Python os.fsdecode() Method
-
Syntax of Python
os.fsdecode()
Method -
Example 1: Use the
os.fsdecode()
Method in Python -
Example 2: Use the
os.fsdecode()
andos.fsencode()
Methods in Python -
Example 3: Unspecified Path in the
os.fsdecode()
Method in Python - Usage and Understanding
- Conclusion
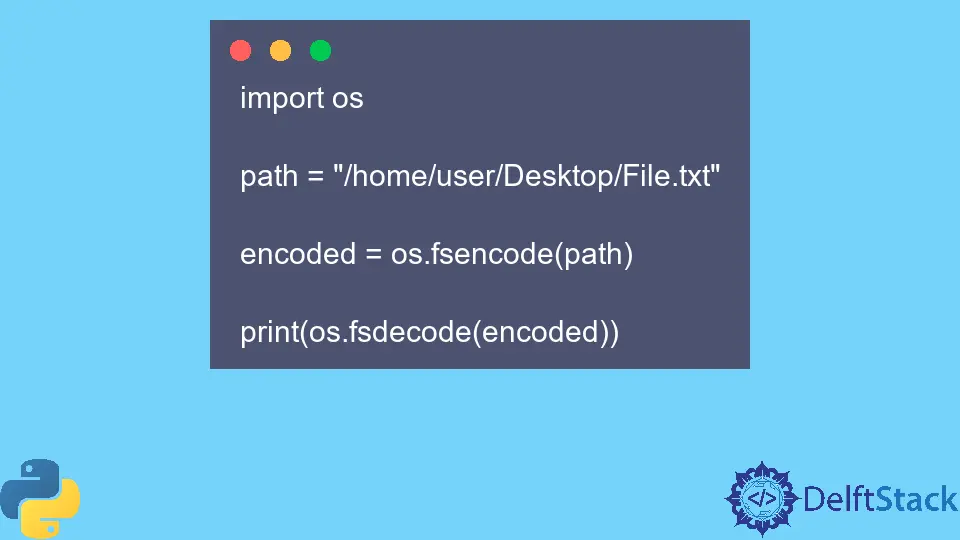
The os.fsdecode()
method is a part of the os
module in Python and is specifically designed to decode filesystem-encoded file names into Unicode strings. This function is crucial for handling file names or paths that might contain non-ASCII characters in a cross-platform manner.
Python’s os.fsdecode()
method is an efficient way of using filesystem encoding and error handlers to decode a file path. The method uses the surrogateescape
error handler and strict
on Windows OS.
Syntax of Python os.fsdecode()
Method
os.fsdecode(path)
Parameters
path |
A string representing a file name or path in the filesystem encoding. The object can either be an str or bytes. |
Return Value
The os.fsdecode()
method returns the filename converted to a string using the system’s filesystem encoding.
Example 1: Use the os.fsdecode()
Method in Python
This example showcases the utilization of os.fsdecode()
in Python to decode a filename represented as a byte string using the filesystem encoding. It takes a byte-encoded file name (file_name_encoded
) and utilizes os.fsdecode()
to convert it into a human-readable string format (file_name_decoded
).
The resulting decoded filename, "some_file_é.txt"
, is then printed as "Decoded File Name: some_file_é.txt"
. This highlights how os.fsdecode()
facilitates the conversion of byte-encoded filenames into more accessible string representations, essential when working with filenames across diverse filesystem encodings in Python.
import os
# File name encoded with filesystem encoding (example for Windows)
file_name_encoded = b"some_file_\xc3\xa9.txt"
# Decoding the encoded file name using fsdecode
file_name_decoded = os.fsdecode(file_name_encoded)
print(f"Decoded File Name: {file_name_decoded}")
Output:
Decoded File Name: some_file_é.txt
The operating system is responsible for encoding and decoding data. The OS
module of Python enables the user to interact with the OS smoothly.
Example 2: Use the os.fsdecode()
and os.fsencode()
Methods in Python
This example uses os.fsencode()
to encode a string path into a bytes object using the filesystem’s encoding and then reverses the encoding using os.fsdecode()
to retrieve the original string path. Ultimately, it confirms that the encoding and subsequent decoding retain the original string format.
import os
path = "/home/user/Desktop/File.txt"
encoded = os.fsencode(path)
print(os.fsdecode(encoded))
Output:
/home/user/Desktop/File.txt
The print()
statement outputs the decoded path after encoding and decoding, confirming that the encoding and subsequent decoding using os.fsencode()
and os.fsdecode()
return the original string path. The reverse method for the os.fsdecode()
method is the os.fsencode()
.
Example 3: Unspecified Path in the os.fsdecode()
Method in Python
This example attempts to encode an empty string representing a path using os.fsencode()
. In most cases, when an empty string is passed for encoding, it generates an empty bytes object (b''
).
Then, it uses os.fsdecode()
to decode the empty bytes object back to a string, which would result in an empty string as well. Both the encoded and decoded outputs here are expected to be empty.
import os
path = ""
encoded = os.fsencode(path)
print(encoded)
print(os.fsdecode(encoded))
Output:
b''
The os.fsdecode()
method converts the Unicode to a byte string.
Usage and Understanding
1. Filesystem Encoding
Different operating systems use specific encodings to represent file names in the filesystem. These encodings can include ASCII or non-ASCII characters, and handling them uniformly across platforms is essential for cross-platform compatibility.
2. Decoding File Names
The os.fsdecode()
method decodes the file name bytes encoded in the filesystem encoding into a Unicode string. It ensures that the file names with non-ASCII characters are correctly decoded and presented as readable strings.
3. Handling Non-ASCII Characters
File names containing non-ASCII characters (such as accented characters or symbols) can be problematic when dealing with different filesystems or platforms. os.fsdecode()
aids in properly handling these characters by decoding the encoded byte sequences.
4. Cross-Platform File Handling
For applications working with files across multiple operating systems, using os.fsdecode()
ensures consistent and accurate handling of file names encoded in different filesystem encodings. It assists in maintaining portability and compatibility.
5. Unicode Strings Representation
The method returns the file name as a Unicode string, enabling developers to work with file names uniformly as strings. This Unicode representation is vital for applications that need to process file names regardless of their encoding.
6. Robustness and Error Handling
When working with file names that may contain non-ASCII characters, using os.fsdecode()
helps prevent decoding errors and ensures the correct interpretation of the file names across various platforms.
7. Compatibility with Older Python Versions:
os.fsdecode()
is especially useful in older Python versions (Python 2 to 3 transition) when dealing with byte strings representing file names, providing a consistent way to decode these names into Unicode strings.
The os.fsdecode()
method is an essential tool for working with file names and paths, particularly in scenarios where file names might contain non-ASCII characters encoded according to the filesystem conventions. Understanding and utilizing this method is crucial for robust and cross-platform file handling in Python.
Conclusion
This comprehensive guide delves into the os.fsdecode()
method in Python, emphasizing its significance in handling filesystem-encoded file names. By decoding file names into Unicode strings, this method facilitates uniformity, compatibility, and error-free operations when working with file names across diverse operating systems and encodings.
Understanding os.fsdecode()
enables developers to handle file names robustly and uniformly in their Python applications, ensuring seamless cross-platform file operations.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn