선언은 C++에서 매개변수 오류를 숨깁니다.
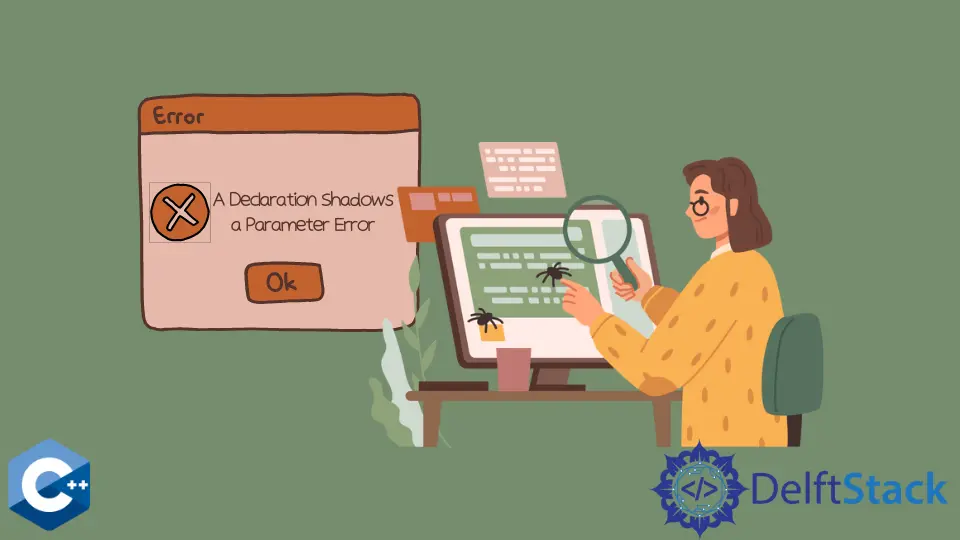
C++의 액세스 지정자에 의해 public
, private
또는 protected
로 정의된 멤버 함수 또는 데이터 멤버와 같은 다른 클래스 멤버에 액세스하기 위한 모든 개체 또는 변수의 일부 경계, 범위 또는 범위가 항상 있습니다.
프로그램의 특정 범위 또는 블록에서 동일한 이름을 가진 변수를 두 번 이상 정의하면 컴파일러가 혼동을 일으키고 선언이 매개변수를 숨긴다
는 오류가 발생합니다.
C++의 액세스 지정자
섀도우 매개변수에 대해 자세히 알아보기 전에 액세스 지정자를 이해하는 것이 중요합니다. 일반적으로 세 가지 액세스 지정자는 public
, private
및 protected
입니다.
C++의 공개 액세스 지정자
Public
지정자는 클래스의 모든 멤버(데이터 멤버 또는 멤버 함수)를 공용으로 만들어 다른 클래스에서 프로그램 전체에 액세스할 수 있습니다. 점 .
을 사용하여 해당 멤버 함수에 직접 액세스할 수 있습니다. 동일한 클래스의 개체가 있는 연산자입니다.
#include <iostream>
using namespace std;
// class definition
class Cal_Circle {
public: // Public access specifier
double radius;
double compute_area() { return 3.14 * radius * radius; }
};
// main function
int main() {
Cal_Circle obj;
// accessing public datamember outside class
obj.radius = 3.4;
cout << "Radius of the circle is: " << obj.radius << "\n";
cout << "Area of the circle is: " << obj.compute_area();
return 0;
}
출력:
Radius of the circle is: 3.4
Area of the circle is: 36.2984
C++의 개인 액세스 지정자
‘Private’ 액세스 지정자는 클래스 경계 내에서 모든 멤버(데이터 멤버 또는 멤버 함수)를 비공개로 유지합니다. 해당 클래스의 개체가 클래스 외부에서 직접 액세스할 수 없습니다.
그들은 프로그램의 보안을 보장하기 위해 클래스 외부의 액세스로부터 숨겨집니다.
#include <iostream>
using namespace std;
// class definition
class Cal_Circle {
private: // private access specifier
double radius;
double compute_area() { return 3.14 * radius * radius; }
};
// main function
int main() {
Cal_Circle obj;
// accessing public datamember outside class
obj.radius = 3.4;
cout << "Radius of the circle is: " << obj.radius << "\n";
cout << "Area of the circle is: " << obj.compute_area();
return 0;
}
출력:
error: 'double Cal_Circle::radius' is private within this context
obj.radius = 3.4;
note: declared private here
double radius;
note: declared private here
double compute_area(){
이 예제에서 액세스 지정자를 private
로 사용하고 기본 함수에서 클래스의 개체를 통해 액세스했습니다. 그리고 여기에서 보호한다고 선언된 각 클래스 구성원에 대해 오류를 발생시킵니다.
C++의 보호 액세스 지정자
마찬가지로 Protected
액세스 지정자는 해당 클래스의 개체를 통해 해당 클래스 외부에서 직접 액세스할 수 없지만 차이점은 Protected
클래스 또는 멤버는 파생 클래스를 통해 액세스할 수 있다는 것입니다.
파생 클래스의 개체를 사용하여 클래스의 보호된 멤버에 액세스할 수 있습니다.
#include <iostream>
using namespace std;
class Radius { // Base class
protected: // protected access specifier
double radius;
};
class Cal_Circle : public Radius { // Derive class
public: // private access specifier
double compute_area(int a) {
radius = a;
return 3.14 * radius * radius;
}
};
// main function
int main() {
Cal_Circle obj;
// accessing public datamember outside class
cout << "Area of the circle is: " << obj.compute_area(3);
return 0;
}
출력:
Area of the circle is: 28.26
기본 클래스는 보호되고 하나의 데이터 멤버인 radius
를 가집니다. 기본 클래스를 Cal_Circle
에 상속했으며 파생 클래스의 개체를 사용하여 보호된 데이터 멤버 radius
에 액세스합니다.
우리는 보호된 클래스의 보호된 구성원에 간접적으로 액세스하고 있습니다.
C++의 Declaration Shadows a Parameter
오류
컴퓨터 프로그래밍에는 범위라고 하는 특정 경계가 있습니다. 이것은 if-else
블록, 함수 또는 클래스일 수 있습니다.
if-else
블록, 함수 또는 클래스 내부에 정의된 변수는 public
으로 정의하지 않는 한 사용할 수 없습니다. 그리고 공용 변수, 함수 또는 클래스는 프로그램 전체에서 액세스할 수 있습니다.
또한 C++에서는 특정 범위 내에서 하나의 변수를 두 번 이상 정의하는 것을 금지합니다. 그리고 이렇게 하면 선언이 매개 변수를 숨김
으로 오류가 발생합니다.
예를 통해 이해해 봅시다.
#include <iostream>
int doublenumber();
using namespace std;
int doublenumber(int x) {
int x; // We are redefining the same variable as the parameter of the
// function
return 2 * x;
cout << endl;
}
int main() { // Main function
int a;
cout << "Enter the number that you want to double it : " << endl;
cin >> a;
int d = doublenumber(a);
cout << "Double : " << d << endl;
return 0;
}
출력:
Test.cpp: In function 'int doublenumber(int)':
Test.cpp:6:9: error: declaration of 'int x' shadows a parameter
int x;
우리가 본 것처럼 함수, 클래스 및 if-else
블록과 같은 특정 코드 블록에서 하나의 변수를 두 번 이상 정의할 수 없습니다.
doublenumber()
함수에는 int x
매개변수가 있고 이 함수의 범위에서 매개변수를 가리고 오류를 발생시키는 또 다른 로컬 int x
도 정의합니다.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn