Python os.dup() Method
-
Syntax of Python
os.dup()
: -
Example Code 1: Use the
os.dup()
Method to Duplicate a File Descriptor -
Example Code 2: Use the
os.dup()
Method to Duplicate a File Descriptor and Create a New File Object -
Example Code 3:
OSError: [Errno 9] Bad file descriptor
in Using theos.dup()
Method to Duplicate a File Descriptor - Conclusion
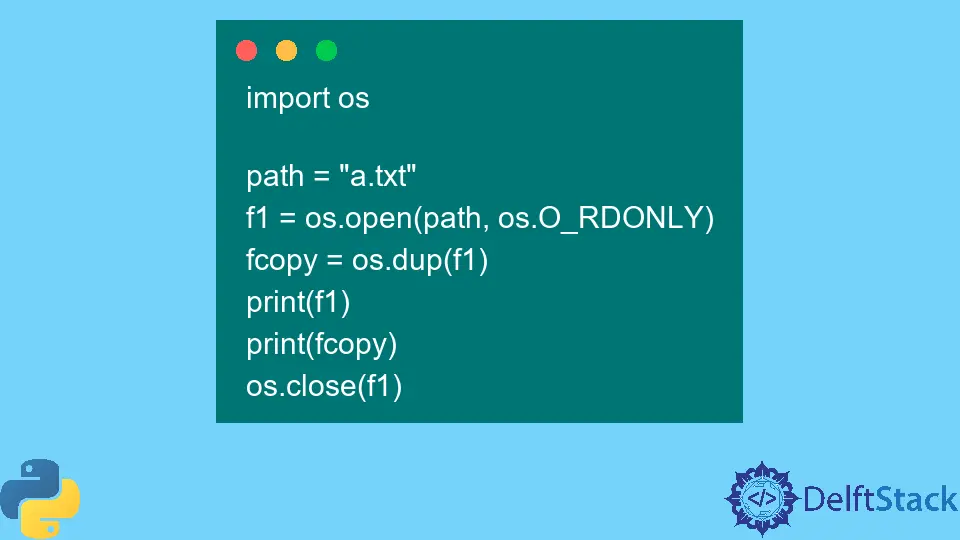
The dup()
method belongs to the os
module. The os
module contains implementations for various functions to communicate and work with the operating system. The dup()
method returns a duplicate for a file descriptor.
When working with processes in Python, you may encounter situations where you need to share file descriptors between different processes or redirect input/output streams. This is where os.dup()
becomes valuable.
By duplicating file descriptors, you can have multiple processes communicate or manipulate the same file or other I/O streams independently.
Syntax of Python os.dup()
:
os.dup(fd)
Parameter
Parameter | Type | Description |
---|---|---|
fd |
integer | A file descriptor associated with a valid resource. |
Return
The dup()
method returns a duplicate for a file descriptor. Note that the new file descriptor is non-inheritable. Non-inheritable means that the file descriptor created can not be inherited by the child processes. In child processes, all the non-inheritable file descriptors are closed.
Example Code 1: Use the os.dup()
Method to Duplicate a File Descriptor
In this example code, let’s explore a concise yet powerful code snippet that employs os.dup()
to duplicate a file descriptor.
a.txt
:
Sample text.
Example code:
import os
# Specify the path to the file to be opened
path = "a.txt"
# Open the file for reading and obtain its file descriptor (f1)
f1 = os.open(path, os.O_RDONLY)
# Duplicate the file descriptor (f1) to create a new one (fcopy)
fcopy = os.dup(f1)
# Print the original and duplicated file descriptors
print(f"Original file descriptor (f1): {f1}")
print(f"Duplicated file descriptor (fcopy): {fcopy}")
# Close the original file descriptor (f1)
os.close(f1)
The code snippet above starts with importing the os
module to use its functions.
Then, it uses os.open()
to open the file a.txt
in read-only mode (os.O_RDONLY
). This returns a file descriptor (f1
), a low-level integer representing the open file.
Next, it utilizes os.dup()
to duplicate the original file descriptor (f1
), creating a new file descriptor (fcopy
) that points to the same open file.
Before closing with os.close(f1)
, it will display both file descriptors (f1
and fcopy
) to provide visibility into the unique integer values associated with each file descriptor.
Output:
As we can conclude from the output, the two file descriptors are different, and the dup()
method created a hard copy of the original file descriptor.
The code demonstrates how to open a file, duplicate its file descriptor, print the descriptors, and finally close the original file descriptor. Understanding this process is fundamental when working with file operations and inter-process communication in Python.
Example Code 2: Use the os.dup()
Method to Duplicate a File Descriptor and Create a New File Object
The code below demonstrates the use of the os.dup()
function in Python to duplicate a file descriptor and create a new file object associated with the duplicated descriptor.
sample.txt
:
Hello, World!
Example code:
import os
file_path = "sample.txt"
# Open the file for reading
with open(file_path, "r") as original_file:
# Get the file descriptor of the original file
original_fd = original_file.fileno()
# Duplicate the file descriptor
new_fd = os.dup(original_fd)
# Create new file object using the duplicated file descriptor
new_file_object = os.fdopen(new_fd, "r")
# Read and print content from the original file object
print("Original file content:")
print(original_file.read())
# Reset the file position of the duplicated file object
new_file_object.seek(0)
# Read and print content from the duplicated file object
print("Duplicated file content:")
print(new_file_object.read())
# Close the file objects
original_file.close()
# Close the duplicated file descriptor
os.close(new_fd)
The Python code above focuses on the reading and duplication of a file’s content using the os.dup()
function.
It begins by specifying the path to the file ('example.txt'
) to be read. The file is opened for reading within a context manager to ensure proper closure after use.
The original file’s descriptor is obtained using original_file.fileno()
, and os.dup(original_fd)
duplicates this descriptor, creating a new one (new_fd
).
Using os.fdopen(new_fd, 'r')
, a new file object (new_file_object
) is created, allowing independent reading from the same file. The code then reads and prints the content of both the original and duplicated file objects.
In order to manage system resources effectively, both file objects are explicitly closed using close()
, and the duplicated file descriptor (new_fd
) is closed using os.close(new_fd)
.
Output:
The output will show the content of both the original and duplicated file objects is successfully read and printed.
The os.dup()
operation allows independent access to the same file content through separate file objects.
Example Code 3: OSError: [Errno 9] Bad file descriptor
in Using the os.dup()
Method to Duplicate a File Descriptor
This example code demonstrates an OSError: [Errno 9] Bad file descriptor
encounter where it attempts to redirect standard output to a file using os.dup()
and later reset it to the original file descriptor.
The primary purpose is to showcase how improper handling of file descriptors can lead to errors.
sample.txt
:
Hello, World!
Example code:
import os
# Open a file for writing
output_file_path = "sample.txt"
with open(output_file_path, "w") as output_file:
# Get the file descriptor of the file
file_fd = output_file.fileno()
# Duplicate the file descriptor
new_file_fd = os.dup(file_fd)
# Save the original stdout file descriptor
original_stdout_fd = 1
try:
# Redirect standard output to the file by closing and duplicating
os.close(1)
os.dup(new_file_fd)
# Now, print statements will write to the output file
print("This is written to the file.")
finally:
# Reset standard output to the original file descriptor by closing and duplicating
os.close(1)
os.dup(original_stdout_fd)
# Close the duplicated file descriptor
os.close(new_file_fd)
# The standard output is now back to the original state
print("This is the final message.")
In summary, the code opens a file named "sample.txt"
in write mode, duplicates its file descriptor, and attempts to redirect standard output to the file.
The original standard output descriptor is saved before the redirection. A print
statement writes to the redirected output.
However, an error occurs during the reset process, resulting in a "Bad file descriptor"
error.
Finally, the duplicated file descriptor is closed, and a message is printed to the original standard output outside the context block.
Output:
The error OSError: [Errno 9] Bad file descriptor
occurs because after duplicating the file descriptor (new_file_fd
), you close the standard output file descriptor (1
) using os.close(1)
. This causes the standard output file descriptor to become invalid or “bad.”
Conclusion
In conclusion, the os.dup()
method in Python, residing in the os
module, serves a crucial role in handling file descriptors.
When working with processes or redirecting input/output streams, os.dup()
becomes invaluable, allowing multiple processes to manipulate the same file independently.
The syntax is simple, taking a file descriptor as a parameter and returning a non-inheritable duplicate. The provided examples illustrate its usage in duplicating file descriptors and creating new file objects.
It is essential to handle file descriptors carefully to prevent errors, as demonstrated in the intentional error-triggering example.
Understanding os.dup()
is fundamental for efficient file descriptor management and inter-process communication in Python.
To explore more similar to the topic os.dup()
method, you can visit os.dup2()
.