Pandas DataFrame.std() Function
Minahil Noor
Jan 30, 2023
-
Syntax of
pandas.DataFrame.std()
: -
Example Codes:
DataFrame.std()
Method to Calculate Standard Deviation Along Row Axis -
Example Codes:
DataFrame.std()
Method to Calculate Standard Deviation Along Column Axis
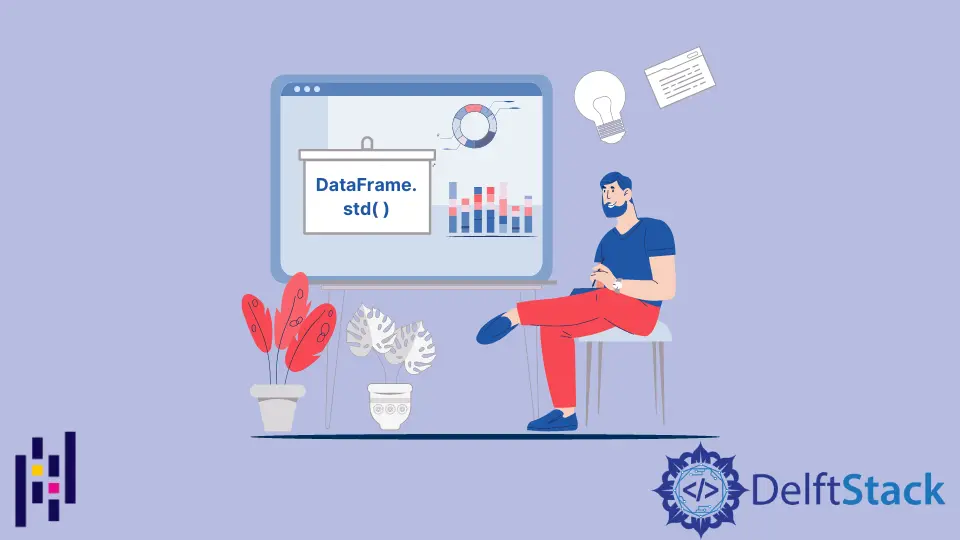
Python Pandas DataFrame.std()
function calculates the standard deviation of numeric columns or rows of a DataFrame
Syntax of pandas.DataFrame.std()
:
DataFrame.std(axis=None, skipna=None, level=None, ddof=1, numeric_only=None, **kwargs)
Parameters
axis |
It is an integer or string type parameter. It specifies the axis over which the standard deviation will be calculated. |
skipna |
It is a Boolean parameter. This parameter tells about excluding null values. If an entire row or column is null, the result will be NA. |
level |
It is an integer or string type parameter. It counts along a particular level if the axis is MultiIndex. |
ddof |
It is an integer parameter. It stands for Delta Degrees of Freedom. The divisor used in calculations is N - ddof, where N represents the number of elements. |
numeric_only |
It is a Boolean parameter. If set to True , then the function includes only float, int, boolean columns, or rows. |
**kwargs |
These are the additional keyword arguments. |
Return
It returns the Series
or Dataframe.
Example Codes: DataFrame.std()
Method to Calculate Standard Deviation Along Row Axis
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.std(axis= 0)
print("The Standard Deviation is: \n")
print(dataframe1)
Output:
The Original Data frame is:
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
The Standard Deviation is:
Attendance 15.773395
Obtained Marks 17.484279
dtype: float64
The function has returned the calculated values of standard deviations.
Example Codes: DataFrame.std()
Method to Calculate Standard Deviation Along Column Axis
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.std(axis= 1)
print("The Standard Deviation is: \n")
print(dataframe1)
Output:
The Original Data frame is:
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
The Standard Deviation is:
0 21.213203
1 17.677670
2 1.414214
3 9.899495
4 35.355339
dtype: float64
The function has returned the calculated values of standard deviations over the column axis.