在 C++ 中检查字符串是否为空
Jinku Hu
2023年10月12日
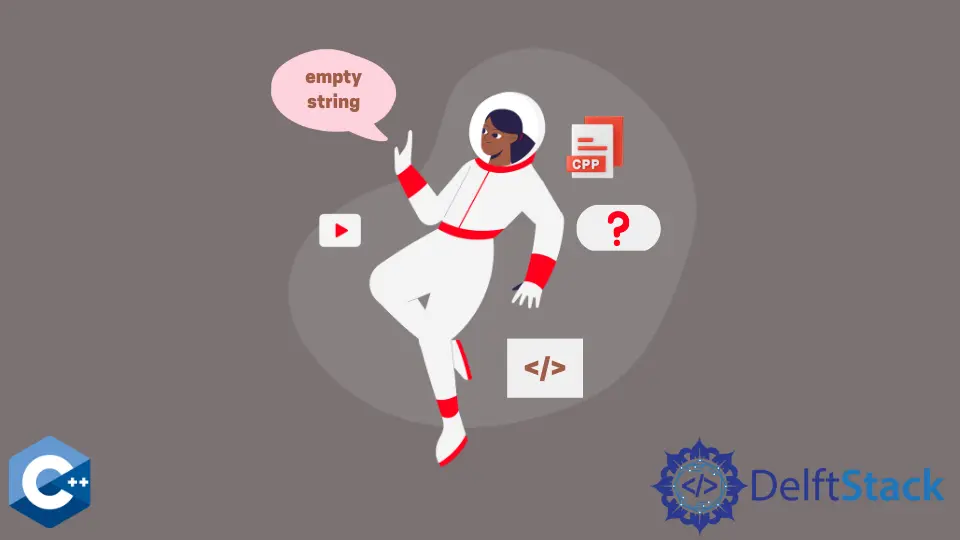
本文将介绍关于如何在 C++ 中检查空字符串的多种方法。
使用内置方法 empty()
来检查 C++ 中的字符串是否为空
std::string
类有一个内置的方法 empty()
来检查给定的字符串是否为空。这个方法的类型是 bool
,当对象不包含字符时返回 true
。empty()
方法不接受任何参数,并实现了一个恒时复杂度函数。
请注意,即使字符串被初始化为空字符串字面–""
,该函数仍然返回 true
值。因此,empty()
函数检查字符串的大小,并本质上返回表达式的评估值-string.size() == 0
。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string string1("This is a non-empty string");
string string2;
string1.empty() ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
string2.empty() ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
输出:
string value: This is a non-empty string
[ERROR] string is empty!
在 C++ 中使用自定义定义的 size
函数检查字符串是否为空
前一种方法可以由用户定义的函数来实现,该函数接受一个 string
参数并检查其是否为空。这个函数将反映 empty
方法的行为,并返回一个 bool
值。下面的例子演示了使用自定义函数 checkEmptyString
的相同代码示例。请注意,该函数只包含一条语句,因为比较表达式的返回类型将是布尔值,我们可以直接将其传递给 return
关键字。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool checkEmptyString(const string &s) { return s.size() == 0; }
int main() {
string string1("This is a non-empty string");
string string2;
checkEmptyString(string1) ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
checkEmptyString(string2) ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
输出:
string value: This is a non-empty string
[ERROR] string is empty!
使用 strlen()
函数检查 C++ 中的字符串是否为空
strlen()
函数是 C 字符串库的一部分,可以用来检索字符串的字节大小。对于代码库中可能出现的 string
和 char*
类型的字符串,这种方法可以更加灵活。strlen
接受 const char*
参数,并计算出不包括终止符 \0
的长度。
请注意,该程序的结构与前面的方法类似,因为我们定义了一个单独的布尔函数,并将其唯一的参数声明为 const char*
。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool checkEmptyString(const char *s) { return strlen(s) == 0; }
int main() {
string string1("This is a non-empty string");
string string2;
checkEmptyString(string1.data())
? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
checkEmptyString(string2.data())
? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
输出:
string value: This is a non-empty string
[ERROR] string is empty!
作者: Jinku Hu