Python os.path.samefile() Method
-
Syntax of Python
os.path.samefile()
Method -
Example 1: Use the
os.path.samefile()
Method to Check if Paths Refer to the Same File or Directory -
Error Occurrence When Using the
os.path.samefile()
Method:OSError: symbolic link privilege not held
-
Example 2: Use the
os.getcwd()
Method With theos.path.samefile()
Method -
Example 3: Make Your Own
os.path.samefile()
Method - Conclusion
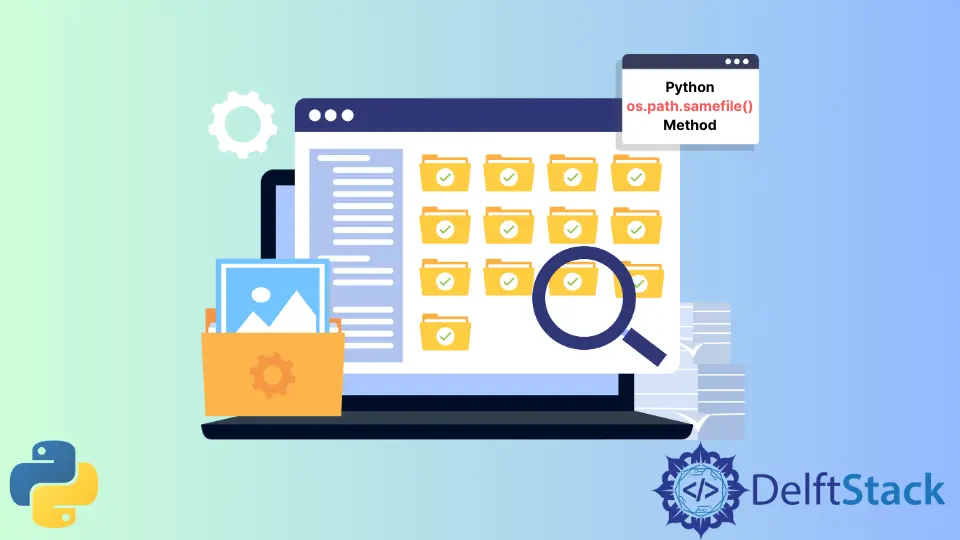
The os.path
module provides numerous functions for such operations, one of which is os.path.samefile()
. This function allows you to determine if two file paths point to the same file or directory on the filesystem, even if they have different path representations.
The working mechanism behind this method is to compare the inode number and device number of the given paths.
Syntax of Python os.path.samefile()
Method
os.path.samefile(path1, path2)
Parameters
path1 |
It is an address object of the first file system path or a symlink. The object can either be an str or bytes. |
path2 |
It is an address object of the second file system path or a symlink. The object can either be an str or bytes. |
Return
The return type of this method is a Boolean value representing true
if both the file paths refer to the same directories.
Example 1: Use the os.path.samefile()
Method to Check if Paths Refer to the Same File or Directory
Often, developers encounter scenarios where they need to compare file paths to ascertain whether they point to the same file or directory on the filesystem.
The os.path.samefile
function comes to the rescue in such situations, providing a reliable means to determine file identity regardless of the path representation used.
The example code snippet below demonstrates the usage of os.path.samefile
to compare two file paths and check if they point to the same file.
File.txt
:
Sample text.
Example Code:
import os
# Define the first file path
path1 = "C:/Users/786/Documents/File.txt"
# Define a symbolic link to the first file
link = "C:/Users/786/Desktop/File(shortcut).txt"
# Create a symbolic link pointing to path1
os.symlink(path1, link)
# Check if the paths point to the same file
similarity = os.path.samefile(path1, link)
# Print the result of the comparison
print("Are the paths pointing to the same file? ", similarity)
The code snippet demonstrates the creation of a symbolic link and comparison of file paths using Python’s os
module.
Initially, the absolute paths of two files are defined: path1
, representing a file named "File.txt"
in the “Documents” directory, and link
, representing a symbolic link named "File(shortcut).txt"
pointing to path1
and residing on the Desktop.
By utilizing os.symlink
, a symbolic link named "File(shortcut).txt"
is created, pointing to the file specified by path1
. The os.path.samefile
function then compares the absolute paths of path1
and link
to determine if they point to the same file, returning True
if they do and False
otherwise.
Finally, the result of the comparison is printed to the console, indicating whether the paths point to the same file.
Output:
Are the paths pointing to the same file? True
This output confirms that the paths specified by path1
and link
indeed point to the same file on the filesystem, as indicated by the return value of os.path.samefile
.
In the background, this method uses the os.stat()
method to get device numbers and inode numbers of the specified paths.
Error Occurrence When Using the os.path.samefile()
Method: OSError: symbolic link privilege not held
The OSError: symbolic link privilege not held
error typically occurs when attempting to create a symbolic link without the necessary permissions.
Symbolic links are a type of file that points to another file or directory. Creating symbolic links may require elevated privileges, especially on certain operating systems or in specific directories.
Here are some potential reasons why you encountered this error:
-
Insufficient Permissions: You may not have the necessary permissions to create symbolic links in the specified directory. This could be due to restrictions imposed by the operating system or file system permissions.
-
Operating System Limitations: Some operating systems restrict the creation of symbolic links in certain directories for security reasons. For instance, creating symbolic links in system directories may require administrative privileges.
-
File System Limitations: Certain file systems may impose restrictions on symbolic link creation. For example, network file systems (NFS) or file systems mounted with specific options may limit symbolic link creation.
-
Antivirus or Security Software: In some cases, antivirus or security software running on your system may interfere with symbolic link creation, especially if it detects it as a potentially malicious action.
To resolve this issue, you can try the following steps:
-
Run as Administrator/Superuser
If you’re running the code on a system where administrative privileges are required to create symbolic links, try running your Python script with elevated permissions. On Windows, you can right-click the Command Prompt or PowerShell and select
Run as administrator
. On Unix-based systems, you can usesudo
to run the script with superuser privileges. -
Check File System Permissions
Ensure that you have the necessary permissions to create symbolic links in the specified directory. You may need to adjust the file system permissions or move the operation to a directory where you have appropriate permissions.
-
Disable Antivirus/Security Software
Temporarily disable any antivirus or security software running on your system and try creating the symbolic link again. If the operation succeeds, consider adding an exception or adjusting the settings of the security software to allow symbolic link creation.
By addressing the underlying cause of the OSError: symbolic link privilege not held error
and taking appropriate actions, you should be able to resolve the issue and create symbolic links successfully in your Python code.
Example 2: Use the os.getcwd()
Method With the os.path.samefile()
Method
The code snippet below illustrates the usage of os.path.samefile
to compare two file paths and determine if they point to the same file on the filesystem.
This comparison is valuable in scenarios where files are referenced by different paths, but their actual identities need verification.
File.txt
:
Sample text.
Example Code:
import os
# Open/create a file named "File.txt"
fd = os.open("File.txt", os.O_RDWR | os.O_CREAT)
# Define the absolute path of the first file
path1 = "File.txt"
# Define the absolute path of the second file using os.path.join
path2 = os.path.join(os.getcwd(), "File.txt")
# Compare the absolute paths of path1 and path2
similarity = os.path.samefile(path1, path2)
# Print the result of the comparison
print("Are the paths pointing to the same file? ", similarity)
The code snippet showcases the utilization of Python’s os
module for file manipulation tasks.
Firstly, the code imports the os
module to gain access to operating system functionalities, particularly those pertaining to file operations.
Using the os.open
function, a file named "File.txt"
is either opened or created in read-write mode, with the os.O_CREAT
flag specifying that the file should be created if it doesn’t already exist.
Two absolute file paths, path1
and path2
, are then defined: path1
represents the file "File.txt"
located in the directory where the code script has executed, while path2
is constructed by combining the current working directory with the filename File.txt
using os.path.join
.
The os.path.samefile
function is employed to compare the absolute paths of path1
and path2
, determining whether they point to the same file on the filesystem.
Finally, the outcome of the comparison is printed to the console, providing clarity on whether the paths indeed reference the same file.
Output:
Are the paths pointing to the same file? True
This output confirms that both path1
and path2
indeed point to the same file on the filesystem, as indicated by the True
result returned by os.path.samefile
.
Note that you might have written the correct code for the os.path.samefile()
method, and still, an exception is thrown. This might be because the os.stat()
call fails on either file pathnames.
Example 3: Make Your Own os.path.samefile()
Method
The code snippet below showcases the comparison of two file paths in Python, aiming to determine if they point to the same file on the filesystem.
This task is facilitated by the custom function os_path_samefile
, which utilizes the os
module to access operating system functionalities, particularly those pertaining to file operations.
The function evaluates whether the files specified by the input paths have identical device numbers (st_dev
) and inode numbers (st_ino
), which are attributes associated with files on Unix-like operating systems.
File.txt
:
Sample text.
Example Code:
import os
def os_path_samefile(path1, path2):
# Retrieve file stats for path1 if it exists
stat1 = os.stat(path1) if os.path.isfile(path1) else None
if not stat1:
return False
# Retrieve file stats for path2 if it exists
stat2 = os.stat(path2) if os.path.isfile(path2) else None
if not stat2:
return False
# Compare device and inode numbers to determine file similarity
return (stat1.st_dev == stat2.st_dev) and (stat1.st_ino == stat2.st_ino)
# Open or create a file named "File.txt"
fd = os.open("File.txt", os.O_RDWR | os.O_CREAT)
# Define the absolute path of the first file
path1 = "File.txt"
# Construct the absolute path of the second file
path2 = os.path.join(os.getcwd(), "File.txt")
# Check if the paths point to the same file using custom function
similarity = os_path_samefile(path1, path2)
# Print the result of the comparison
print("Are the paths pointing to the same file? ", similarity)
The custom function os_path_samefile
is defined, which takes two file paths (path1
and path2
) as input parameters. It first checks if the paths point to existing files using os.path.isfile
, retrieving file stats (stat1
and stat2
) if they exist.
If either of the paths does not represent an existing file, the function returns False
, indicating that they cannot point to the same file.
The function then compares the device and inode numbers of the files (if they exist) to determine if they are the same. If both device and inode numbers match, the function returns True
, signifying that the paths indeed point to the same file.
The code creates a file named "File.txt"
using os.open
and defines two file paths (path1
and path2
), one absolute and the other constructed using os.path.join
with the current working directory.
The os_path_samefile
function is invoked to compare path1
and path2
, determining whether they point to the same file.
Finally, the outcome of the comparison is printed to the console, indicating whether the paths reference the same file.
Output:
Are the paths pointing to the same file? True
This output confirms that both path1
and path2
indeed point to the same file on the filesystem, as indicated by the True
result returned by the os_path_samefile
function.
In some versions of Python, the original os.path.samefile()
method might not be available so that you can make your method.
Conclusion
In conclusion, this article elaborates on the os.path.samefile()
method in Python, which is used to determine if two file paths reference the same file or directory on the filesystem.
Initially, the document outlines the importance of this function within the broader context of file manipulation tasks. It highlights the significance of accurately comparing file paths, particularly in scenarios where files are referenced by different paths.
The syntax and parameters of the method are elucidated, along with a concise explanation of its return value. Subsequently, the material presents multiple examples demonstrating the usage of os.path.samefile()
in different scenarios, accompanied by detailed code explanations and outputs.
Additionally, it addresses potential errors that users might encounter while employing this method and provides troubleshooting steps to resolve them.
Finally, the document concludes by mentioning that users can create their own os.path.samefile()
method if necessary, particularly in environments where the original method is unavailable.
This comprehensive overview equips readers with a thorough understanding of os.path.samefile()
and empowers them to utilize it effectively in their Python projects.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn