Python os.path.isdir() Method
-
Syntax of the
os.path.isdir()
Method -
Example Codes: Working With the
os.path.isdir()
Method in Python -
Example Codes:
os.path.isdir()
vsos.path.exists()
vsos.path.isfile()
Methods -
Example Codes: Python
os.path.dir()
Method With Exception -
Example Codes: Use the
os.mkdir()
Method if theos.path.dir()
Method ReturnsFalse
-
Example Codes: Use the
os.path.dir()
Method to Check for a Symbolic Link
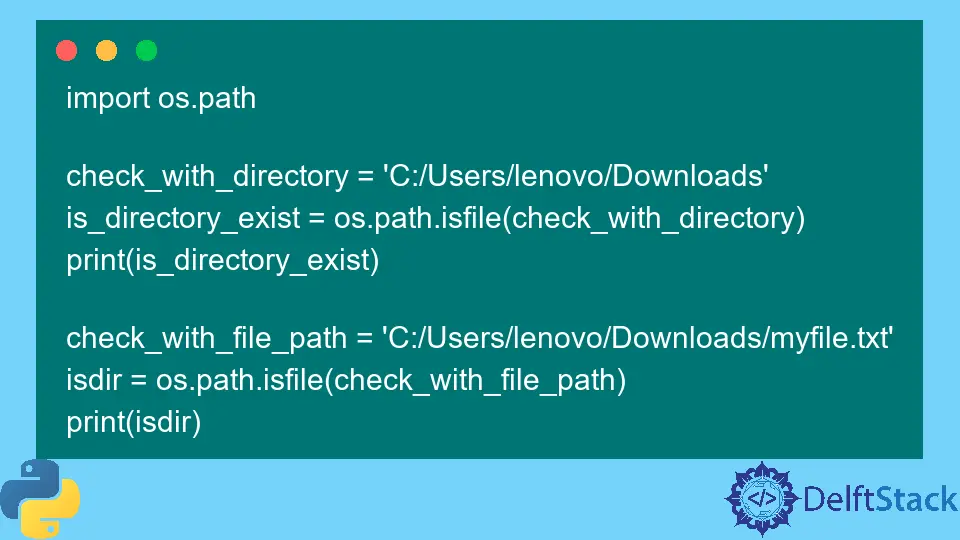
In Python, the method os.path.isdir()
is used to check whether the given path is a directory or not. Further, if the given path is a symbolic link, which means the path directs towards a link, then the method os.path.isdir()
will return true.
Syntax of the os.path.isdir()
Method
os.path.isdir(path)
Parameter
path |
This is the specified directory or path passed as a parameter to check whether it exists or not. This path can be a directory, a full path with filename + extension, or a symbolic link. |
Return
This method returns the Boolean value either True
or False
. It returns True
if the given path is a directory; otherwise, it returns False
.
Example Codes: Working With the os.path.isdir()
Method in Python
In this example, we will pass a directory path to the isdir()
method and check whether the path exists or not. In the function, os.path.isdir()
should be a directory or a file location with any extension.
Following is the example to check the valid directory with and without file extension inside the os.path.isdir()
method.
# importing os.path module for common path name interactions
import os.path
# directory to validate
check_path = "C:/Users/lenovo/Downloads"
# using the os.path.isdir method to check whether the path exists or not
is_directory_exist = os.path.isdir(check_path)
print(is_directory_exist)
# file path with the directory
check_path = "C:/Users/lenovo/Downloads/file.txt"
# check the directory along with the file extension
is_directory_exist = os.path.isdir(check_path)
print(is_directory_exist)
Output:
True #if the path is a directory
False #if the path is a directory along with filename + extension
In the above code, the function os.path.isdir()
returns true when the check_path
variable contains the valid directory path, and when the file path is given, it returns false.
Example Codes: os.path.isdir()
vs os.path.exists()
vs os.path.isfile()
Methods
We already discussed the os.path.isdir()
method in the above example. So, let’s look at the os.path.exists()
method, which is pretty similar in functionality.
The os.path.exists()
method will return true if the path is an existing directory or file. The only condition for it to return false is if the path is invalid (which means it does not exist).
import os.path
# valid directory as a parameter
check_with_directory = "C:/Users/lenovo/Downloads"
is_directory_exist = os.path.exists(check_with_directory)
print(is_directory_exist)
# valid full path directory as a parameter
check_with_file_path = "C:/Users/lenovo/Downloads/myfile.txt"
isdir = os.path.exists(check_with_file_path)
print(isdir)
Output:
True #this method shows True for both directory and full path file
True
As the name suggests, the os.path.isfile()
method will return true only when the path exists and is a file sent as a parameter. Otherwise, it will return as false.
import os.path
check_with_directory = "C:/Users/lenovo/Downloads"
is_directory_exist = os.path.isfile(check_with_directory)
print(is_directory_exist)
check_with_file_path = "C:/Users/lenovo/Downloads/myfile.txt"
isdir = os.path.isfile(check_with_file_path)
print(isdir)
Output:
False
True #this method returns True, only when the specified parameters contains the file path
Example Codes: Python os.path.dir()
Method With Exception
As we already know, the function os.path.dir()
returns a Boolean value. So, we can handle the exception with an if-else
condition to tell the user whether the path exists or not.
In the below program, the method projectDir()
handles the directory exception using the conditional statement. So, if the specified directory exists, it will output as existed or not existed depending on the condition.
import os.path
# method to handle the exception with an if-else statement
def projectDir():
directory = "C:/Users/lenovo/Downloads"
print("Check to see if", directory, "exist?")
# if-else statement to handle directory exception
if os.path.isdir(directory):
print(directory, "directory exists!")
else:
print(directory, "directory does not exist!")
projectDir()
Output:
Check to see if C:/Users/lenovo/Downloads exist?
C:/Users/lenovo/Downloads directory exists!
Example Codes: Use the os.mkdir()
Method if the os.path.dir()
Method Returns False
Suppose we follow the above example, where we check the directory exception with the if-else
statement. We can include the os.mkdir()
method, where the directory is not found (in the else
statement part).
In this way, if the directory is not found, the user can make a directory on the given path and show that a new one is made for your specified path. Let’s take an example code to demonstrate the situation further.
import os.path
def projectDir():
directory = "C:/Users/lenovo/Downloads/Junaid"
print("Check to see if", directory, "exist?")
if os.path.isdir(directory):
print(directory, " directory exists!")
else:
print(directory, "directory does not exist!")
# using os.mkdir() method to make a directory on a specified path
os.mkdir(directory)
print("A new directory is made on path: ", directory)
projectDir()
Output:
Check to see if C:/Users/lenovo/Downloads/Junaid exist?
C:/Users/lenovo/Downloads/Junaid direcotry does not exist!
A new directory is made on path: C:/Users/lenovo/Downloads/Junaid
Example Codes: Use the os.path.dir()
Method to Check for a Symbolic Link
See the example below to check the symbolic link for a specified path.
import os.path
directory = "Junaid"
os.mkdir(directory)
symlink = "C:/Users/lenovo/Downloads"
os.symlink(directory, symlink)
path = directory
is_directory_exist = os.path.isdir(path)
print(is_directory_exist)
path = symlink
is_directory_exist = os.path.isdir(path)
print(is_directory_exist)
Output:
True
True
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn