How to Create Array of Structs in C
- How to Create an Array of Structs in C Using Static Array Initialization
-
How to Create an Array of Structs in C Using the
malloc()
Function - Conclusion
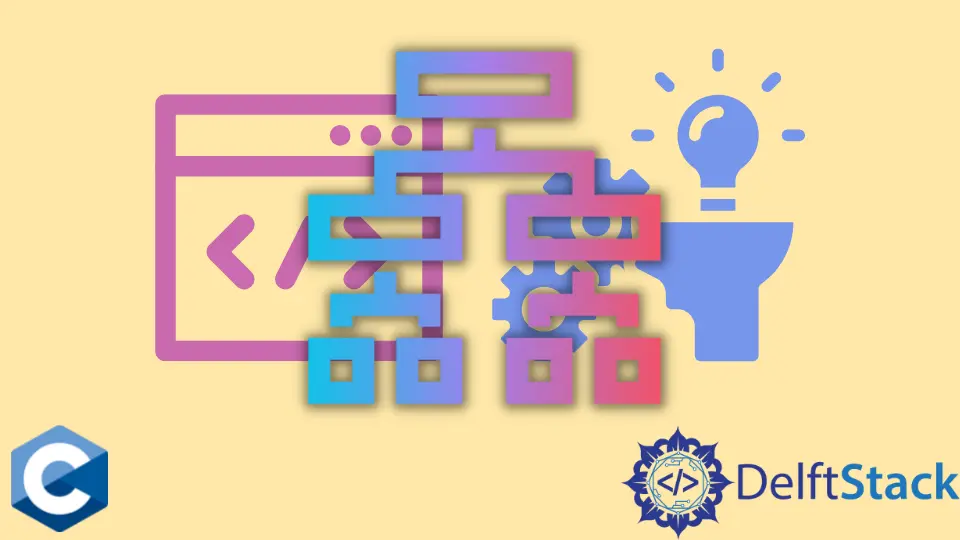
Creating arrays of structures in C is a fundamental aspect of programming, providing a powerful mechanism for organizing and managing complex data.
In this article, we explore various methods to achieve this task, catering to different needs and scenarios.
Whether opting for the simplicity of static array initialization or the flexibility of dynamic memory allocation with the malloc()
function, each approach brings its own advantages to the table.
Understanding these methods equips programmers with the knowledge to handle data efficiently and maintain code clarity in C applications.
How to Create an Array of Structs in C Using Static Array Initialization
Before diving into the array aspect, let’s review the basics of structs. A struct is a composite data type that groups variables of different data types under a single name.
This allows you to organize related information into a cohesive unit. Let’s consider a simple example using a structure named Student
with integer, character array, and float members:
struct Student {
int rollNumber;
char studentName[10];
float percentage;
};
An array of structures in C is a data structure that allows you to store multiple instances of a structure in a contiguous block of memory. Each element of the array is a structure, and each structure contains multiple fields (or members).
This arrangement enables you to organize and manage related data in a more structured and efficient manner.
To create an array of structs, you can use static array initialization. This involves declaring an array of structs and initializing its elements at the time of declaration.
Let’s use the Student
struct from the previous example:
struct Student studentRecord[5] = {{1, "John", 78.5},
{2, "Alice", 89.2},
{3, "Bob", 76.8},
{4, "Eva", 91.7},
{5, "Mike", 85.0}};
Here, the studentRecord
is an array of 5 elements where each element is of type struct Student
. The individual elements are accessed using the index notation ([]
), and members are accessed using the dot .
operator.
The studentRecord[0]
points to the 0th
element of the array, and the studentRecord[1]
points to the first element of the array.
Similarly,
- The
studentRecord[0].rollNumber
refers to therollNumber
member of the 0th element of the array. - The
studentRecord[0].studentName
refers to thestudentName
member of the 0th element of the array. - The
studentRecord[0].percentage
refers to thepercentage
member of the 0th element of the array.
The complete program to declare an array of the struct
in C is as follows.
#include <stdio.h>
// Define the structure
struct Student {
int rollNumber;
char studentName[20];
float percentage;
};
int main() {
// Declare and initialize an array of structs
struct Student studentRecord[5] = {{1, "John", 78.5},
{2, "Alice", 89.2},
{3, "Bob", 76.8},
{4, "Eva", 91.7},
{5, "Mike", 85.0}};
// Display student information
printf("Student Information:\n");
for (int i = 0; i < 5; ++i) {
printf("Roll Number: %d\tName: %s\tPercentage: %.2f\n",
studentRecord[i].rollNumber, studentRecord[i].studentName,
studentRecord[i].percentage);
}
return 0;
}
In the C program above, we create an array of structs to represent student records.
The code begins by including the standard input/output library (stdio.h
). Next, a structure named Student
is defined, containing three members: rollNumber
(integer), studentName
(character array of size 20), and percentage
(float).
Inside the main()
function, an array of structs named studentRecord
is declared and initialized with five elements. Each element corresponds to a student record and includes the student’s roll number, name, and percentage.
This initialization is done using curly braces and providing values for each member of the struct.
struct Student studentRecord[5] = {{1, "John", 78.5},
{2, "Alice", 89.2},
{3, "Bob", 76.8},
{4, "Eva", 91.7},
{5, "Mike", 85.0}};
Subsequently, a for
loop is utilized to iterate through each element of the studentRecord
array. Inside the loop, the printf
function is used to display the student information.
printf("Student Information:\n");
for (int i = 0; i < 5; ++i) {
printf("Roll Number: %d\tName: %s\tPercentage: %.2f\n",
studentRecord[i].rollNumber, studentRecord[i].studentName,
studentRecord[i].percentage);
}
The %d
placeholder is used to print the integer rollNumber
, %s
for the string studentName
, and %.2f
for the floating-point percentage
. The .2f
format specifier ensures that the percentage is displayed with two decimal places.
Finally, the program returns 0, indicating successful execution. The output of the program should be a clear and formatted display of the student information, presenting the roll number, name, and percentage for each student in the array.
Code Output:
This output demonstrates the successful creation and display of an array of structs in C using static array initialization. Each student’s information is presented clearly, showcasing the organization and accessibility of data through the combination of structures and arrays.
How to Create an Array of Structs in C Using the malloc()
Function
While static array initialization is convenient, it may not always provide the flexibility needed in certain scenarios.
Dynamic memory allocation, achieved through the malloc()
function, allows us to create an array of structs with a size determined at runtime. This approach is particularly useful when dealing with variable-sized datasets.
The malloc()
function is used to dynamically allocate memory. Its syntax is as follows:
ptrVariable = (cast - type*)malloc(byte - size)
Here, ptrVariable
is a pointer variable of the specified cast type, and byte-size
is the amount of memory to allocate in bytes. In the case of creating an array of structs, we determine the size by multiplying the number of elements by the size of each struct using sizeof
.
Let’s consider an example program where we create an array of student records using dynamic memory allocation:
#include <stdio.h>
#include <stdlib.h>
// Define the structure
struct Student {
int rollNumber;
char studentName[20];
float percentage;
};
int main() {
int numStudents = 3; // Set the number of students
struct Student *studentRecord;
// Dynamically allocate memory for the array of structs
studentRecord =
(struct Student *)malloc(numStudents * sizeof(struct Student));
// Input student information
printf("Enter Student Information:\n");
for (int i = 0; i < numStudents; ++i) {
printf("Enter Roll Number: ");
scanf("%d", &studentRecord[i].rollNumber);
printf("Enter Name: ");
scanf("%s", studentRecord[i].studentName);
printf("Enter Percentage: ");
scanf("%f", &studentRecord[i].percentage);
}
// Display student information
printf("\nStudent Information:\n");
for (int i = 0; i < numStudents; ++i) {
printf("Roll Number: %d\tName: %s\tPercentage: %.2f\n",
studentRecord[i].rollNumber, studentRecord[i].studentName,
studentRecord[i].percentage);
}
// Free dynamically allocated memory
free(studentRecord);
return 0;
}
In this C program, dynamic memory allocation is utilized to create an array of structs representing student records. The program begins by including the necessary libraries, stdio.h
for standard input/output and stdlib.h
for dynamic memory allocation functions.
A structure named Student
is defined to hold information about each student, including their roll number, name, and percentage. Inside the main()
function, an integer variable numStudents
is declared and set to 3, representing the desired number of students.
Next, a pointer to a struct Student
named studentRecord
is declared.
The malloc()
function is then used to dynamically allocate memory for an array of structs based on the product of numStudents
and sizeof(struct Student)
. The cast (struct Student*)
is applied to the result of malloc()
to ensure that the allocated memory is treated as an array of struct Student
.
A for
loop is employed to iterate through each student in the dynamically allocated array. Inside the loop, the program prompts the user to input information for each student using printf
and scanf
functions.
The entered data is stored in the corresponding members of the studentRecord
array.
After collecting the input, another for
loop is used to display the student information, utilizing printf
to format and print each student’s roll number, name, and percentage. The .2f
format specifier ensures that the percentage is displayed with two decimal places.
Finally, the free()
function is called to release the dynamically allocated memory, preventing memory leaks. The program returns 0, indicating successful execution.
Code Output:
This output demonstrates the dynamic creation of an array of structs using the malloc()
function. Users can input information for the specified number of students, and the program displays the entered data.
The dynamic allocation allows for a variable number of elements and provides more flexibility in memory management.
Conclusion
The ability to create arrays of structs in C is an essential skill for developers working with diverse datasets. The static approach provides straightforward initialization and ease of use, while dynamic memory allocation offers flexibility when dealing with varying data sizes.
The examples provided showcase practical implementations of both methods, highlighting their strengths and use cases. As you delve into struct arrays in C, consider the specific requirements of your program and choose the method that best aligns with your coding objectives.
With these tools at your disposal, you’ll be well-equipped to navigate the complexities of data organization in C programming.
Related Article - C Array
- How to Copy Char Array in C
- How to Dynamically Allocate an Array in C
- How to Clear Char Array in C
- Array of Strings in C
- How to Initialize Char Array in C
- How to Print Char Array in C