Python os.access() Method
-
Syntax of Python
os.access()
Method -
Example 1: Use the
os.access()
Method With Different Modes in Python -
Example 2: Use Conditional Statement With the
os.access()
Method in Python -
Example 3: Write Into File After Using the
os.access()
Method in Python -
Example 4: Check File Existence Using the
os.access()
Method in Python - Example 5: Checking Read Permission
- Example 6: Checking Write Permission
- Example 7: Checking Execute Permission
- Use Cases
- Conclusion
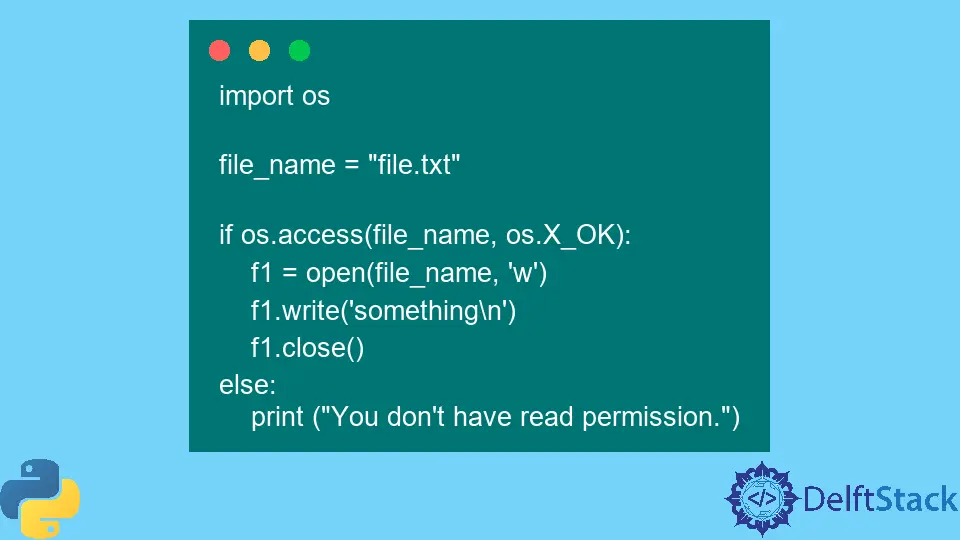
The os.access()
method in Python is a utility that allows you to check the accessibility of a file according to the provided mode. This method is part of the os
module, which provides a way to interact with the operating system.
Python’s os.access()
method is an efficient way of using the OS-dependent functionality. This method uses the uid
/gid
(user identifier/group identifier) to test for access to a path/folder.
Syntax of Python os.access()
Method
The os.access()
method determines whether a specified file can be accessed in the given mode.
os.access(path, mode)
Parameters
path |
The path of the file or directory to be checked. The path is used for existence, validity, or existence mode. |
mode |
The access mode to be tested. The mode tells which characteristics of the path we are checking. |
To test the existence of a path, we use os.F_OK . |
|
To test the readability of a path, we use os.R_OK . |
|
To test if the path is writeable or not, we use the mode os.W_OK . |
|
The last mode is os.X_OK , which is used to determine if the path can be executed or not. |
Return Value
The return type of this method is a Boolean value:
- Returns
True
if the specified access is allowed. - Returns
False
if the specified access is not allowed or the path doesn’t exist.
Example 1: Use the os.access()
Method With Different Modes in Python
The example below uses the os.access()
method to check different permissions associated with the file "file.txt"
. It verifies existence, reads, writes, and executes permissions, and prints the results for each check.
The os.access()
method, combined with various flags like os.F_OK
, os.R_OK
, os.W_OK
, and os.X_OK
, examines specific permissions and returns True
if the permission is granted and False
otherwise. The printed output displays the status of each permission check.
import os
directory1 = os.access("file.txt", os.F_OK)
print("Does the path exist? :", directory1)
directory2 = os.access("file.txt", os.R_OK)
print("Access to read the file granted :", directory2)
directory3 = os.access("file.txt", os.W_OK)
print("Access to write the file granted :", directory3)
directory4 = os.access("file.txt", os.X_OK)
print("Is the path executable?:", directory4)
Output:
Does the path exist? : False
Access to read the file granted : False
Access to write the file granted : False
Is the path executable?: False
The os.access()
may not work on some operating systems. You can try writing import sys
at the start of the code to include the required libraries per your system requirement.
Example 2: Use Conditional Statement With the os.access()
Method in Python
Use the os.access()
method to check if a user is authorized to open a file before it is made available.
import os
if os.access("file.txt", os.R_OK):
with open("file.txt") as file:
a = file.read()
print(a)
else:
print("An error has occurred.")
Output:
An error has occurred.
This example uses the os.access()
method to check if the file "file.txt"
has read
(os.R_OK
) permission.
If the file has “read” permission, it proceeds to open and read the file content using a with
statement to handle file operations. It then prints the content of the file to the console.
However, suppose the file does not have read
permission (os.access()
returns False
). In that case, it prints an error message indicating that an error has occurred, bypassing the attempt to open and read the file to avoid potential permission-related issues.
Example 3: Write Into File After Using the os.access()
Method in Python
This example checks the file permissions using os.access()
for the file named "file.txt"
. Specifically, it checks if the file has execute
(os.X_OK
) permission.
If the file has “execute” permission (os.access()
returns True
), it opens the file in write mode ("w"
), writes the string "something\n"
into it, and then closes the file (f1.close()
).
However, if the file does not have “execute” permission (os.access()
returns False
), it prints a message indicating that the user does not have the required permissions to write to the file.
import os
file_name = "file.txt"
if os.access(file_name, os.X_OK):
f1 = open(file_name, "w")
f1.write("something\n")
f1.close()
else:
print("You don't have read permission.")
Output:
You don't have read permission.
I/O file operations may fail even when os.access()
returns true
because some network file systems may have permissions semantics beyond the usual POSIX permission-bit model.
Example 4: Check File Existence Using the os.access()
Method in Python
This example checks for the existence and accessibility of a file named "example.txt"
using Python’s os.access()
method. If the file exists and is accessible, it prints "File exists"
; otherwise, it prints "File does not exist or is inaccessible"
.
import os
file_path = "example.txt"
if os.access(file_path, os.F_OK):
print("File exists")
else:
print("File does not exist or is inaccessible.")
Example 5: Checking Read Permission
This example employs os.access()
to verify if a file ('example.txt'
) has read
permission. If the file is readable, it prints "File is readable"
; otherwise, if it lacks read
permission or is inaccessible, it prints "File is not readable or is inaccessible"
.
import os
file_path = "example.txt"
if os.access(file_path, os.R_OK):
print("File is readable")
else:
print("File is not readable or is inaccessible.")
Example 6: Checking Write Permission
This example uses the os.access()
method to check if the file named "example.txt"
has write
(os.W_OK
) permission. If the file is writable, it prints "File is writable"
; otherwise, it prints "File is not writable or is inaccessible"
.
import os
file_path = "example.txt"
if os.access(file_path, os.W_OK):
print("File is writable")
else:
print("File is not writable or is inaccessible.")
Example 7: Checking Execute Permission
This example uses the os.access()
method in Python to check whether the file at the specified path (example.sh
) has execute
permission (os.X_OK
). If the file is executable, it prints "File is executable"
; otherwise, it prints "File is not executable or is inaccessible"
.
import os
file_path = "example.sh"
if os.access(file_path, os.X_OK):
print("File is executable")
else:
print("File is not executable or is inaccessible.")
Use Cases
- Security Checks: Validate permissions on files before performing any operation.
- File Handling: Determine if a file can be opened for reading, writing, or execution.
Notes
- The method’s behavior may differ across different operating systems.
- Some permission checks might not work as expected due to system-level permissions or user privileges.
Conclusion
os.access()
provides a straightforward way to check file accessibility based on the specified mode. It’s a handy tool for file manipulation, ensuring that your code can safely interact with files without running into permission-related errors.
However, it’s important to consider system-specific behaviors and the context in which the method is used for accurate file access validation in Python programs.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn