Array de Estruturas em C
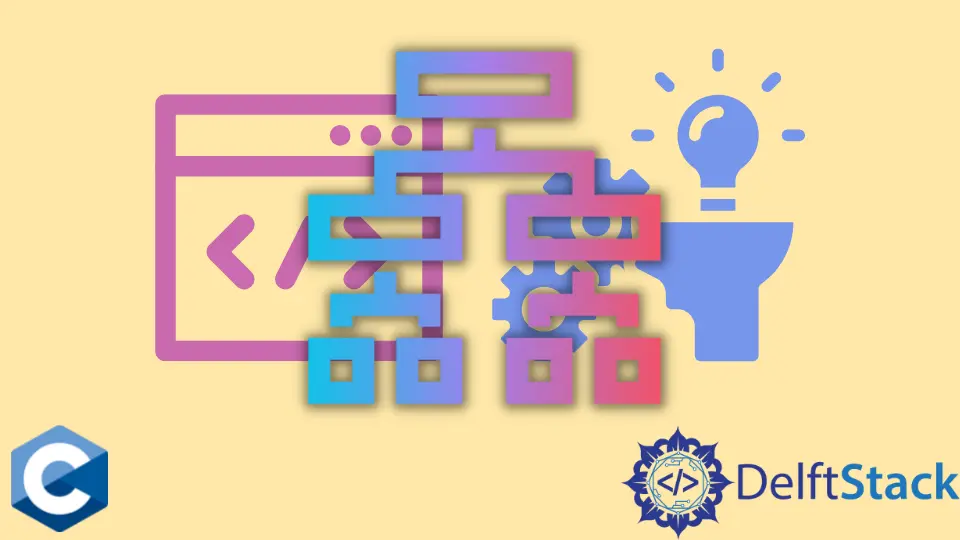
Este tutorial introduz como criar um array de estruturas em C. É a colecção de múltiplas variáveis de estrutura onde cada variável contém informação sobre diferentes entidades.
Array de struct
em C
um array é uma colecção sequencial do mesmo tipo de dados, e uma estrutura é um tipo de dados definido pelo utilizador. A declaração de um array de estruturas é a mesma que um array dos tipos de dados primitivos, mas utiliza a estrutura tem o tipo de dados dos seus elementos.
Considere um exemplo de uma estrutura chamada Student
, como abaixo:
struct Student {
int rollNumber;
char studentName[10];
float percentage;
};
Podemos declarar um array de estruturas como a seguir.
struct Student studentRecord[5];
Aqui, o studentRecord
é um array de 5 elementos em que cada elemento é do tipo struct
Student
. Os elementos individuais são acedidos utilizando a notação de índice ([]
), e os membros são acedidos utilizando o ponto .
operador.
O studentRecord[0]
aponta para o elemento 0th
do array, e o studentRecord[1]
aponta para o primeiro elemento do array.
Da mesma forma,
- O
studentRecord[0].rollNumber
refere-se aorollNumber
membro do 0º elemento do array. - O
studentRecord[0].studentName
refere-se aostudentName
membro do 0º elemento do array. - O
studentRecord[0].percentage
refere-se aopercentagem
membro do 0º elemento do array.
O programa completo para declarar um array do struct
em C é o seguinte.
#include <stdio.h>
#include <string.h>
struct Student {
int rollNumber;
char studentName[10];
float percentage;
};
int main(void) {
int counter;
struct Student studentRecord[5];
printf("Enter Records of 5 students");
for (counter = 0; counter < 5; counter++) {
printf("\nEnter Roll Number:");
scanf("%d", &studentRecord[counter].rollNumber);
printf("\nEnter Name:");
scanf("%s", &studentRecord[counter].studentName);
printf("\nEnter percentage:");
scanf("%f", &studentRecord[counter].percentage);
}
printf("\nStudent Information List:");
for (counter = 0; counter < 5; counter++) {
printf("\nRoll Number:%d\t Name:%s\t Percentage :%f\n",
studentRecord[counter].rollNumber,
studentRecord[counter].studentName,
studentRecord[counter].percentage);
}
return 0;
}
Resultado:
Enter Record of 5 students
Enter Roll number:1
Enter Name: John
Enter percentage: 78
Enter Roll number:2
Enter Name: Nick
Enter percentage: 84
Enter Roll number:3
Enter Name: Jenny
Enter percentage: 56
Enter Roll number:4
Enter Name: Jack
Enter percentage: 77
Enter Roll number:5
Enter Name: Peter
Enter percentage: 76
Student Information List
Roll Number: 1 Name: John percentage:78.000000
Roll Number: 2 Name: Nick percentage:84.000000
Roll Number: 3 Name: Jenny percentage:56.000000
Roll Number: 4 Name: Jack percentage:77.000000
Roll Number: 5 Name: Peter percentage:76.000000
Criar um array do struct
utilizando a malloc()
Função em C
Existe outra forma de fazer um array de struct
em C. A memória pode ser alocada utilizando a função malloc()
para um array de struct
. A isto chama-se alocação dinâmica de memória.
A função malloc()
(alocação de memória) é utilizada para alocar dinamicamente um único bloco de memória com o tamanho especificado. Esta função devolve um ponteiro do tipo void
.
O ponteiro retornado pode ser lançado num ponteiro de qualquer forma. Inicializa cada bloco com o valor de lixo predefinido.
A sintaxe da função malloc()
é a que se segue:
ptrVariable = (cast - type*)malloc(byte - size)
O programa completo para criar um array de funções dinâmicas é o que se segue.
#include <stdio.h>
int main(int argc, char** argv) {
typedef struct {
char* firstName;
char* lastName;
int rollNumber;
} STUDENT;
int numStudents = 2;
int x;
STUDENT* students = malloc(numStudents * sizeof *students);
for (x = 0; x < numStudents; x++) {
students[x].firstName = (char*)malloc(sizeof(char*));
printf("Enter first name :");
scanf("%s", students[x].firstName);
students[x].lastName = (char*)malloc(sizeof(char*));
printf("Enter last name :");
scanf("%s", students[x].lastName);
printf("Enter roll number :");
scanf("%d", &students[x].rollNumber);
}
for (x = 0; x < numStudents; x++)
printf("First Name: %s, Last Name: %s, Roll number: %d\n",
students[x].firstName, students[x].lastName, students[x].rollNumber);
return (0);
}
Resultado:
Enter first name:John
Enter last name: Williams
Enter roll number:1
Enter first name:Jenny
Enter last name: Thomas
Enter roll number:2
First Name: John Last Name: Williams Roll Number:1
First Name: Jenny Last Name: Thomas Roll Number:2
Artigo relacionado - C Array
- Alocar dinamicamente un array em C
- Array de Strings em C
- Clear Char Array em C
- Copiar Char Array em C
- Inicializar Char Array em C