C++ std::bad_alloc Exception
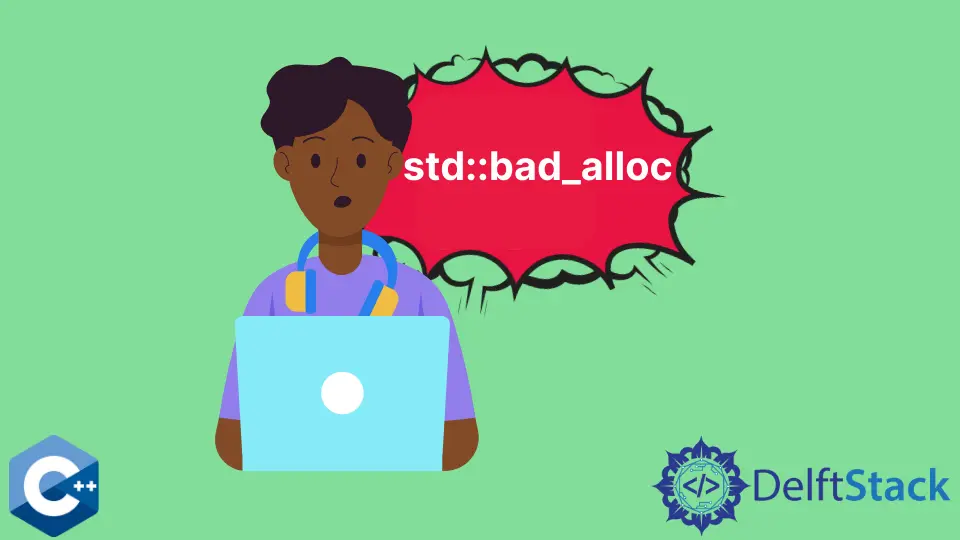
This short article discusses the main cause of the std::bad_alloc()
exception in C++. It also discusses the method to smoothly tackle this run-time exception using C++ exception handling.
Exception Handling in C++
All exceptions derive from the std::exception
class. It’s a run-time error that can be fixed.
If we don’t deal with the exception, the program will print a message of failure and terminate. The execution control can be transferred from one part of a program to another - thanks to exceptions.
C++ uses the three keywords try
,catch
, and throw
to deal with exceptions.
Keyword | Description |
---|---|
throw |
We throw an exception using the throw keyword and define the sort of exception that may arise if there is a portion of your code that has the potential to cause a problem. |
try |
The code segment that can create an error and has to throw an exception is contained in the try block. |
catch |
The block called when an exception is thrown is known as the catch block. If necessary, we can stop the application programmatically and notify the user of the mistake in this block of code. |
the std::bad_alloc
Exception in C++
Whenever we try to create an object using a new
keyword, the compiler requests the memory on the heap. If the required amount of memory is unavailable on the heap, an exception is thrown, known as the std::bad_alloc
exception.
If you declare a single object using the new
keyword or a dynamic array, an exception will be thrown at run-time if the required memory is unavailable.
Let’s look at the example below to handle the bad_alloc
exception.
Example Code:
#include <iostream>
#include <new>
int main() {
try {
int* newarr = new int[2000000000];
} catch (std::bad_alloc& all) {
std::cerr << "bad_alloc exception occurred: " << all.what() << '\n';
}
return 0;
}
Output:
bad_alloc exception occurred: std::bad_alloc
If we do not enclose this code in a try-catch
block, this will probably crash down our program. To avoid such situations, we should always handle this exception so that the program can be terminated normally by informing the user about the error.