Python os.mkdir() Method
-
Syntax of the
os.mkdir()
Method -
Example 1: Create a Folder With the
os.mkdir()
Method in Python -
Example 2: Create a Folder at a Specific Location With the
os.mkdir()
Method in Python -
Example 3: Handle the
FileExistsError
Exception in Python
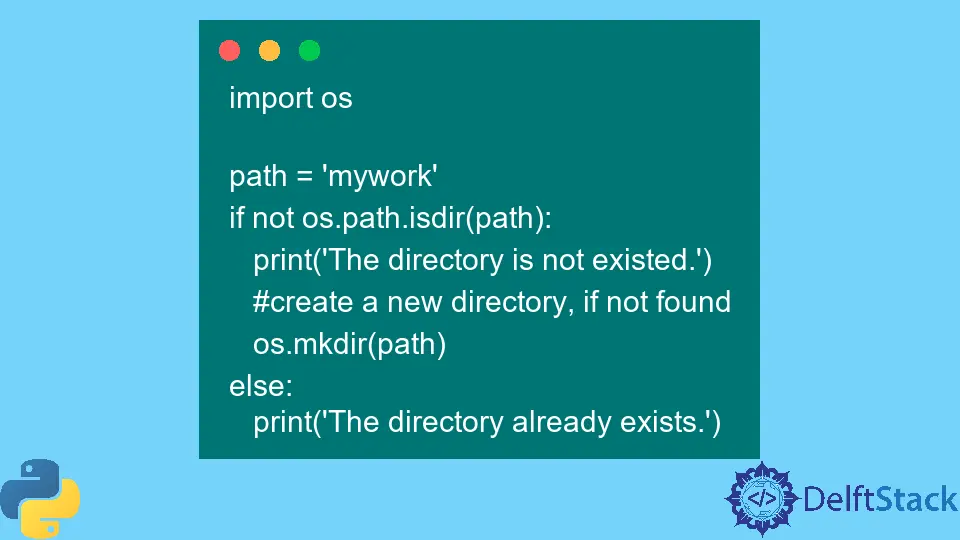
We use the os.mkdir()
in Python to create a directory to a specified path. A path is sent as an argument to the os.mkdir()
method for the directory folder, which is the only required argument for this function.
Syntax of the os.mkdir()
Method
os.mkdir(path, mode=0o777, *, dir_fd=None)
Parameters
path |
Create a directory named path . FileExistsError is raised if the path directory is already present. FileNotFoundError is raised if the parent path directory is not present. |
mode (optional) |
the mode is ignored in some systems |
* |
shows the following parameters (dir_fd ) are keyword-only |
dir_fd (optional) |
Its default value is None . dir_fd is ignored, if the given path is absolute. |
Return Value
This method doesn’t return any value.
Example 1: Create a Folder With the os.mkdir()
Method in Python
We will create a folder named mywork
by passing it as an argument inside the mkdir()
function.
import os
os.mkdir("mywork")
print("the folder is created successfully")
Output:
the folder is created successfully
The above source code will create the folder named mywork
inside the directory where you run the Python file. If you want to change the location of the newly created folder, then you can specify the complete directory location as an argument.
Let’s see the next example of how we can do so.
Example 2: Create a Folder at a Specific Location With the os.mkdir()
Method in Python
To create a folder at a specific location/directory, you must pass the complete path as an argument.
import os
os.mkdir("E:\mywork")
print("the folder is created successfully")
Output:
the folder is created successfully
Example 3: Handle the FileExistsError
Exception in Python
This FileExistsError
error will occur if you create an existing directory. In the first example, we already created the mywork
directory, let’s create it again from the same path, and we will see the error.
import os
# this directory is already created
os.mkdir("mywork")
print("the folder is created successfully")
Output:
Traceback (most recent call last):
File "/tmp/user_code.py", line 4, in <module>
os.mkdir("mywork")
FileExistsError: [WinError 183] Cannot create a file when that file already exists: 'mywork'
[Execution complete with exit code 1]
To provide a solution to this error on runtime, we can place a check to find whether a directory is already there or not. We can solve the above issue by using the following source code.
import os
path = "mywork"
if not os.path.isdir(path):
print("The directory is not existed.")
# create a new directory, if not found
os.mkdir(path)
else:
print("The directory already exists.")
Output:
The directory already exists.
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn