Python os.path.ismount() Method
-
Syntax of Python
os.path.ismount()
: -
Example Codes: Use the
os.path.ismount()
Method -
Example Codes: The
os.path.ismount()
Method ReturnsFalse
Instead of an Exception -
Example Codes: Difference Between the
os.path.ismount()
andpath.is_mount()
Methods -
Example Codes: Use the
os.path.ismount()
Method to Find the Mount Point
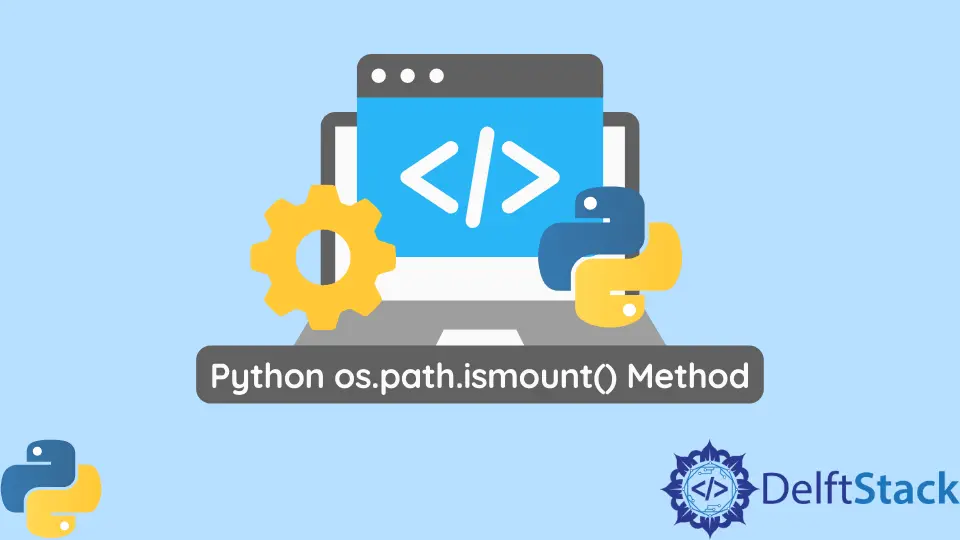
In Python, we use the OS module for using different path methods. These methods provide us with a facility to interact with the operating system.
To use the path methods, we use the os.path
as it is a sub-module of the OS module. One method we will discuss in this blog is os.path.ismount()
.
This method is used to check whether the specified path is a mount point or not. This means that a different file system has been mounted, called a point in a file system.
This method works on Windows, Unix, and Linux operating systems. However, this method’s behavior is different for all operating systems.
Syntax of Python os.path.ismount()
:
os.path.ismount(path)
Parameter
path |
This is the specified path. This can be a string or a byte representing a path-like object on a file system. |
Return
This method returns a Boolean value, either True
if the specified path is a mount point or False
if not a mount point.
Example Codes: Use the os.path.ismount()
Method
In the below example, we will use a simple example to check the usage of the os.path.ismount()
method. We will try passing the parameter path
to Windows and Unix operating systems.
-
Windows Operating System
In Windows operating system, we will check two scenarios, where we pass the
C:
drive as a path to theismount()
method and then pass theE:
drive to the same method.Let’s see the below demonstration and its source code. The output console shows that the root drive always returns
True
.
```python
# importing os.path module
import os.path
# On Windows, a root drive always shows the mount point
source_path = "C:"
# passing the path to the ismount method
is_mount_path = os.path.ismount(source_path)
print("ismount() method returns ", is_mount_path)
# checking another path for mount point
source_path = "D:"
is_mount_path = os.path.ismount(source_path)
print("ismount() method returns ", is_mount_path)
```
Output:
```text
ismount() method returns True
ismount() method returns False
```
-
Unix Operating System
Let’s take a similar example to test the
ismount()
method on the Unix operating system. Theos.path
methods work differently for various operating systems.
Let's see the example below to examine the behavior of the `ismount()` method.
```python
# import os.path module on Unix operating system
import os.path
# first source Path
source_path = "/dev"
is_mount_point = os.path.ismount(source_path)
print("ismount() method returns ", is_mount_point)
# second source Path
source_path = "/run"
is_mount_point = os.path.ismount(source_path)
print("ismount() method returns ", is_mount_point)
```
Output:
```text
ismount() method returns True
ismount() method returns False
```
Example Codes: The os.path.ismount()
Method Returns False
Instead of an Exception
In Python, the os.path.ismount()
method returns the exception if the specified path has characters or bytes. After version 3.8, the ismount()
method is changed and cannot return an exception; instead, it shows False
on the console.
Let’s see an example to check the output if a character is sent as a parameter to the ismount()
method and if it does not return an exception for an unpresentable path at the OS level.
import os.path
# character is passed as a parameter
source_path = "a"
# passing the path to the ismount method
is_mount_path = os.path.ismount(source_path)
print("ismount() method returns ", is_mount_path)
Output:
ismount() method returns False
Example Codes: Difference Between the os.path.ismount()
and path.is_mount()
Methods
The os.path
module returns the string, whereas the Pathlib
module returns the PosixPath object. With Pathlib
, we can do many more operations with the returned object.
If you execute a program with the path.is_mount()
method on the Windows operating system, then the output will say that Path.is_mount
is not supported on this system. However, we can use this method on different operating systems such as Unix or Linux.
-
Linux Operating System
Now, let’s take an example of the
path.is_mount()
method (assuming that we are not using the Windows operating system).
```python
# importing the pathlib module
from pathlib import Path
# source path for checking
source_path = Path("/some/mounted/dir/")
# Pathlib module is_mount() method
print(source_path.is_mount())
```
<!--adsense-->
Output:
```text
False
```
-
Windows Operating System
As we have already discussed, the behavior of this method varies according to the operating system. So, let’s test the above code on the Windows OS and discuss the following output shown on the console.
from pathlib import Path # source path for checking source_path = Path("/some/mounted/dir/") # Pathlib module is_mount() method print(source_path.is_mount())
Output:
```text
#do not use this pathlib module + is_mount() method on windows OS, or you will get this exception
NotImplementedError: Path.is_mount() is unsupported on this system
```
`NotImplementedError` is an exception thrown by the `ismount()` method when we try to run the above source code on the Windows OS.
Example Codes: Use the os.path.ismount()
Method to Find the Mount Point
In this example, we will pass the full path to the os.path.ismount()
method. The full path is passed to the real path, which is passed as a parameter.
We have to use the os.path.realpath()
method for the real path. This method is used when we want to return the canonical path of the file name provided.
Let’s examine the below source code to elaborate on the example further. We have defined a function mount_point_search()
method, which returns the mount point of the specified path.
import os.path
# method to search the mount point
def mount_point_search(path):
# to get the canonical path by removing the symbolic links
source_path = os.path.realpath(path)
# method needed to avoid an infinite loop
while not os.path.ismount(source_path):
source_path = os.path.split(source_path)[0]
# returns the mount point
return source_path
mount_point = mount_point_search("C:/Users/lenovo/Downloads/API Spec")
print("ismount() method returns the mount point as: ", mount_point)
Output:
ismount() method returns the mount point as: C:\
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn