Python os.path.getsize() Method
-
Syntax of the
os.path.getsize()
Method -
Example 1: Working With the
os.path.getsize()
Method in Python -
Example 2: Handling
OSError
While Using theos.path.getsize()
Method in Python -
Example 3: Set the Path as Empty in the
os.path.getsize()
Method in Python
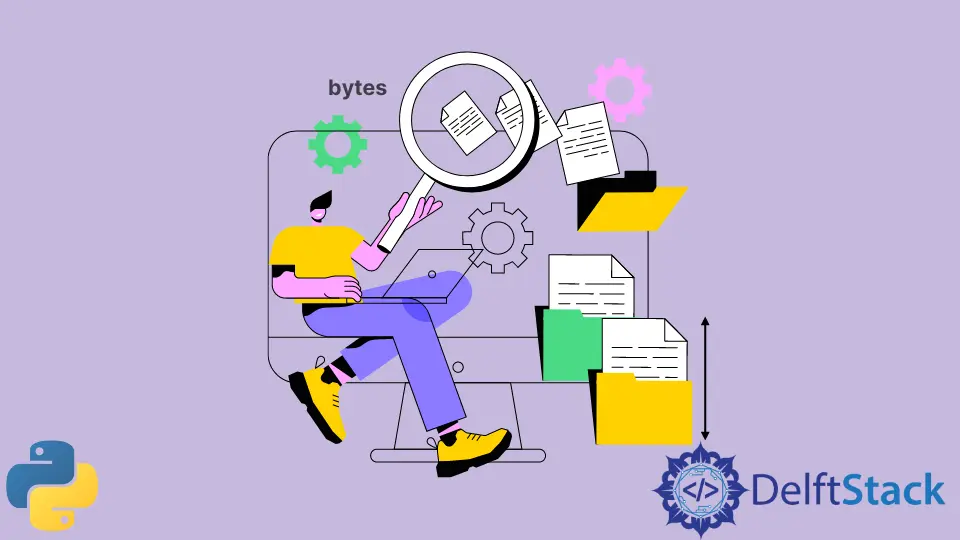
Python os.path.getsize()
method is the most efficient way of finding the size of a file/path/link. The os.path.getsize()
method throws an OSError
if the specified file does not exist or is inaccessible.
Syntax of the os.path.getsize()
Method
os.path.getsize(path)
Parameters
path |
It is an address object of a file system path or a symlink. The object can either be a string or bytes. |
Return
The return type of this method is an integer value. The integer represents the size of the specified path
in bytes.
Example 1: Working With the os.path.getsize()
Method in Python
import os
path = "/home/User/Desktop/file.txt"
size = os.path.getsize(path)
print("The size, in bytes, of '%s':" % path, size)
Output:
The size, in bytes, of '/home/User/Desktop/file.txt': 243
The os.path.getsize()
method will return the size of the directory and not the size of its content.
Example 2: Handling OSError
While Using the os.path.getsize()
Method in Python
import os
import sys
path = "/home/Admin/Documents/file.txt"
try:
size = os.path.getsize(path)
except OSError:
print("The path '%s' does not exist or is inaccessible." % path)
sys.exit()
print("The size, in bytes, of '% s':" % path, size)
Output:
The path '/home/Admin/Documents/file.txt' does not exist or is inaccessible.
The bytes returned by the os.path.getsize()
method can be converted to KBs, MBs, and GBs, for a better understanding by users.
Example 3: Set the Path as Empty in the os.path.getsize()
Method in Python
import os
path = ""
size = os.path.getsize(path)
print("File size:", size)
Output:
FileNotFoundError: [Errno 2] No such file or directory: ''
An alternative to this method is using the os.stat()
Python function. It returns some information about the file path, which includes the size of the path.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn