C++ で文字列が空かどうかをチェックする
-
C++ で文字列が空かどうかをチェックするには組み込みのメソッド
empty()
を使用する -
C++ で文字列が空かどうかをチェックするには
size
を持つカスタム定義関数を使用する -
C++ で文字列が空かどうかをチェックするには
strlen()
関数を使用する
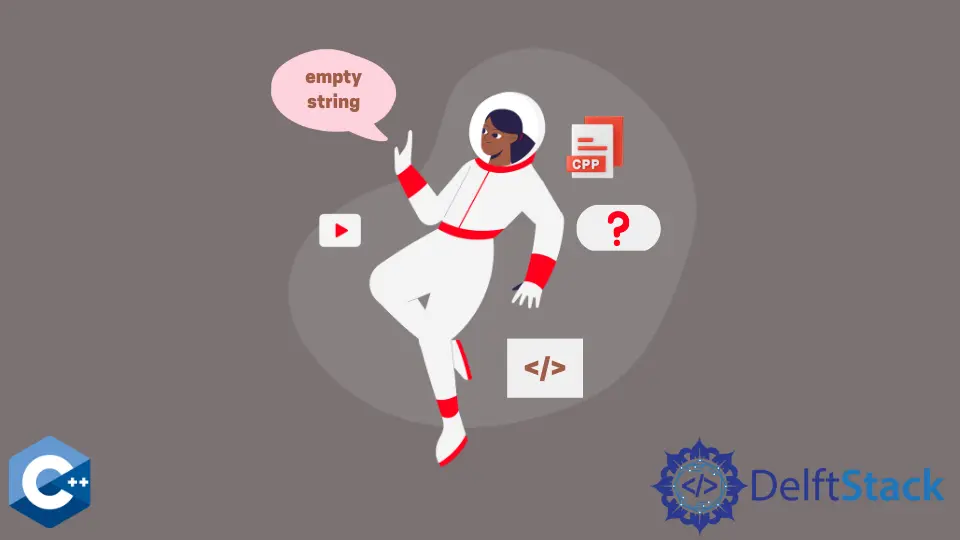
この記事では、C++ で文字列が空かどうかをチェックする方法について複数のメソッドを紹介します。
C++ で文字列が空かどうかをチェックするには組み込みのメソッド empty()
を使用する
std::string
クラスには、与えられた文字列が空かどうかをチェックするためのメソッド empty()
が組み込まれています。このメソッドは bool
型で、オブジェクトに文字が含まれていない場合は true
を返します。empty()
メソッドは引数を取らず、一定の時間複雑度関数を実装しています。
また、string
が空の文字列リテラル - ""
で初期化されていても、この関数は true
を返すことに注意してください。このように、関数 empty()
は文字列のサイズを調べ、本質的には式 - string.size() == 0
の評価を返します。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string string1("This is a non-empty string");
string string2;
string1.empty() ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
string2.empty() ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
出力:
string value: This is a non-empty string
[ERROR] string is empty!
C++ で文字列が空かどうかをチェックするには size
を持つカスタム定義関数を使用する
前のメソッドは、引数 string
を 1つ受け取り、それが空かどうかをチェックするユーザ定義の関数で実装することができます。この関数は empty
メソッドの動作を反映し、bool
値を返すことになります。以下の例は、カスタム定義の関数 checkEmptyString
を用いて同じコード例を示しています。比較式の戻り値の型はブール値であり、これを return
キーワードに直接渡すことができるので、この関数には 1つの文しか含まれていないことに注意してください。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool checkEmptyString(const string &s) { return s.size() == 0; }
int main() {
string string1("This is a non-empty string");
string string2;
checkEmptyString(string1) ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
checkEmptyString(string2) ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
出力:
string value: This is a non-empty string
[ERROR] string is empty!
C++ で文字列が空かどうかをチェックするには strlen()
関数を使用する
関数 strlen()
は C 言語の文字列ライブラリの一部であり、文字列のサイズをバイト単位で取得するために利用することができます。このメソッドは、コードベースに登場する可能性のある string
と char*
型の文字列に対して、より柔軟に対応することができます。strlen
は引数 const char*
を受け取り、終端の \0
文字を除いた長さを計算します。
プログラムの構造は前のメソッドと似ていることに注意してください。別のブール関数を定義し、それが取る唯一のパラメーターを const char*
として宣言しているからです。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool checkEmptyString(const char *s) { return strlen(s) == 0; }
int main() {
string string1("This is a non-empty string");
string string2;
checkEmptyString(string1.data())
? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
checkEmptyString(string2.data())
? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
出力:
string value: This is a non-empty string
[ERROR] string is empty!