Python os.ftruncate() Method
-
Syntax of Python
os.ftruncate()
Method -
Example Code: Use the
os.ftruncate()
Method -
Example Codes: Use the
os.ftruncate()
Method With Length Exceeding the File Size in Python -
Example Codes: Use the
os.ftruncate()
Method to Empty a File - Conclusion
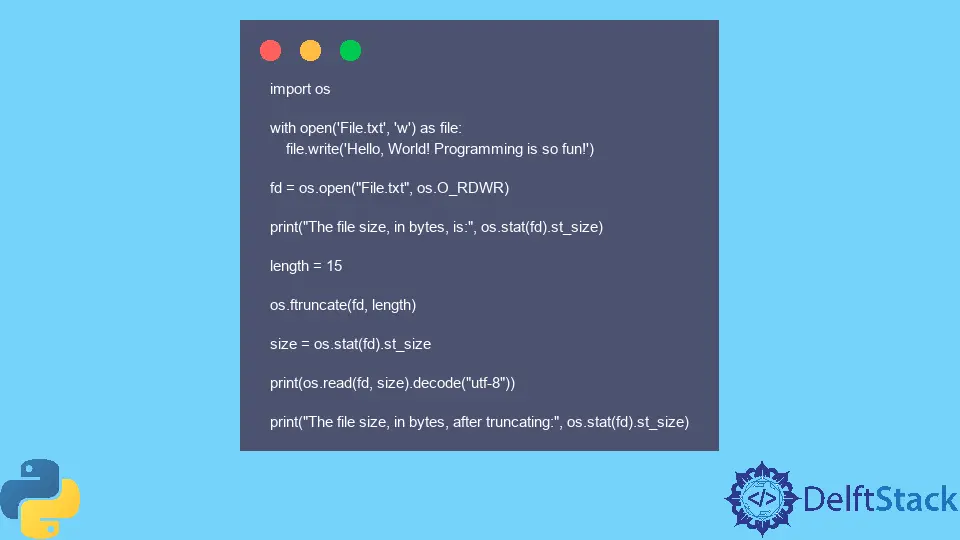
In the realm of file manipulation within Python, the os.ftruncate()
method emerges as a powerful and versatile tool for precisely adjusting the size of files.
This method is designed to truncate a file associated with a given file descriptor, allowing developers to dynamically modify file lengths according to specific requirements.
This article explores the nuances of this method, shedding light on its functionality and applications within the broader context of Python file operations.
Syntax of Python os.ftruncate()
Method
os.ftruncate(file_descriptor, length)
Parameters
Parameters | Type | Explanation |
---|---|---|
file_descriptor |
The file descriptor that must be truncated. | |
length |
Integer | A value representing a length in bytes to which the file is to be reduced. |
Returns
The os.ftruncate
function doesn’t return anything explicitly (returns None
). Instead, it modifies the file’s size in place.
Example Code: Use the os.ftruncate()
Method
The os.ftruncate()
method is equivalent to another Python method os.truncate()
. It’s worth noting that os.ftruncate
works directly with file descriptors, while the more commonly used os.truncate
works with file names. In our example, since you obtained a file descriptor with os.open
, you use os.ftruncate
to truncate the file directly using the file descriptor.
Below is an example that demonstrates how to use the os.ftruncate()
method when interacting with files.
Example:
import os
with open("File.txt", "w") as file:
file.write("Hello, World! Programming is so fun!")
fd = os.open("File.txt", os.O_RDWR)
print("The file size, in bytes, is:", os.stat(fd).st_size)
length = 15
os.ftruncate(fd, length)
size = os.stat(fd).st_size
print(os.read(fd, size).decode("utf-8"))
print("The file size, in bytes, after truncating:", os.stat(fd).st_size)
os.close(fd)
First, we import the os
module, making all of its functions and attributes accessible in the current Python script. We then open a file named File.txt
in write mode (w
).
The with
statement ensures that the file is properly closed after writing. The string Hello, World! Programming is so fun!
is then written to the file.
Now we open the file File.txt
and return a file descriptor (fd
), which is an integer representing the file, and os.O_RDWR
indicates that the file is opened for both reading and writing.
The os.stat(fd)
then returns a named tuple with several file-related information, and st_size
retrieves the size of the file in bytes. We then print the current file size to the console.
With the variable length
set to 15
, os.ftruncate(fd, length)
truncates the file pointed to by the file descriptor fd
to the specified length (15
in this case).
When you call os.ftruncate(fd, length)
, it adjusts the size of the file associated with the given file descriptor (fd
). If the file is larger than the specified length, the extra data at the end of the file is discarded.
The os.stat(fd).st_size
is used again to get the updated size of the file after truncation, and os.read(fd, size)
reads the content of the file up to the new size. The content is then decoded from bytes to a UTF-8 encoded string and printed to the console along with the final file size after truncation.
Finally, we close the file descriptor with os.close(fd)
. Closing the file descriptor releases system resources associated with the open file, and it’s important for proper resource management in your program.
Output:
The file size, in bytes, is: 36
Hello, World! P
The file size, in bytes, after truncating: 15
In summary, this code creates a file, writes a string to it, truncates the file to a specified length, reads and prints the truncated content, and then prints the updated file size after truncation.
Example Codes: Use the os.ftruncate()
Method With Length Exceeding the File Size in Python
If we set the length to be greater than the current file size using os.ftruncate()
, it will increase the size of the file by padding it with null bytes. This is also known as extending the file.
Below is an example showcasing this instance.
Example:
import os
with open("File.txt", "w") as file:
file.write("Hello, World! Programming is so fun!")
fd = os.open("File.txt", os.O_RDWR)
print("The file size, in bytes, is:", os.stat(fd).st_size)
length = 100
os.ftruncate(fd, length)
size = os.stat(fd).st_size
print(os.read(fd, size).decode("utf-8"))
print("The file size, in bytes, after truncating:", os.stat(fd).st_size)
os.close(fd)
In the code above, the only difference here is that the length
is now set to 100
.
Like the previous example mentioned, when you call os.ftruncate(fd, length)
, it adjusts the size of the file associated with the given file descriptor (fd
). But If the file is smaller than the specified length, it is extended, and the extended portion is filled with null bytes.
Output:
The file size, in bytes, is: 36
Hello, World! Programming is so fun!
The file size, in bytes, after truncating: 100
In this example, the File.txt
content did not change to its original size. But to increase the file size to the entered length, it got filled with random invalid characters.
Example Codes: Use the os.ftruncate()
Method to Empty a File
Emptying a file using the os.ftruncate()
method in Python involves truncating the file to a size of 0
bytes, effectively removing all content from the file.
Setting the length to 0
using os.ftruncate()
will not directly delete the file, but it will effectively truncate the file to an empty size, giving the appearance of an empty file. The file still exists, but its content will be removed.
Example:
import os
with open("File.txt", "w") as file:
file.write("Hello, World! Programming is so fun!")
fd = os.open("File.txt", os.O_RDWR)
print("The file size, in bytes, is:", os.stat(fd).st_size)
length = 0
os.ftruncate(fd, length)
size = os.stat(fd).st_size
print(os.read(fd, size).decode("utf-8"))
print("The file size, in bytes, after truncating:", os.stat(fd).st_size)
os.close(fd)
In this example, the file is initially created with some content. Then, os.ftruncate(fd, 0)
is used to truncate the file associated with the file descriptor fd
to zero bytes.
After the truncation, the file is effectively empty, and its size becomes zero. Finally, the program prints the file size in the console to confirm that the file is now empty and closes the file descriptor with os.close(fd)
.
Output:
The file size, in bytes, is: 36
The file size, in bytes, after truncating: 0
As seen in the output, the file size is now zero bytes after truncating. The final output confirms that the file has been successfully truncated and the file descriptor is closed.
In summary, the code demonstrates how to open a file, write content to it, get its original size, truncate it to empty, read and print its content (which is an empty string in this case), and confirm the change in file size.
Conclusion
In this exploration of the os.ftruncate()
method in Python, we have navigated through the intricacies of file manipulation. os.ftruncate()
emerges as a powerful tool for resizing files, offering the flexibility to truncate at specific lengths or the current file position.
By understanding file descriptors and utilizing functions from the os
module, we can create, write to, and effectively manage files.
This journey provides valuable insights into employing os.ftruncate()
for tailored file size adjustments, offering a comprehensive approach to handling files in Python with precision and efficiency.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn