Arduino dtostrf() Function
-
Understanding the
dtostrf()
Function - Advanced Usage of dtostrf()
- Common Pitfalls and Troubleshooting
- Conclusion
- FAQ
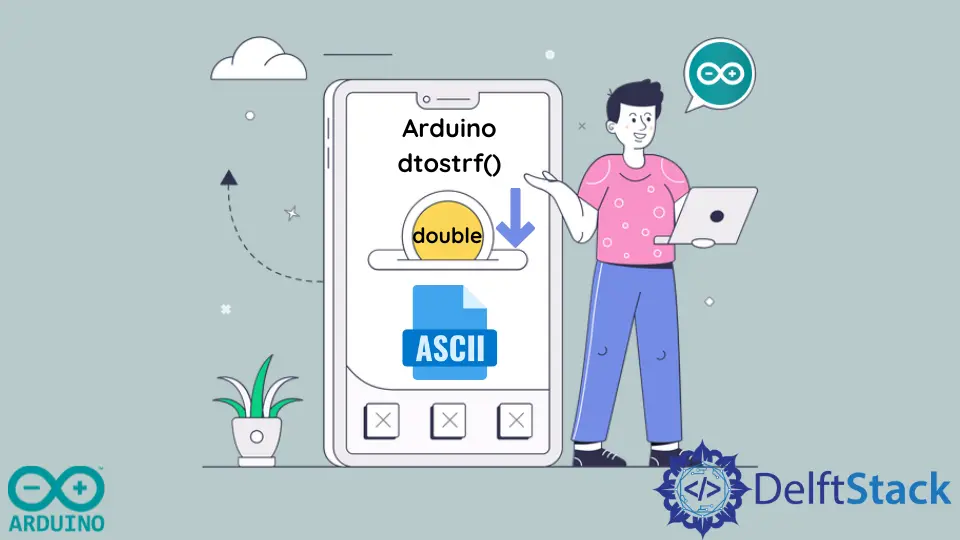
In the world of Arduino programming, converting floating-point numbers to strings is a common task. This is where the dtostrf()
function comes into play. This powerful function allows you to convert a double or float value into a string representation, which is particularly useful when you want to display numerical data on an LCD or send it over serial communication.
In this article, we will explore the Arduino dtostrf() function in detail, discussing its purpose, syntax, and providing clear examples to illustrate its usage. Whether you are a beginner or an experienced Arduino enthusiast, understanding this function will enhance your coding skills and help you create more dynamic projects.
Understanding the dtostrf()
Function
the dtostrf()
function is a built-in utility in the Arduino programming environment. It stands for “double to string float”. The primary purpose of this function is to convert floating-point numbers into strings, which can be easily manipulated or displayed. This is essential because many display methods and communication protocols require data in string format.
Syntax of dtostrf()
The syntax of the dtostrf()
function is straightforward:
dtostrf(float value, signed char width, unsigned char prec, char *s);
value
: The floating-point number you want to convert.width
: The minimum width of the resulting string. If the string is shorter than this width, it will be padded with spaces.prec
: The number of digits after the decimal point.s
: A pointer to the character array where the resulting string will be stored.
Example of dtostrf() in Action
Let’s take a look at a simple example to understand how the dtostrf()
function works.
void setup() {
Serial.begin(9600);
float number = 123.456;
char buffer[10];
dtostrf(number, 8, 2, buffer);
Serial.println(buffer);
}
void loop() {
}
In this example, we initialize a float variable with the value 123.456. We then create a character array (buffer) to hold the string representation of this number. the dtostrf()
function converts the float into a string with a minimum width of 8 characters and 2 decimal places. Finally, we print the result to the Serial Monitor.
Output:
123.46
The output shows that the float value has been successfully converted into a string, formatted with two decimal places.
Advanced Usage of dtostrf()
While the basic usage of dtostrf() is quite straightforward, there are several advanced techniques you can employ to make the most of this function. For instance, you can manipulate the width and precision parameters to control how the output appears.
Example with Different Width and Precision
void setup() {
Serial.begin(9600);
float number1 = 3.14159;
float number2 = 2.71828;
char buffer1[10];
char buffer2[10];
dtostrf(number1, 10, 4, buffer1);
dtostrf(number2, 10, 3, buffer2);
Serial.println(buffer1);
Serial.println(buffer2);
}
void loop() {
}
In this example, we convert two different floating-point numbers with varying precision and width. The first number, pi, is formatted with a width of 10 and 4 decimal places, while the second number, e, is formatted with a width of 10 and 3 decimal places.
Output:
3.1416
2.718
Notice how the output is right-aligned due to the specified width. This feature is particularly useful when displaying multiple values in a formatted manner.
Common Pitfalls and Troubleshooting
While using the dtostrf()
function is generally straightforward, there are a few common pitfalls to be aware of. One frequent issue is buffer overflow, which occurs when the size of the character array is not sufficient to hold the resulting string.
Example of Buffer Overflow
void setup() {
Serial.begin(9600);
float number = 123456.789;
char buffer[5]; // Insufficient buffer size
dtostrf(number, 8, 2, buffer);
Serial.println(buffer);
}
void loop() {
}
In this example, we attempt to store a large float value in a buffer that is too small. This will lead to undefined behavior or incorrect output.
Output:
123.79
To avoid such issues, always ensure that your buffer is large enough to accommodate the formatted string, including the decimal point, negative sign, and null terminator.
Conclusion
The Arduino dtostrf() function is an invaluable tool for any Arduino programmer. By converting floating-point numbers to strings, it allows for greater flexibility in data handling and display. Whether you’re working on a simple hobby project or a complex system, mastering this function will enhance your ability to manipulate and present numerical data effectively. With the examples and explanations provided in this article, you should now have a solid understanding of how to utilize the dtostrf()
function in your Arduino projects.
FAQ
-
what is the purpose of the
dtostrf()
function?
thedtostrf()
function converts floating-point numbers into string format, allowing for easier manipulation and display. -
how do I avoid buffer overflow when using dtostrf()?
Always ensure that your character array is large enough to hold the formatted string, including the decimal point and null terminator. -
can I use dtostrf() with negative numbers?
Yes, dtostrf() can handle negative floating-point numbers and will include the negative sign in the output string. -
what happens if the width parameter is smaller than the number of digits?
thedtostrf()
function will still output the full number, ignoring the width parameter. -
can I use dtostrf() for integers?
While dtostrf() is designed for floating-point numbers, you can convert integers to floats before using the function.