C++에서 문자열이 비어 있는지 확인
-
내장 메서드
empty()
를 사용하여 C++에서 문자열이 비어 있는지 확인 -
size
와 함께 사용자 정의 함수를 사용하여 C++에서 문자열이 비어 있는지 확인 -
strlen()
함수를 사용하여 C++에서 문자열이 비어 있는지 확인
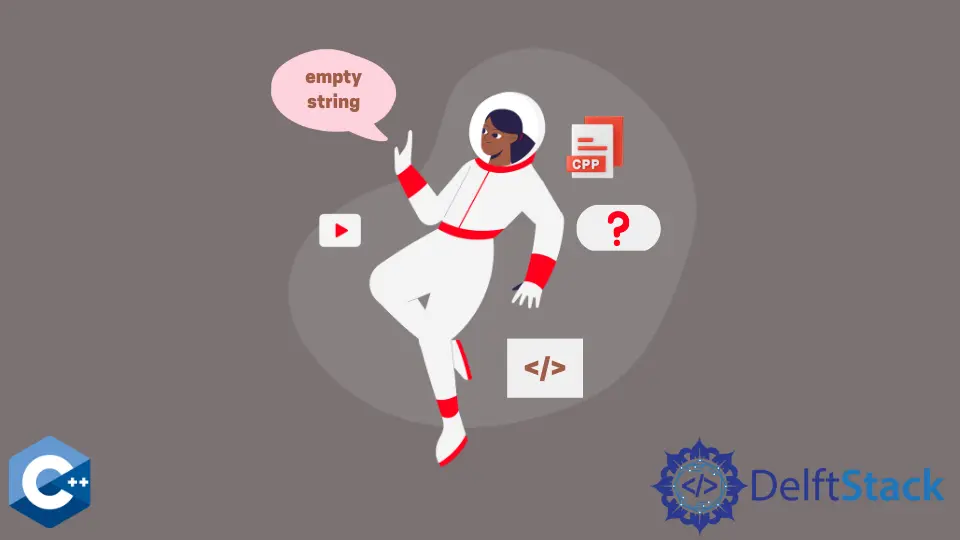
이 기사에서는 C++에서 빈 문자열을 확인하는 방법에 대한 여러 방법을 소개합니다.
내장 메서드empty()
를 사용하여 C++에서 문자열이 비어 있는지 확인
std::string
클래스에는 주어진 문자열이 비어 있는지 여부를 확인하는 내장 메소드empty()
가 있습니다. 이 메소드는 bool
유형이며 개체에 문자가 포함되지 않은 경우 true
를 반환합니다. empty()
메소드는 인수를 취하지 않으며 일정한 시간 복잡도 함수를 구현합니다.
string
이 빈 문자열 리터럴""
로 초기화 되더라도 함수는 여전히true
값을 반환합니다. 따라서empty()
함수는 문자열의 크기를 확인하고 기본적으로string.size() == 0
표현식의 평가를 반환합니다.
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string string1("This is a non-empty string");
string string2;
string1.empty() ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
string2.empty() ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
출력:
string value: This is a non-empty string
[ERROR] string is empty!
size
와 함께 사용자 정의 함수를 사용하여 C++에서 문자열이 비어 있는지 확인
이전 메소드는 단일 문자열인수를 취하고 비어 있는지 확인하는 사용자 정의 함수로 구현할 수 있습니다. 이 함수는empty
메소드의 동작을 미러링하고bool
값을 반환합니다. 다음 예제는 사용자 정의 함수checkEmptyString
이있는 동일한 코드 예제를 보여줍니다. 함수에는 비교 표현식의 반환 유형이 부울이므로 하나의 문만 포함되어 있으며이를 return
키워드에 직접 전달할 수 있습니다.
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool checkEmptyString(const string &s) { return s.size() == 0; }
int main() {
string string1("This is a non-empty string");
string string2;
checkEmptyString(string1) ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
checkEmptyString(string2) ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
출력:
string value: This is a non-empty string
[ERROR] string is empty!
strlen()
함수를 사용하여 C++에서 문자열이 비어 있는지 확인
strlen()
함수는 C 문자열 라이브러리의 일부이며 문자열의 크기를 바이트 단위로 검색하는 데 사용할 수 있습니다. 이 메서드는 코드베이스에 나타날 수있는string
및char *
유형 문자열 모두에 대해 더 유연 할 수 있습니다. strlen
은const char *
인수를 취하고 종료\0
문자를 제외한 길이를 계산합니다.
프로그램의 구조는 별도의 부울 함수를 정의하고 const char *
로 사용하는 유일한 매개 변수를 선언하기 때문에 이전 메소드와 유사합니다.
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool checkEmptyString(const char *s) { return strlen(s) == 0; }
int main() {
string string1("This is a non-empty string");
string string2;
checkEmptyString(string1.data())
? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
checkEmptyString(string2.data())
? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
출력:
string value: This is a non-empty string
[ERROR] string is empty!
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook