How to Solve Error - Python.h: No Such File or Directory in C++
-
Causes of
'Python.h': No such file or directory
Error in C++ - Python Installation That Allows Embedding Its Code in C++
-
Steps to Add Python Path to IDE’s
Include
and Linker -
Add Python Path to
Include
and Linker - Write Python Codes in C++ and Compile Them
- Conclusion
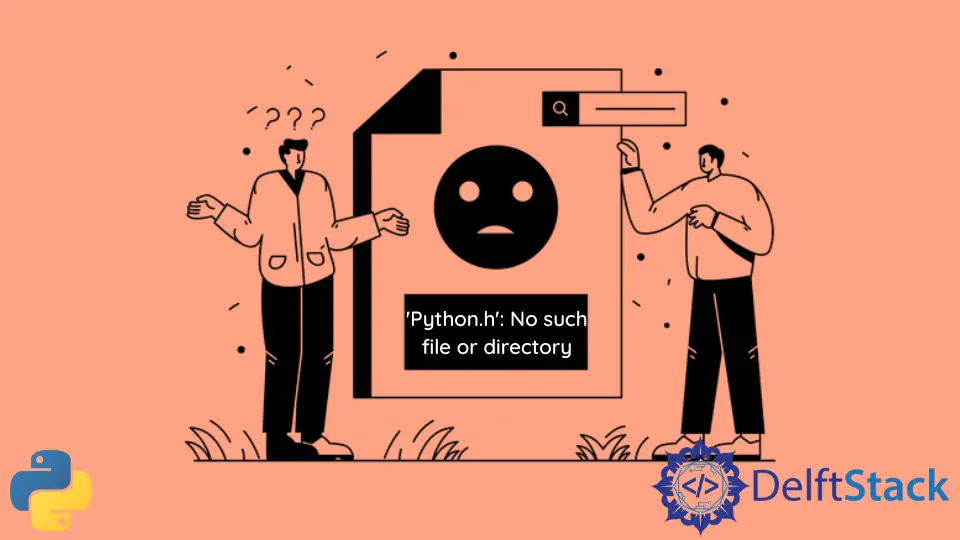
This article will explain how to solve the error 'Python.h': No such file or directory
. This usually happens when we try to embed Python code in C++, but the compiler cannot find a reference to Python inside the system.
Causes of 'Python.h': No such file or directory
Error in C++
Below is a C++ program that uses Python codes.
#include <Python.h>
#include <conio.h>
#include <stdio.h>
int main() {
PyObject* pInt;
Py_Initialize();
PyRun_SimpleString("print('Hello World from Embedded Python!!!')");
Py_Finalize();
printf("\nPress any key to exit...\n");
if (!_getch()) _getch();
return 0;
}
When this program is compiled, it gives the error 'Python.h': No such file or directory
.
Build started...
1>------ Build started: Project: Python into Cpp, Configuration: Debug x64 ------
1>1.cpp
1>C:\Users\Win 10\source\repos\Python into Cpp\1.cpp(3,10): fatal error C1083: Cannot open include file: 'Python.h': No such file or directory
1>Done building project "Python into Cpp.vcxproj" -- FAILED.
========== Build: 0 succeeded, 1 failed, 0 up-to-date, 0 skipped ==========
Various reasons cause the 'Python.h': No such file or directory
issue.
-
Python is not installed inside the system.
Python is third-party enterprise software that does not come with standard Windows and C++ compilers installation. It must be installed with correct settings and linked to the C++ compiler.
-
C++ compiler cannot find Python.
If the compiler is run through an IDE or mingw4, it can only detect that standard C++ includes packages that come with a standard installation.
Third-party libraries are needed to be added to the IDE
include
and linker files.Three steps need to be executed to solve the above issues.
- Custom installing Python that allows it to be embedded in IDEs.
- Adding Python path to IDE
include
and linker paths. - Writing Python codes inside C++ codes and compiling them.
Python Installation That Allows Embedding Its Code in C++
If there is no Python inside the system, or if it is installed and linked but still the error 'Python.h': No such file or directory
occurs, it’s because the Python installation did not download the debug binaries with it.
It is recommended that Python be uninstalled and then reinstalled correctly to solve the error 'Python.h': No such file or directory
.
Head to the Python website and download the latest Python version. If a certain version is required, head to the All Releases
download section.
This article demonstrates the steps in Windows OS, so the suitable option is to go with the Windows installer(64bit or 32bit)
. It is recommended to use the 64-bit version if a modern PC is used.
After the download is complete, run the installer, and choose Customize Installation
. The Install now
option will install Python with default settings but leave the debug binaries out that allows embedding it into IDEs.
Tick the box that asks to Add Python X.X(version) to PATH
and then click on Customize Installation
.
On the next page, mark all the boxes and then click next.
In the third menu, tick the box Download debug binaries
and click on Install
. Python will be installed inside the selected directory.
Steps to Add Python Path to IDE’s Include
and Linker
Any third-party library that does not come with C++ in its standard installation must be referenced to the IDE. IDEs cannot automatically search and detect third-party libraries inside the system.
This is the reason that causes the error 'Python.h': No such file or directory
.
Though IDE can search for files when the package library is stored in the same directory where the C++ script is located, its path should be mentioned inside the program’s #include<>
header.
But storing dependencies this way is a bad practice.
What needs to be done is that the path of the library should be linked to the IDE so that it can be included the way standard header files are included, removing the need to provide a file path with the source code.
Any third-party library that does not come with C++ in its standard installation must be referenced to the IDE. IDEs cannot automatically search and detect third-party libraries inside the system.
This is the reason that causes the error 'Python.h': No such file or directory
.
At first, an IDE is needed. It is recommended to go with Visual Studio (2017 or later), and this article will cover the same.
Any other IDE can also be used, the steps are identical in most of them, but Visual Studio makes it simple.
Install Visual Studio in the System
The installer of Visual Studio can be downloaded from here. After downloading the 64-bit Community version, install the software, including the C++ development option.
After installation is completed, create a new project.
The window can look intimidating for readers who have never used Visual Studio before, but there’s no need to worry. Most of the features that will be needed are in front, and this article will guide the rest.
Create a project, and give it a name like ‘Python in cpp’. After the project is created, the main window would look something like this:
If there is just a black screen, press Ctrl+Alt+l, and the solution explorer will open. The solution explorer shows all the files and dependencies available for the project.
Add Python Path to Include
and Linker
In this step, the Python path will be included and linked to Visual Studio so that the IDE knows where to look when #include <python.h>
is written.
A prerequisite for this step is to know the directory where Python is installed and keep it open in another window. Now head down to Debug
> Project Name
> Properties
(Instead of Project name
, it will display the project’s name).
Inside the project properties window, go to C/C++
> General
> Additional Include Directories
, click on the tiny drop button on the extreme right, and then click on <Edit...>
.
The Additional Include Directories
dialog box will open. Here, double-click on the first blank space and paste the path of the include
folder from the directory where Python is installed inside the system, then click OK
.
After the path of include
directory is added, head down to Linker
> General
> Additional Library Directories
. Like the previous step, click on <Edit...>
.
Now, the libs
folder should be added to the linker. This folder is also found inside the Python directory, where the include
folder is located.
After it is added, click on OK
and then click on Apply
.
Write Python Codes in C++ and Compile Them
Once the include
and linker paths are added, a sample program must be compiled to check whether the above steps have been executed correctly, and the 'Python.h': No such file or directory
error is not encountered again.
Go to the solution explorer and right-click on source files. Click on add
> new item
, give the file a name and click add.
This new file will contain the code that will be compiled.
Below is the C++ program used at the start of the article that threw errors.
#include <Python.h>
#include <conio.h>
#include <stdio.h>
int main() {
PyObject* pInt;
Py_Initialize();
PyRun_SimpleString("print('Hello World from Embedded Python!!!')");
Py_Finalize();
printf("\nPress any key to exit...\n");
if (!_getch()) _getch();
return 0;
}
Now, when the file is compiled by clicking on the green play button on the top, we get the output:
Hello World from Embedded Python!!!
Press any key to exit...
Conclusion
This article explains the steps to solve the error 'Python.h': No such file or directory
. After reading this article, the reader can install Python and Visual Studio and link them to the Python library.