Python os.getcwdb() Method
-
Understanding Python
os.getcwdb()
Method -
Syntax of Python
os.getcwdb()
Method -
Example 1: Use
os.getcwdb()
to Get the Current Working Directory - Example 2: File and Directory Operations
- Example 3: Cross-Platform Compatibility
- Example 4: Working With Non-Unicode File Systems
- Example 5: Integration With External Commands
- Conclusion
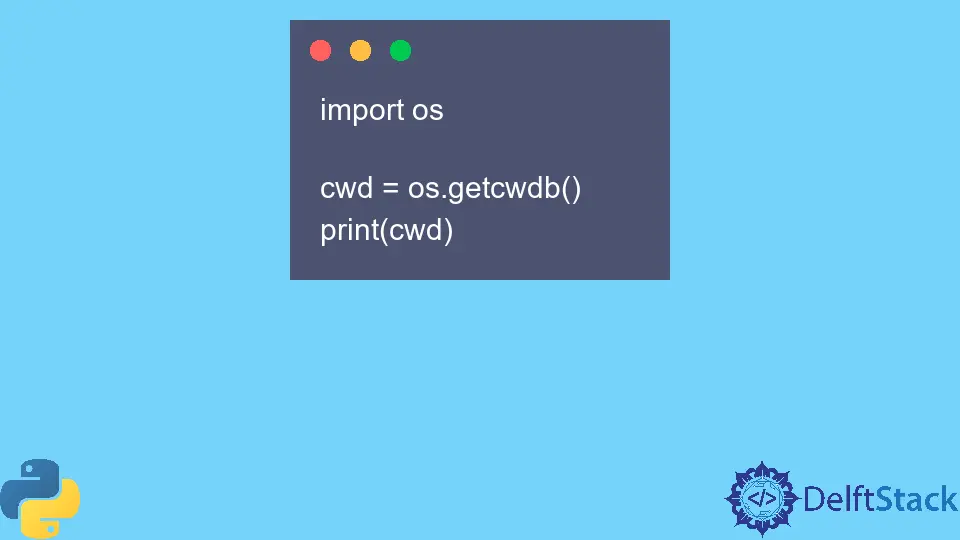
In the world of Python, dealing with file systems and directories is a common task for developers. Python provides a powerful and versatile module, os
, which includes a variety of functions for interacting with the operating system. One such function, os.getcwdb()
, plays a crucial role in obtaining the current working directory in a platform-independent manner.
The getcwdb()
method is available in the os
module in the Python programming language. The os
module offers utilities such as methods and system calls to interact with the operating system.
The getcwdb()
method returns a byte string representation of the current working directory. The os.getcwd()
method is similar to the getcwdb()
method but it returns a string representation.
In this article, we’ll explore the intricacies of os.getcwdb()
, understanding its functionality, use cases, and the importance it holds in file and directory management.
Understanding Python os.getcwdb()
Method
The os.getcwdb()
function belongs to the os
module, which is part of the Python Standard Library. The name of the function itself provides a hint about its purpose: “get current working directory (bytes)”.
It returns a bytes object representing the current working directory, ensuring compatibility with both Unicode and non-Unicode file systems.
The os.getcwdb()
function is an improvement over the older os.getcwd()
method, which returns the current working directory as a string. The decision to introduce os.getcwdb()
was driven by the need to handle file system paths consistently and reliably across different platforms.
Syntax of Python os.getcwdb()
Method
os.getcwdb()
Parameters
This method doesn’t accept any parameters.
Return Value
The getcwdb()
method returns a byte string representation of the current working directory. A byte string refers to a sequence of bytes, whereas a standard string is a sequence of characters.
Example 1: Use os.getcwdb()
to Get the Current Working Directory
This code snippet uses the os
module to obtain the current working directory in bytes format using the os.getcwdb()
function. The obtained bytes object represents the current working directory and is then printed to the console.
The use of os.getcwdb()
is valuable in scenarios where binary data or non-Unicode file systems are involved. If a Unicode representation is needed, the bytes can be decoded using an appropriate encoding, such as UTF-8.
import os
cwd = os.getcwdb()
print(cwd)
Output:
b'/home/user/python'
Example 2: File and Directory Operations
When performing file or directory operations, it is essential to have the correct working directory. os.getcwdb()
becomes particularly useful when constructing absolute paths for file manipulations.
import os
current_dir_bytes = os.getcwdb()
current_dir_str = current_dir_bytes.decode("utf-8")
file_path = os.path.join(current_dir_str, "example.txt")
with open(file_path, "wb") as file:
file.write(b"Hello, os.getcwdb()!")
Output in example.txt
:
Hello, os.getcwdb()!
This code obtains the current working directory in bytes, decodes it to a string using UTF-8 encoding, constructs a file path by joining the current directory and a filename, and then writes a binary message to a file named 'example.txt'
in the current working directory.
The code ensures compatibility with different file systems and uses encoding to represent the directory in a human-readable format.
Example 3: Cross-Platform Compatibility
Different operating systems handle file paths and encodings differently. The use of bytes in os.getcwdb()
ensures cross-platform compatibility, making it suitable for applications that need to run on various systems.
import os
current_dir_bytes = os.getcwdb()
print("Current working directory:", current_dir_bytes.decode("utf-8"))
Output:
Current working directory: /home
Example 4: Working With Non-Unicode File Systems
In some scenarios, file systems may not be Unicode-aware. os.getcwdb()
ensures that the working directory is represented as bytes, making it suitable for environments where Unicode encoding is not guaranteed.
import os
current_dir_bytes = os.getcwdb()
print("Working directory as bytes:", current_dir_bytes)
Output:
Working directory as bytes: b'/home'
Example 5: Integration With External Commands
In cases where Python scripts need to call external commands or tools that expect byte-encoded paths, os.getcwdb()
provides a seamless way to obtain the necessary working directory information.
import os
import subprocess
current_dir_bytes = os.getcwdb()
subprocess.run(["ls", current_dir_bytes])
Output:
example.txt main.py
On Windows, you might use the dir
command:
import os
import subprocess
current_dir_bytes = os.getcwdb()
subprocess.run(["dir", current_dir_bytes])
Replace 'ls'
or 'dir'
with the actual command you want to run. If the command requires additional arguments, you can include them as additional elements in the list.
Keep in mind that the behavior of the external command will depend on the operating system you are using. The examples provided are based on Unix-like systems and Windows, but you should adjust the command accordingly if you are working on a different platform.
Conclusion
In conclusion, the os.getcwdb()
method in Python’s os
module serves as a valuable tool for obtaining the current working directory in a bytes format. This function ensures consistency in handling file paths across different platforms and supports scenarios where Unicode encoding may not be guaranteed.
By using os.getcwdb()
, developers can write robust and platform-independent code when dealing with file and directory operations. As Python continues to evolve, understanding and leveraging features like os.getcwdb()
become essential for building applications that seamlessly interact with the underlying operating system.