Python os.truncate() Method
- Understanding File Truncation
-
Syntax of Python
os.truncate()
Method -
Example 1: Use the Python
os.truncate()
Method -
Example 2: Respective Length Exceeds the File’s Size in the
os.truncate()
Method in Python -
Example 3: Use the
os.truncate()
Method to Delete a File in Python -
Example 4: Truncate a File Using
truncate()
Method in Python - Practical Use Cases
- Conclusion
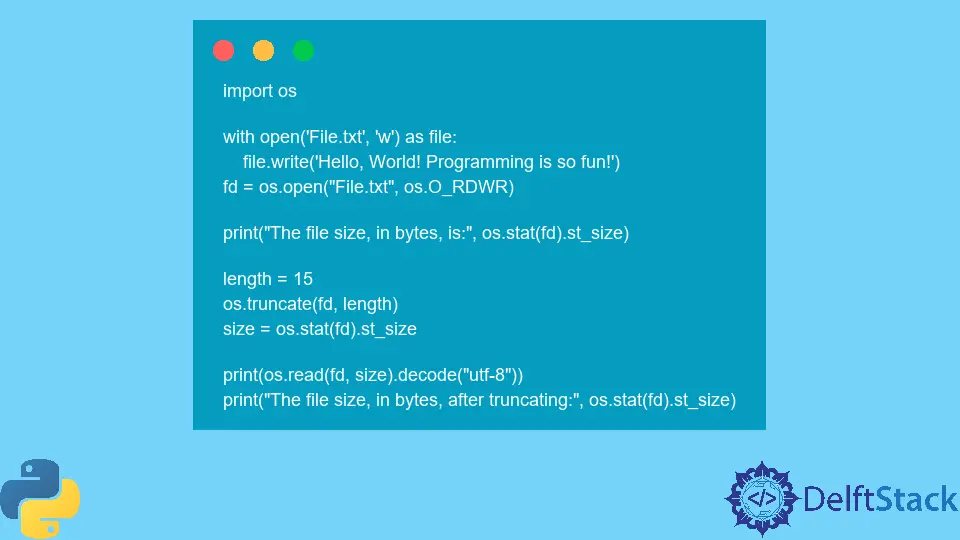
The os.truncate()
method in Python is a powerful tool for file manipulation, allowing developers to resize a file to a specified size. This method is part of the os
module, providing essential functionalities for interacting with the operating system.
Python os.truncate()
method truncates a file indicated by a specific path. A specific length supplied as a parameter causes the file to be truncated.
In this article, we will explore the intricacies of os.truncate()
, understand its syntax and parameters, and delve into practical examples to demonstrate its usage.
Understanding File Truncation
File truncation involves changing the size of a file by either reducing or increasing its length. In the context of os.truncate()
, we focus on reducing the size of a file.
This can be particularly useful when you want to discard the remaining content of a file beyond a certain point or when you need to shrink a file to a specific size.
Syntax of Python os.truncate()
Method
os.truncate(path address, length)
Parameters
path address |
A file system path or a symlink address object. The object can either be an str or bytes. |
length |
An integer value that specifies the number of bytes that the file should be reduced to. |
Return Value
The Python os.truncate()
method does not return any value.
Example 1: Use the Python os.truncate()
Method
This code manipulates a file using the os
module. It writes a string to a file, opens the file using low-level os.open()
, prints its initial size, truncates it to 15 bytes, reads and prints the truncated content, and displays the updated file size after truncation.
The code showcases file operations such as writing, truncating, and reading using os
functions.
import os
with open("File.txt", "w") as file:
file.write("Hello, World! Programming is so fun!")
fd = os.open("File.txt", os.O_RDWR)
print("The file size, in bytes, is:", os.stat(fd).st_size)
length = 15
os.truncate(fd, length)
size = os.stat(fd).st_size
print(os.read(fd, size).decode("utf-8"))
print("The file size, in bytes, after truncating:", os.stat(fd).st_size)
Output:
The file size, in bytes, is: 36
Hello, World! P
The file size, in bytes, after truncating: 15
The os.truncate()
method is equivalent to os.ftruncate()
method in Python 3.3.
Example 2: Respective Length Exceeds the File’s Size in the os.truncate()
Method in Python
This code writes a string to a file, opens the file using low-level os.open()
, prints its initial size, truncates it to 100 bytes, reads and prints the truncated content, and displays the updated file size after truncation.
import os
with open("File.txt", "w") as file:
file.write("Hello, World! Programming is so fun!")
fd = os.open("File.txt", os.O_RDWR)
print("The file size, in bytes, is:", os.stat(fd).st_size)
length = 100
os.truncate(fd, length)
size = os.stat(fd).st_size
print(os.read(fd, size).decode("utf-8"))
print("The file size, in bytes, after truncating:", os.stat(fd).st_size)
Output:
The file size, in bytes, is: 36
Hello, World! Programming is so fun!
The file size, in bytes, after truncating: 100
The File.txt
content did not change up to its original size. The file was filled with random invalid characters to increase the file to the provided length.
Example 3: Use the os.truncate()
Method to Delete a File in Python
This code creates a file, writes a string to it, opens the file using low-level os.open()
, prints its initial size, truncates it to 0 bytes, reads and prints the truncated content (an empty string), and displays the updated file size after truncation (0 bytes).
import os
with open("File.txt", "w") as file:
file.write("Hello, World! Programming is so fun!")
fd = os.open("File.txt", os.O_RDWR)
print("The file size, in bytes, is:", os.stat(fd).st_size)
length = 0
os.truncate(fd, length)
size = os.stat(fd).st_size
print(os.read(fd, size).decode("utf-8"))
print("The file size, in bytes, after truncating:", os.stat(fd).st_size)
Output:
The file size, in bytes, is : 36
The file size, in bytes, after truncating : 0
The os.truncate()
method resizes the file to a specified number of bytes. However, the current position is used if the length is not given as a parameter.
Example 4: Truncate a File Using truncate()
Method in Python
This code showcases file truncation in Python. It creates a file, writes some initial text, gets the original file size, truncates the file to a specified length (10 bytes), and then retrieves and prints the size of the file after truncation.
The code provides insights into how file truncation affects the size of the file.
import os
# Open a file in write mode
with open("example.txt", "w") as file:
file.write("This is a sample text.")
# Get the file size before truncation
original_size = os.path.getsize("example.txt")
print(f"Original file size: {original_size} bytes")
# Truncate the file to 10 bytes
with open("example.txt", "r+") as file:
file.truncate(10)
# Get the file size after truncation
truncated_size = os.path.getsize("example.txt")
print(f"Truncated file size: {truncated_size} bytes")
Output:
Original file size: 22 bytes
Truncated file size: 10 bytes
In this example, a file named "example.txt"
is created with some sample text. The file is then truncated to 10 bytes using os.truncate()
, and the sizes before and after truncation are printed.
Practical Use Cases
- Log Rotation: In applications that generate log files,
os.truncate()
can be used for log rotation. Once a log file reaches a certain size or age, it can be truncated to create a new, empty log file, ensuring efficient log management. - Data Cleanup: When working with files that store temporary or intermediate data,
os.truncate()
provides a convenient way to clean up the file content, removing unnecessary information and preserving only the required data. - Disk Space Management: In scenarios where disk space needs to be reclaimed, especially in applications dealing with large files,
os.truncate()
can be employed to shrink files to a specified size, freeing up disk space. - Partial Data Processing: For applications reading data from files,
os.truncate()
can be used to limit the amount of data processed, allowing developers to focus on specific sections of a file without reading unnecessary content.
Conclusion
The os.truncate()
method is a valuable asset for Python developers engaged in file manipulation tasks. Whether it’s log management, data cleanup, or efficient disk space usage, this method provides a straightforward and efficient means of resizing files.
By understanding its usage and integration into various scenarios, developers can optimize file operations and enhance the overall efficiency of their Python applications.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn