How to Jump to Case Label in the switch Statement
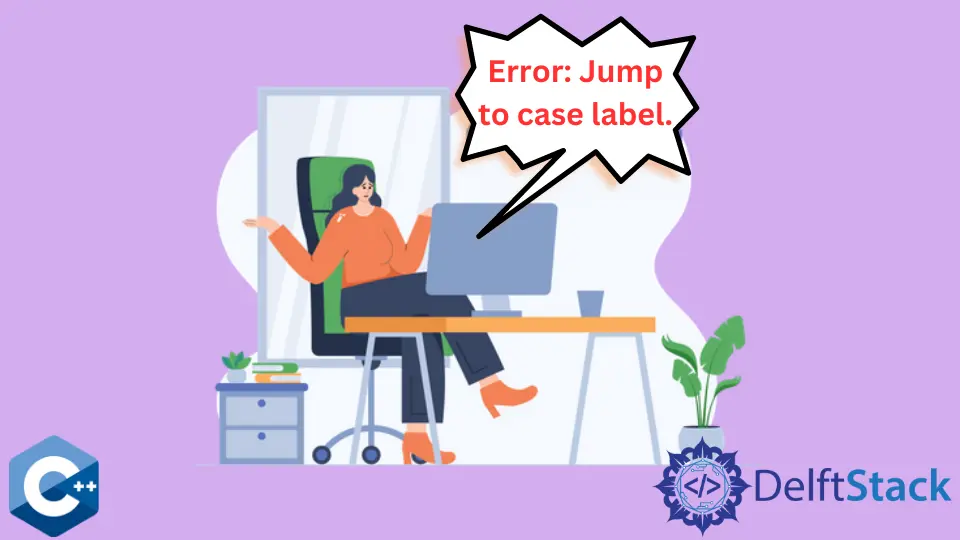
This article will discuss the use of switch
statements in C++. Moreover, it will discuss errors that may arise while using the switch
statement, including the Jump to case label
error.
the switch
Statement in C++
The switch
statement evaluates a given Boolean or integer expression and executes the statement(s) associated with the cases
based on the evaluation of the given expression. It is the best alternative to the lengthy if-else-if
statements as it reduces the code length and enhances clarity.
In C/C++, the following syntax is used for the switch
statement to perform the evaluation.
Syntax:
switch (exp) {
case a:
// Block of code
break;
case b:
// Block of code
break;
default:
// Block of code
}
The switch
statement works in the following way:
- The expression is evaluated once in the
switch
statement. - The
case
value is compared to theswitch
value. - After evaluating the
switch
expression with acase
statement, the block of code subsequent to the matchedcase
is executed if the condition is true. - The
break
anddefault
keywords are optional with theswitch
statement. We’ll discuss these in detail at the end of this tutorial.
Suppose we want to calculate the weekday name from the weekday number.
Example Code:
#include <iostream>
using namespace std;
int main() {
int weak_day = 3;
switch (weak_day) {
case 1:
cout << "Monday";
break;
case 2:
cout << "Tuesday";
break;
case 3:
cout << "Wednesday";
break;
case 4:
cout << "Thursday";
break;
case 5:
cout << "Friday";
break;
case 6:
cout << "Saturday";
break;
case 7:
cout << "Sunday";
break;
default:
cout << "Invalid input";
}
}
Output:
Wednesday
the break
Keyword
The break
keyword is used with a switch
statement to skip the remaining cases of the switch
body after a given case
is met.
In the above example, when the switch
statement is evaluated and meets the criteria in case 3
, it skips the remaining code block of the switch
body due to the break;
statement.
the default
Keyword
The default
keyword is used with the switch
statement to execute a specified block of code when none of the cases is met in the given switch
statement.
Let’s look at the following example, which demonstrates using the default
keyword in the switch
statement.
Example Code:
#include <iostream>
using namespace std;
int main() {
int a = 4;
switch (a) {
case 6:
cout << "Value of a is 6";
break;
case 7:
cout << "Value of a is 7";
break;
default:
cout << "The number is other than 6 or 7";
}
}
Output:
The number is other than 6 or 7
In this example, we have specified the value of the integer variable as 4, but none of the cases will satisfy the given condition during the execution. Therefore, the default
block is executed.
Fix the Jump to case label
Error in the switch
Statement in C++
A common error that may arise while using the switch
statement is a Jump to case label
error. The error occurs when a declaration is made within/under some case
label.
Let’s look at the following example to understand the issue:
#include <iostream>
using namespace std;
int main() {
int a = 1;
switch (a) {
case 1:
int i = 66;
cout << i;
break;
case 2:
cout << i * 3;
break;
default:
cout << "Looking forward to the Weekend";
}
return 0;
}
In the above example, when we initialize i=66
in case 1:
and execute the code. The code generates an error Jump to case label
as the value of i
is visible to the other cases.
A case
is just a label and therefore doesn’t restrict the scope of the code written next to it. Hence, if case 2
is executed during execution, i
will be an uninitialized variable.
So, a strongly typed language like C++ will never allow this to happen. Thus, it generates a compile-time error.
The scope delimiters {}
within case 1
can overcome this scope issue and help execute the code without error.
#include <iostream>
using namespace std;
int main() {
int a = 1;
switch (a) {
case 1: {
int i = 66;
cout << i;
break;
}
case 2:
cout << "value does not exist";
break;
default:
cout << "Looking forward to the Weekend";
}
return 0;
}