Python os.supports_effective_ids Method
-
Syntax of Python
os.supports_effective_ids
Method -
Example 1: Use the
os.supports_effective_ids
Method in Python -
Example 2:
NotImplementedError
Exception in theos.supports_effective_ids
Method -
Example 3: Define the
check_filewritable()
Function Withos.supports_effective_ids
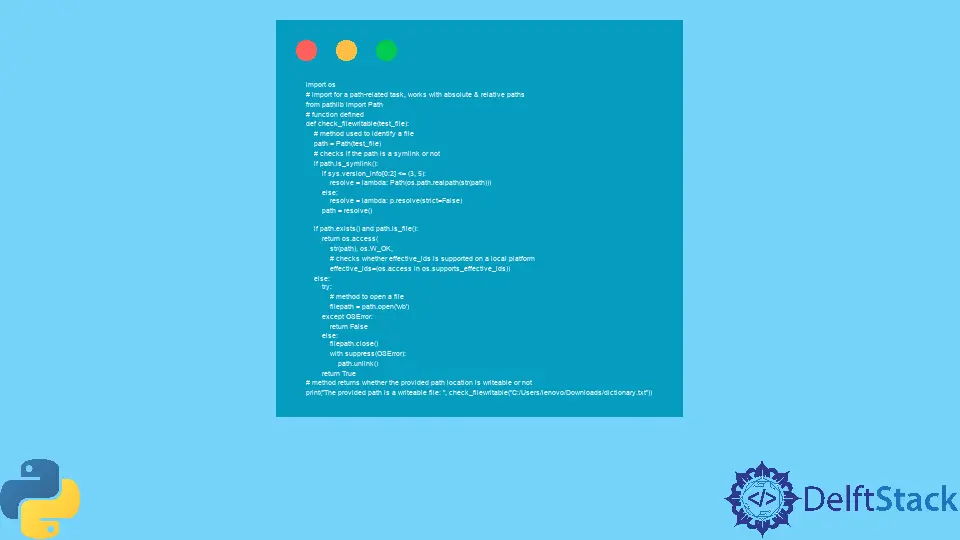
We use the OS module to interact with the operating system using Python. This module is widely used for this purpose and contains several other methods to facilitate the users.
One of the method is os.supports_effective_ids
method, which checks and indicates whether os.access()
method supports the effective_ids = True
on a local machine. If the method supports the platform, it shows True
; otherwise, it shows False
.
On Python version 3.3, the method os.supports_effective_ids
supports Unix operating system and has no support for Windows operating system. To further understand this method, let’s see its syntax, parameters, return type and a few examples.
Syntax of Python os.supports_effective_ids
Method
os.access in os.supports_effective_ids
Parameter
effective_ids
- a parameter used to check whether the method has support on a local platform or not.
Return
This method returns a Boolean value, either True
or False
. An object called set
shows whether method os.access()
permits True
for the parameter effective_ids
on a local machine or False
if it is not supported.
Example 1: Use the os.supports_effective_ids
Method in Python
In Python, we use the method os.supports_effective_ids
to check whether the local platform supports the effective_ids
value as True
using the os.access()
method.
The os.access()
method shows a True
Boolean value on the console if the effective_ids
has support and to check the support, we need the os.supports_effective_ids
method.
To prove the above discussion about the method, let’s take the example below implementation on Windows and Unix operating systems.
In the case of the Unix operating system, the value of effective_ids
returns as True
. The os.access()
method can do the access checks using the effective_ids
instead of real ids.
Windows Operating System
# import the os module to use its methods for OS interaction
import os
# to check the support of effective_ids in Windows OS
print(
"The effective ids support on Windows OS: ", os.access in os.supports_effective_ids
)
Output:
The effective ids support on Windows OS: False
Unix Operating System
import os
# to check the support of effective_ids in Unix OS
print("The effective ids support on Unix OS: ", os.access in os.supports_effective_ids)
Output:
The effective ids support on Unix OS: True
Example 2: NotImplementedError
Exception in the os.supports_effective_ids
Method
Before Python version 3.3, if the method is not available, the method shows the exception and outputs NotImplementedError
on the console. In the later versions or after Python version 3.3, if the method is unavailable or does not support, it returns False
.
Look at the below example, where we see the exception found in the method.
import os
# to check the support of effective_ids
print("Exception found: ", os.access in os.supports_effective_ids)
Output:
Exception found: NotImplementedError exception
Example 3: Define the check_filewritable()
Function With os.supports_effective_ids
In this example, we define a method check_filewritable()
and pass a valid file location to it. The function returns a Boolean value, either True
if the file is valid and writeable or False
if the file location is invalid.
The below example is the perfect demonstration of the method os.supports_effective_ids
with comments explanation.
import os
# import for a path-related task, works with absolute & relative paths
from pathlib import Path
# function defined
def check_filewritable(test_file):
# method used to identify a file
path = Path(test_file)
# checks if the path is a symlink or not
if path.is_symlink():
if sys.version_info[0:2] <= (3, 5):
def resolve():
return Path(os.path.realpath(str(path)))
else:
def resolve():
return p.resolve(strict=False)
path = resolve()
if path.exists() and path.is_file():
return os.access(
str(path),
os.W_OK,
# checks whether effective_ids is supported on a local platform
effective_ids=(os.access in os.supports_effective_ids),
)
else:
try:
# method to open a file
filepath = path.open("wb")
except OSError:
return False
else:
filepath.close()
with suppress(OSError):
path.unlink()
return True
# method returns whether the provided path location is writeable or not
print(
"The provided path is a writeable file: ",
check_filewritable("C:/Users/lenovo/Downloads/dictionary.txt"),
)
Output:
The provided path is a writeable file: True