How to Check if String Is Empty in C++
-
Use Built-In Method
empty()
to Check if String Is Empty in C++ -
Use Custom Defined Function With
size
to Check if String Is Empty in C++ -
Use the
strlen()
Function to Check if String Is Empty in C++
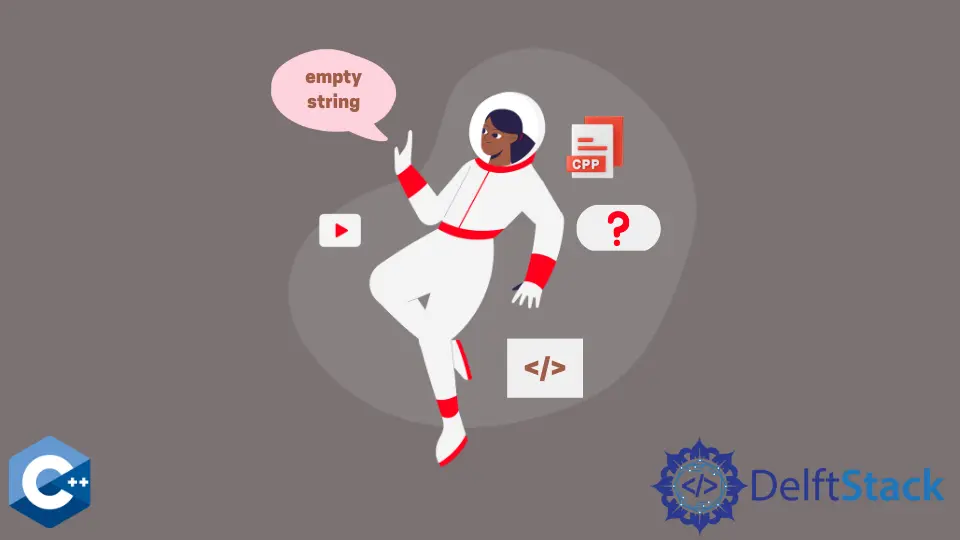
This article will introduce multiple methods about how to check for an empty string in C++.
Use Built-In Method empty()
to Check if String Is Empty in C++
The std::string
class has a built-in method empty()
to check if the given string is empty or not. This method is of type bool
and returns true
when the object does not contain characters. The empty()
method does not take any argument and implements a constant time complexity function.
Note that even if the string
is initialized with an empty string literal - ""
, the function still returns the true
value. Thus, the empty()
function checks for the size of the string and essentially returns the evaluation of the expression - string.size() == 0
.
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string string1("This is a non-empty string");
string string2;
string1.empty() ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
string2.empty() ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
Output:
string value: This is a non-empty string
[ERROR] string is empty!
Use Custom Defined Function With size
to Check if String Is Empty in C++
The previous method could be implemented by the user-defined function that takes a single string
argument and checks if it is empty. This function would mirror the behavior of the empty
method and return a bool
value. The following example demonstrates the same code example with the custom-defined function checkEmptyString
. Notice that the function contains only one statement as the return type of the comparison expression would be boolean, and we can directly pass that to the return
keyword.
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool checkEmptyString(const string &s) { return s.size() == 0; }
int main() {
string string1("This is a non-empty string");
string string2;
checkEmptyString(string1) ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
checkEmptyString(string2) ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
Output:
string value: This is a non-empty string
[ERROR] string is empty!
Use the strlen()
Function to Check if String Is Empty in C++
The strlen()
function is part of the C string library and can be utilized to retrieve the string’s size in bytes. This method could be more flexible for both string
and char*
type strings that may come up in the codebase. strlen
takes const char*
argument and calculates the length excluding the terminating \0
character.
Note that the program’s structure is similar to the previous methods, as we define a separate boolean function and declare the only parameter it takes as const char*
.
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool checkEmptyString(const char *s) { return strlen(s) == 0; }
int main() {
string string1("This is a non-empty string");
string string2;
checkEmptyString(string1.data())
? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
checkEmptyString(string2.data())
? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
Output:
string value: This is a non-empty string
[ERROR] string is empty!
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++