Python os.readlink() Method
- What is a Symbolic Link
-
Introduction to
os.readlink()
-
Syntax of Python
os.readlink()
Method -
Example 1: Use Python
os.readlink()
Method -
Example 2: Find the Absolute Path Using the
os.readlink()
Method in Python -
Example 3: Understanding the
os.readlink()
Method in Python - Example 4: Error Handling
- Real-world Applications
- Conclusion
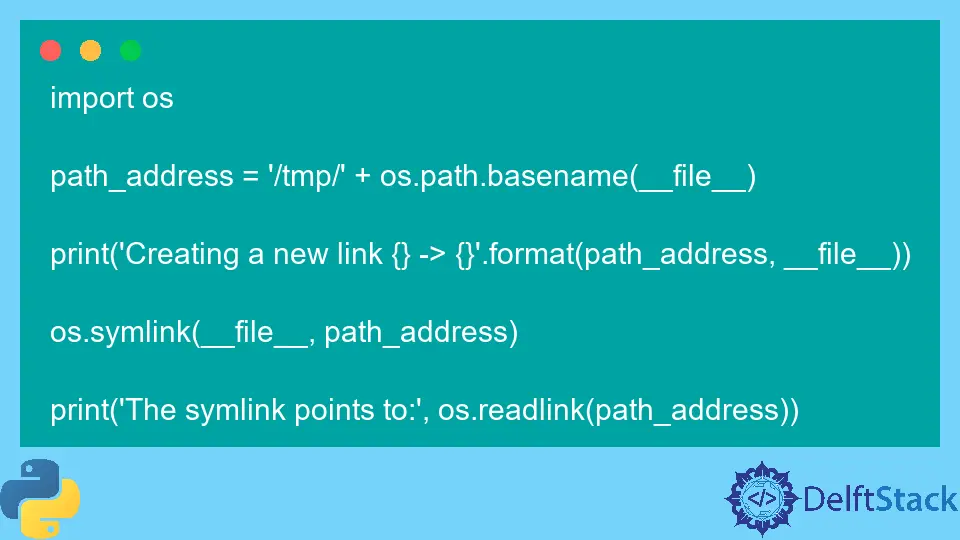
In the realm of Python programming, the os
module serves as a powerful tool for interacting with the operating system. One of its lesser-known yet incredibly useful functions is os.readlink()
.
This method specifically deals with symbolic links, providing a means to extract the path or target of a symbolic link concisely and efficiently.
Python os.readlink()
method is an efficient way of finding a path using a symbolic link. It may return an absolute or a relative path of the symbolic link to which it relates.
What is a Symbolic Link
Before delving into the intricacies of the os.readlink()
method, it’s essential to grasp the concept of symbolic links. Also known as symlinks
or soft links, these are references to a file or directory that act as pointers to another file or directory in the filesystem.
Unlike hard links, symbolic links are independent entities. Changes to the original file do not affect the symlink
.
Introduction to os.readlink()
The os.readlink()
method falls under the umbrella of file-related functions in the os
module. Its primary purpose is to retrieve the path or target of a symbolic link.
The method takes a single argument, the path of the symbolic link, and returns a string representing the path or target of the link.
Syntax of Python os.readlink()
Method
os.readlink(path address)
os.readlink(path address, *, fd_dir=None)
Parameters
path address |
It is an address object of a file system path or a symlink . The object can either be an str or bytes. |
* |
The asterisk shows that all following parameters are keyword-only. |
fd_dir |
It is an optional parameter representing a file descriptor referring to a path. Its default value is set as None . |
Return
The return type of this method is a path string relating to the symlink
entered as the parameter. A string object is returned if the symlink
is also a string object, and a byte object is returned if the symlink
is a byte object.
Example 1: Use Python os.readlink()
Method
This code uses the os
module to create and interact with symbolic links in a Unix-like file system. It starts by defining a directory path (path_address
) and a symbolic link (link
) to a file within that directory.
The code then checks if the symbolic link already exists using os.path.exists()
. If it doesn’t exist, the os.symlink()
function is used to create a symbolic link from the specified link to the directory path.
The code then prints a message indicating whether the symbolic link was created or already existed. Finally, the code reads the target of the symbolic link using os.readlink()
and prints the path to which the symbolic link points.
import os
path_address = "/home"
link = "/home/File.txt"
# Check if the symbolic link already exists
if not os.path.exists(link):
os.symlink(path_address, link)
print(f"Symbolic link created from {link} to {path_address}")
else:
print(f"Symbolic link {link} already exists")
# Read the symbolic link
Path = os.readlink(link)
print("The symbolic link points to", Path)
Output:
Symbolic link created from /home/File.txt to /home
The symbolic link points to /home
An OSError
might occur while using the os.readlink()
method due to invalid or inaccessible processes and paths.
Example 2: Find the Absolute Path Using the os.readlink()
Method in Python
This code defines a directory path (path_address
) and a symbolic link (link
) pointing to a file within that directory. The code checks if the symbolic link already exists using os.path.exists()
.
If it doesn’t, the code creates the symbolic link using os.symlink()
and prints a message indicating the creation. If the symbolic link already exists, it prints a message acknowledging its existence.
After that, the code uses os.readlink()
to retrieve the target of the symbolic link and then prints the absolute path to which the symbolic link points using os.path.realpath()
. This provides information about the actual file or directory associated with the symbolic link.
import os
path_address = "/home"
link = "/home/File.txt"
# Check if the symbolic link already exists
if not os.path.exists(link):
os.symlink(path_address, link)
print(f"Symbolic link created from {link} to {path_address}")
else:
print(f"Symbolic link {link} already exists")
# Read the symbolic link
Path = os.readlink(link)
# Print the absolute path to which the symbolic link points
print(
"The symbolic link points to the following absolute path:", os.path.realpath(Path)
)
Output:
Symbolic link created from /home/File.txt to /home
The symbolic link points to the following absolute path: /home
When resolving a path containing links, you can use os.path.realpath()
to properly handle recursion and systems and platform differences.
Example 3: Understanding the os.readlink()
Method in Python
This code utilizes the os
module to create a symbolic link to the current script file. It specifies a desired path for the symbolic link (path_address
).
The code then prints a message indicating the intention to create a symbolic link from the specified path to the current script file (__file__
). It checks if the symbolic link already exists using os.path.exists()
.
If it doesn’t exist, the code creates the symbolic link using os.symlink()
and prints the path to which the symlink
points using os.readlink()
. If the symbolic link already exists, it prints a message acknowledging its existence.
This script essentially automates the process of creating a symbolic link to the current script file at a user-specified location.
import os
path_address = "/tmp/my_temp_file.py" # Change this to a desired name
print("Creating a new link {} -> {}".format(path_address, __file__))
# Check if the symbolic link already exists
if not os.path.exists(path_address):
os.symlink(__file__, path_address)
print("The symlink points to:", os.readlink(path_address))
else:
print("Symbolic link {} already exists".format(path_address))
Output:
Creating a new link /tmp/my_temp_file.py -> /home/main.py
The symlink points to: /home/main.py
Note that the file descriptors returned by the method os.readlink()
are non-inheritable.
Example 4: Error Handling
If the specified path is not a symbolic link, the os.readlink()
method raises an OSError
. Therefore, it’s crucial to handle exceptions appropriately when using this method.
import os
try:
target_path = os.readlink("path/to/symlink")
print("Target path:", target_path)
except OSError as e:
print(f"Error: {e}")
Output:
Error: [Errno 2] No such file or directory: 'path/to/symlink'
In this example, an attempt is made to read the target path of a nonexistent symbolic link, leading to an OSError
.
Real-world Applications
The os.readlink()
method finds applications in various scenarios, including:
- Configuration Files: Retrieving the path to configuration files linked from a common location.
- Dependency Management: Determining the paths of linked libraries or modules in a project.
- Dynamic File Routing: Managing dynamic routing of files based on symbolic links.
Conclusion
The os.readlink()
method in Python is a valuable tool for dealing with symbolic links. Its ability to efficiently extract the target path of a symbolic link makes it a go-to choice in scenarios where file or directory references need to be resolved dynamically.
By understanding the nuances of this method, Python developers can enhance their ability to work with symbolic links effectively, contributing to more robust and flexible file handling in their applications.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn