Python os.fsencode() Method
-
Syntax of Python
os.fsencode()
Method -
Example 1: Use
os.fsencode()
to Encode File System Paths of 3 Operating Systems -
Example 2: Use
os.fsencode()
to Encode a Unicode File Name in Python - Example 3: Encoding a File Path
- Example 4: Encoding a File Name Stored in a Variable
- Example 5: Handling Invalid Characters
- Usage and Understanding
- Conclusion
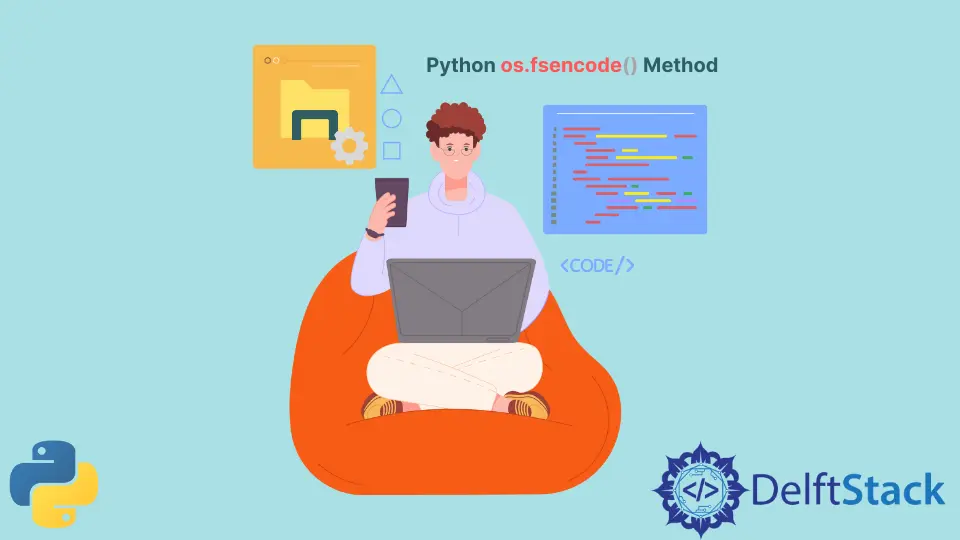
The os.fsencode()
method is a part of the os
module in Python, primarily used to encode file system names or paths from Unicode strings into bytes. This function is particularly useful when dealing with file system operations that require byte-encoded file names or paths, ensuring compatibility and correct representation across different platforms.
The fsencode()
method is a part of the os
module available in Python. The fsencode()
method encodes the passed filename to file system encoding.
The encoded filename can be decoded back with the help of the fsdecode()
method.
Syntax of Python os.fsencode()
Method
os.fsencode(filename)
Parameters
Type | Description | |
---|---|---|
filename |
string/byte string | A string representing a file name or path as a Unicode string. |
Return Value
The os.fsencode()
method returns the file name encoded as bytes using the system’s filesystem encoding.
Example 1: Use os.fsencode()
to Encode File System Paths of 3 Operating Systems
This example demonstrates the use of os.fsencode()
to convert different types of file paths (both string and byte string representations) from various operating systems into their corresponding byte representations. It covers file paths for Linux, Windows, and MacOS environments.
For instance:
f1
,f2
, andf3
are strings representing paths for Linux, Windows, and MacOS, respectively.f4
,f5
, andf6
are byte strings representing paths for Linux, Windows, and MacOS, respectively.
The os.fsencode()
function is applied to each path variable, attempting to encode them into byte representations suitable for the filesystem. The output will display the encoded byte strings for the respective paths provided.
import os
f1 = "/home/user/Photos/School-Trip.zip" # Linux path (string)
f2 = "D:\\User\\Photos\\School-Trip.zip" # Windows path (string)
f3 = "/Users/Username/Desktop/Photos/School-Trip.zip" # MacOS path (string)
f4 = b"/home/user/Photos/School-Trip.zip" # Linux path (bytes string)
f5 = b"D:\User\Photos\School-Trip.zip" # Windows path (bytes string)
# MacOS path (bytes string)
f6 = b"/Users/Username/Desktop/Photos/School-Trip.zip"
print(os.fsencode(f1))
print(os.fsencode(f2))
print(os.fsencode(f3))
print(os.fsencode(f4))
print(os.fsencode(f5))
print(os.fsencode(f6))
Output:
b'/home/user/Photos/School-Trip.zip'
b'D:\\User\\Photos\\School-Trip.zip'
b'/Users/Username/Desktop/Photos/School-Trip.zip'
b'/home/user/Photos/School-Trip.zip'
b'D:\\User\\Photos\\School-Trip.zip'
b'/Users/Username/Desktop/Photos/School-Trip.zip'
With the help of the fsencode()
method, the Python code above encodes 6
file system paths. Out of the 6
paths, 3
paths are strings, and the remaining are byte strings.
Moreover, file system paths of Linux, Windows, and macOS operating systems are selected.
Example 2: Use os.fsencode()
to Encode a Unicode File Name in Python
The os.fsencode()
method in Python is used to convert a Unicode file name (a string representing the name of a file that might contain characters from various languages) into a bytes object that can be used as a file name in the file system.
Let’s explore how os.fsencode()
operates with an example:
import os
# Unicode file name
file_name_unicode = "some_file_é.txt"
# Encoding the Unicode file name using fsencode
file_name_encoded = os.fsencode(file_name_unicode)
print(f"Encoded File Name: {file_name_encoded}")
Output:
Encoded File Name: b'some_file_\xc3\xa9.txt'
The code illustrates the use of os.fsencode()
in Python, encoding a Unicode file name into a byte string suitable for file system interactions. The provided example initializes a Unicode file name containing a special character ('é'
).
os.fsencode()
is then applied to encode this Unicode string into a byte string, creating an encoded representation that’s compatible with file system operations. Finally, the code displays the resulting encoded file name.
Example 3: Encoding a File Path
This example demonstrates the usage of os.fsencode()
in Python. It encodes a file path containing non-ASCII characters into a byte-encoded string.
This function is useful for scenarios where operating systems or file systems require byte-encoded strings, especially when handling non-ASCII characters.
The resulting encoded file path, stored in file_path_encoded
, showcases the byte-encoded representation of the original file path, enabling compatibility with systems or operations that demand byte-encoded strings.
import os
# File path containing a non-ASCII character
file_path = "/home/user/étudiant/file.txt"
# Encoding the file path using fsencode
file_path_encoded = os.fsencode(file_path)
print(f"Encoded File Path: {file_path_encoded}")
Output:
Encoded File Path: b'/home/user/\xc3\xa9tudiant/file.txt'
Example 4: Encoding a File Name Stored in a Variable
This example uses os.fsencode()
in Python to encode a file name containing the Greek letter alpha (α
) into a byte-encoded format. This allows handling non-ASCII characters in file names by converting them into byte-encoded strings, ensuring compatibility with systems that require byte-encoded file names.
import os
# Variable storing a file name
file_name_variable = "document_α.txt"
# Encoding the file name stored in a variable using fsencode
file_name_encoded = os.fsencode(file_name_variable)
print(f"Encoded File Name: {file_name_encoded}")
Output:
Encoded File Name: b'document_\xce\xb1.txt'
Example 5: Handling Invalid Characters
This example attempts to encode a Unicode file name containing the delta character (Δ
) using os.fsencode()
in Python. However, this delta character may not be representable or valid in the file system encoding being used.
The code utilizes a try-except
block to handle a possible UnicodeEncodeError
that might occur during the encoding process due to the presence of characters that cannot be represented in the file system’s encoding. If such an error occurs, it catches the exception and prints an error message indicating the issue.
import os
# Unicode file name with invalid characters for the file system encoding
file_name_unicode = "file_\u0394.txt" # Contains a delta character (Δ)
try:
# Attempt to encode the Unicode file name using fsencode
file_name_encoded = os.fsencode(file_name_unicode)
print(f"Encoded File Name: {file_name_encoded}")
except UnicodeEncodeError as e:
print(f"Error: {e}")
Output:
Encoded File Name: b'file_\xce\x94.txt'
Usage and Understanding
1. Encoding File Names
Different operating systems use specific encodings to represent file names in the filesystem. os.fsencode()
is used to encode Unicode file names into bytes using the filesystem’s encoding, ensuring correct representation.
2. Byte Encoding
File names that contain non-ASCII characters or special symbols can create compatibility issues. os.fsencode()
converts Unicode strings into byte-encoded file names, which are essential for some file operations.
3. Cross-Platform Compatibility
By converting Unicode strings to bytes using the system’s filesystem encoding, os.fsencode()
helps in maintaining compatibility across different platforms, ensuring that file names are correctly represented.
4. Handling Non-ASCII Characters
File names containing characters beyond the ASCII range might need special encoding for correct interpretation across filesystems. os.fsencode()
encodes these characters properly for file system operations.
5. Creating Byte-encoded File Names
Certain filesystem operations or APIs require file names in byte-encoded format rather than Unicode strings. os.fsencode()
aids in generating byte-encoded versions of file names for such operations.
6. Consistent Handling of File Names
For applications interacting with the filesystem, especially when dealing with non-ASCII characters in file names, using os.fsencode()
ensures uniform handling and representation of file names as byte-encoded values.
7. Compatibility with Older Python Versions
os.fsencode()
provides a consistent method for encoding file names into bytes, particularly useful in older Python versions during the transition from byte strings to Unicode strings.
The os.fsencode()
method in Python is a valuable tool for converting Unicode file names or paths into bytes using the system’s filesystem encoding. This functionality is crucial for cross-platform compatibility, ensuring correct and consistent representation of file names while interacting with the filesystem.
Conclusion
This comprehensive guide explores the os.fsencode()
method in Python, emphasizing its significance in encoding Unicode file names into byte-encoded representations. By providing a consistent way to generate byte-encoded file names, this method ensures compatibility and accuracy when working with file names across diverse platforms and encodings.
Understanding os.fsencode()
facilitates robust and consistent file operations in Python applications, especially when byte-encoded file names are necessary for filesystem interactions.