Error: Cannot Call Member Function Without Object in C++
-
Fix the
error: cannot call member function without object
in C++ - Use an Instance of the Class to Access the Member Functions
- Use Static Member Functions
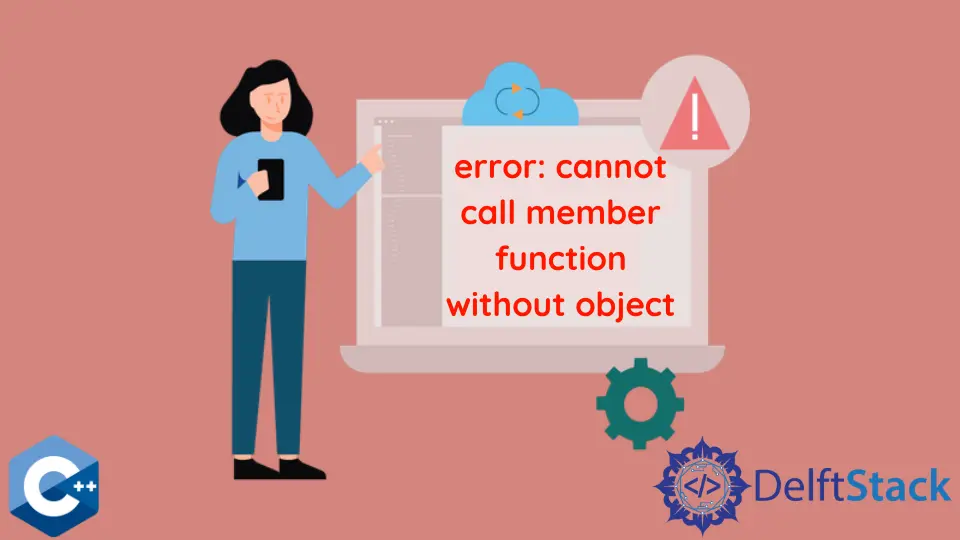
This article describes the commonly occurring error cannot call member function without object
while doing object-oriented programming using C++. Furthermore, it also provides potential fixes to the error.
Fix the error: cannot call member function without object
in C++
A common error in C++ frequently occurs when working in object-oriented programming, stating cannot call member function without object
. The cause of this error is that you are calling some member method of the class without instantiating the class.
Every class has a set of data members and some member functions. We need to create the class object to access the member methods or functions and then call/access the methods using this object.
Consider the following code.
#include <iostream>
using namespace std;
class Rectangle {
private:
int length = 5;
int width = 8;
public:
double getArea() { return length * width; }
};
int main() { cout << "Area: " << Rectangle::getArea() << endl; }
This code will generate the following output.
Line #16 in the above code snippet tries to call the getArea()
method using the class name. All the non-static members of a class must only be accessed through an object of the class; therefore, the line generates the error.
Use an Instance of the Class to Access the Member Functions
The error can be solved by calling the function/method with an object of the class like this:
int main() {
Rectangle r;
cout << "Area: " << r.getArea() << endl;
}
This will give the correct output.
Area: 40
Use Static Member Functions
Static member functions are the functions of a class that do not need an object to call them. They can be called directly with the class name using the scope resolution operator ::
.
Certain limitations must be kept in mind when using the static member functions. A static member function can access only the static data members of the class and can only call the other static member functions.
Let’s look at the example below discussing the solution to the cannot call member function without object
error.
#include <iostream>
using namespace std;
class Rectangle {
private:
int length = 5;
int width = 8;
public:
double getArea() { return length * width; }
static void getShapeName() { cout << "Hello, I am a Rectangle." << endl; }
};
int main() {
Rectangle r;
cout << "Area: " << r.getArea() << endl;
Rectangle::getShapeName();
}
This will give the following output.
Area: 40
Hello, I am a Rectangle.