宣言は C++ のパラメーター エラーを隠します
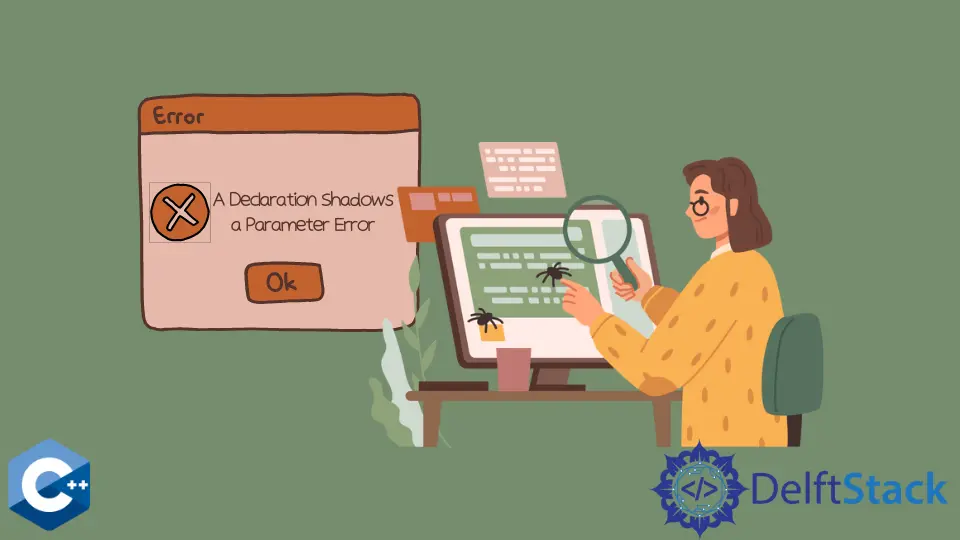
public
、private
、または protected
として C++ のアクセス指定子によって定義されたデータ メンバーまたはメンバー関数のような他のクラス メンバーにアクセスするために、すべてのオブジェクトまたは変数には常にいくつかの境界、範囲、またはスコープがあります。
プログラムの特定のスコープまたはブロックで同じ名前の変数を複数回定義すると、コンパイラは混乱し、宣言がパラメーターをシャドウする
というエラーをスローします。
C++ のアクセス指定子
シャドウ パラメーターの詳細に入る前に、アクセス指定子を理解することが重要です。 通常、3つのアクセス指定子は public
、private
、および protected
です。
C++ のパブリック アクセス指定子
Public
指定子は、クラスのすべてのメンバー (データ メンバーまたはメンバー関数) をパブリックにします。これは、プログラム全体で他のクラスからアクセスできます。 ドット .
を使用して、これらのメンバー関数に直接アクセスできます。 同じクラスのオブジェクトを持つ演算子。
#include <iostream>
using namespace std;
// class definition
class Cal_Circle {
public: // Public access specifier
double radius;
double compute_area() { return 3.14 * radius * radius; }
};
// main function
int main() {
Cal_Circle obj;
// accessing public datamember outside class
obj.radius = 3.4;
cout << "Radius of the circle is: " << obj.radius << "\n";
cout << "Area of the circle is: " << obj.compute_area();
return 0;
}
出力:
Radius of the circle is: 3.4
Area of the circle is: 36.2984
C++ のプライベート アクセス指定子
プライベート
アクセス指定子は、データ メンバーまたはメンバー関数のいずれかのすべてのメンバーを、クラスの境界内でプライベートに保ちます。 クラスのオブジェクトから直接クラス外にアクセスすることはできません。
これらは、プログラムのセキュリティを確保するために、クラス外のアクセスから隠されています。
#include <iostream>
using namespace std;
// class definition
class Cal_Circle {
private: // private access specifier
double radius;
double compute_area() { return 3.14 * radius * radius; }
};
// main function
int main() {
Cal_Circle obj;
// accessing public datamember outside class
obj.radius = 3.4;
cout << "Radius of the circle is: " << obj.radius << "\n";
cout << "Area of the circle is: " << obj.compute_area();
return 0;
}
出力:
error: 'double Cal_Circle::radius' is private within this context
obj.radius = 3.4;
note: declared private here
double radius;
note: declared private here
double compute_area(){
この例では、accessed 指定子を private
として使用し、main 関数からクラスのオブジェクトを介してアクセスしています。 そして、ここで保護されていると宣言されているクラス メンバーごとにエラーをスローしています。
C++ の保護されたアクセス指定子
同様に、Protected
アクセス指定子は、そのクラスのオブジェクトを介して直接そのクラスの外部にアクセスすることはできませんが、違いは Protected
クラスまたはメンバーに派生クラスを介してアクセスできることです。
派生クラスのオブジェクトを使用して、クラスの保護されたメンバーにアクセスできます。
#include <iostream>
using namespace std;
class Radius { // Base class
protected: // protected access specifier
double radius;
};
class Cal_Circle : public Radius { // Derive class
public: // private access specifier
double compute_area(int a) {
radius = a;
return 3.14 * radius * radius;
}
};
// main function
int main() {
Cal_Circle obj;
// accessing public datamember outside class
cout << "Area of the circle is: " << obj.compute_area(3);
return 0;
}
出力:
Area of the circle is: 28.26
基本クラスは保護されており、1つのデータ メンバー radius
があります。 その基本クラスを Cal_Circle
に継承し、派生クラスのオブジェクトを使用して、保護されたデータ メンバー radius
にアクセスしています。
保護されたクラスの保護されたメンバーに間接的にアクセスしています。
C++ での宣言がパラメーターをシャドウする
エラー
コンピューター プログラミングでは、スコープと呼ばれる特定の境界があります。 これは、if-else
ブロック、関数、またはクラスである可能性があります。
if-else
ブロック、関数、またはクラス内で定義された変数は、public
として定義しない限り使用できません。 また、パブリック変数、関数、またはクラスは、プログラム全体でアクセスできます。
また、C++ では、特定のスコープ内で 1つの変数を 2 回以上定義することは禁止されています。 そして、それを行うと、宣言がパラメーターをシャドウする
としてエラーがスローされます。
例を通してそれを理解しましょう。
#include <iostream>
int doublenumber();
using namespace std;
int doublenumber(int x) {
int x; // We are redefining the same variable as the parameter of the
// function
return 2 * x;
cout << endl;
}
int main() { // Main function
int a;
cout << "Enter the number that you want to double it : " << endl;
cin >> a;
int d = doublenumber(a);
cout << "Double : " << d << endl;
return 0;
}
出力:
Test.cpp: In function 'int doublenumber(int)':
Test.cpp:6:9: error: declaration of 'int x' shadows a parameter
int x;
これまで見てきたように、関数、クラス、if-else
ブロックなどの特定のコード ブロックで、1つの変数を複数回定義することはできません。
doublenumber()
関数には、パラメーター int x
があり、この関数のスコープでは、パラメーターをシャドウしてエラーをスローする別のローカル int x
も定義しています。
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn