (or methods). Declarations have completely distinct sets of default arguments in different scopes.
Each parameter after a parameter with a default argument shall have a default argument supplied, a previous declaration, or a function parameter pack in a given function declaration. The redefinition of default parameter
error occurs when you set default parameters in the definition (implementation), not only in the declaration.
Code Example:
#include <iostream>
// to process variables whenever called
void print(int x, int y) {
std::cout << "x: " << x << '\n'; // x output
std::cout << "y: " << y << '\n'; // y output
}
int main() {
// function declaration
void print(int x, int y = 3);
print(7, 4); // in this case, `y` is user-defined variable hence default
// argument will be neglected
print(6); // in this case, `y` has no user-defined value hence, it will use
// default argument
}
The code defines a function print
that takes two integer parameters. Inside the main
function, print
is redeclared with a default value for the second parameter.
When calling print
, if both parameters are provided, the default value is ignored. If only one parameter is given, the default value is used for the second parameter.
Output:
x: 7
y: 4
x: 6
y: 3
If there is more than one parameter in your function (or method), then those that are defaulted must be listed after all non-defaulted parameters like void my_function (int a, char b);
.
In C++, default arguments do not directly cause tarpits of Boolean parameters, but these arguments make their pernicious effects worse, and as a result, Boolean tarpits become extremely complex to handle.
Specify Arguments in the Function Definition to Handle the Redefinition of Default Parameter Error in C++
A function call can retrieve the arguments for any function parameter from the caller if any of the function parameters have a default argument, and in case the caller doesn’t provide an argument, the value of the default argument of that particular parameter is used instead.
Once declared, a default argument cannot be redeclared because of the duplication, which means the default arguments can be declared in a function definition.
The forward declaration of a default parameter is one of the best practices for declaring a default argument instead of declaring it in the function definition.
Code Example:
#include <iostream>
void print(int x, int y = 4) // 4 is the default argument
{
std::cout << "x: " << x << '\n';
std::cout << "y: " << y << '\n';
}
int main() {
print(1, 2); // y will use user-supplied argument 2
print(3); // y will use default argument 4
}
// in case of multiple default arguments
void printmulti(int x = 10, int y = 20, int z = 30) {
std::cout << "x: " << x << '\n';
std::cout << "y: " << y << '\n';
std::cout << "z: " << z << '\n';
}
In the print
function, there are two parameters, x
and y
, with y
having a default value of 4
. The main
function demonstrates two calls to print
: one supplying both arguments (print(1, 2)
), assigning x
as 1
and y
as 2
, and another with only x
provided (print(3)
), where y
uses the default value of 4
.
Moreover, a function printmulti
showcases multiple default arguments (x = 10
, y = 20
, z = 30
). When invoked without arguments, it utilizes these defaults for x
, y
, and z
.
Output:
x: 1
y: 2
x: 3
y: 4
A default argument appears syntactically in a function (or method) declaration, but the default argument itself behaves like a definition in that there must be only one of it per translation unit.
You aren’t allowed to repeat the default argument’s value in the function definition, which means a piece of the function’s interface is invisible at the site of its implementation.
Default argument’s definition in one place and evaluation in another leads to confusion about aspects of C++ in the middle, such as name lookup, access control, and template instantiation.
Use Function Overloading to Resolve Redefinition of Default Parameter Error in C++
Another approach to circumvent the error involves function overloading, providing multiple versions of the function with different parameter lists, including default parameters.
Function overloading in C++ involves creating multiple functions with the same name but differing parameter lists. Leveraging function overloading provides a viable strategy to bypass the "redefinition of default parameter"
error by offering distinct function signatures with unique default parameter configurations.
Consider the following code snippet illustrating function overloading to resolve the error:
#include <iostream>
// Function declaration with default parameter
void display(int value, int defaultValue);
// Overloaded function with default parameter
void display(int value) {
display(value, 10); // Calls the function with the default parameter
}
int main() {
display(5); // Calls the function with the default parameter
display(7, 20); // Calls the function with a different value
return 0;
}
// Function definition with default parameter
void display(int value, int defaultValue) {
std::cout << "Value: " << value << ", Default: " << defaultValue << std::endl;
}
In this example, function overloading is utilized to circumvent the error. Two display
functions are declared: one with a single parameter and another with two parameters.
The single-parameter display
function acts as a wrapper, calling the overloaded version with both parameters and utilizing the default parameter configuration.
Output:
Value: 5, Default: 10
Value: 7, Default: 20
Use Function Prototypes and Definitions Carefully to Resolve Redefinition of Default Parameter Error in C++
Ensure consistency between function prototypes and their corresponding definitions, abstaining from specifying default parameters in both. Make certain that declarations and definitions mirror each other accurately.
Example:
#include <iostream>
// Function prototype with default parameter
void display(int value, int defaultValue = 10);
int main() {
display(5); // Calls the function with the default parameter
display(7, 20); // Calls the function with a different value
return 0;
}
// Function definition without default parameter
void display(int value, int defaultValue) {
std::cout << "Value: " << value << ", Default: " << defaultValue << std::endl;
}
In this case, the function prototype specifies the default parameter, while the function definition doesn’t include a default value for the parameter. This alignment between declaration and definition avoids the redefinition error.
Output:
Value: 5, Default: 10
Value: 7, Default: 20
Conclusion
In C++, the "redefinition of default parameter"
error occurs when default parameters are redundantly defined in both function declarations and definitions. Resolving this error involves several strategies:
- Single Declaration: Specify default parameters in function prototypes but not in both the prototype and definition. This ensures clarity and alignment between declaration and definition.
- Avoid Redundant Declarations: Refrain from redundant default parameter declarations across header files and multiple inclusions. Declare default arguments in forward declarations and define them once to mitigate redefinition issues.
- Utilize Function Overloading: Leverage function overloading to create multiple function versions with different parameter lists, including default parameters. This approach offers distinct function signatures and resolves redefinition errors.
- Careful Usage of Prototypes and Definitions: Maintain consistency between function prototypes and definitions. Avoid duplicating default parameter declarations in both prototypes and definitions to ensure clarity and prevent errors.
Adhering to these strategies enables developers to effectively address the "redefinition of default parameter"
error, ensuring cleaner and error-free C++ code.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub