Pandas DataFrame DataFrame.assign() Function
-
Syntax of
pandas.DataFrame.assign()
: -
Example Codes:
DataFrame.assign()
Method to Assign a Single Column -
Example Codes:
DataFrame.assign()
Method to Assign Multiple Columns
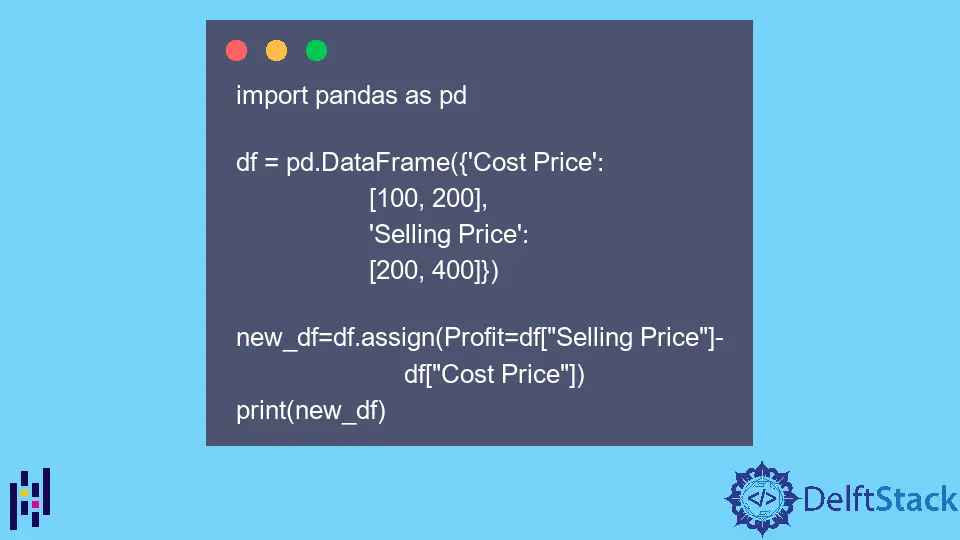
Python Pandas DataFrame.assign()
function assigns new columns to the DataFrame
.
Syntax of pandas.DataFrame.assign()
:
DataFrame.assign(**kwargs)
Parameters
**kwargs |
keyword arguments to the assign() function. The column names to be assigned to DataFrame are passed as keyword arguments. |
Return
It returns the DataFrame
object with new columns assigned along with existing columns.
Example Codes: DataFrame.assign()
Method to Assign a Single Column
import pandas as pd
df = pd.DataFrame({'Cost Price':
[100, 200],
'Selling Price':
[200, 400]})
new_df=df.assign(Profit=df["Selling Price"]-
df["Cost Price"])
print(new_df)
The caller DataFrame
is
Cost Price Selling Price
0 100 200
1 200 400
Output:
Cost Price Selling Price Profit
0 100 200 100
1 200 400 200
It assigns a new column Profit
to the Dataframe
which corresponds to the difference between columns Selling Price
and Cost Price
.
We can also assign a new column to df
by using the lambda
function for callable objects.
import pandas as pd
df = pd.DataFrame({'Cost_Price':
[100, 200],
'Selling_Price':
[200, 400]})
new_df=df.assign(Profit=lambda x:
x.Selling_Price-
x.Cost_Price)
print(new_df)
The caller DataFrame
is
Cost Price Selling Price
0 100 200
1 200 400
Output:
Cost_Price Selling_Price Profit
0 100 200 100
1 200 400 200
Example Codes: DataFrame.assign()
Method to Assign Multiple Columns
import pandas as pd
df = pd.DataFrame({'Cost_Price':
[100, 200],
'Selling_Price':
[200, 400]})
new_df=df.assign(Cost_Price_Euro =
df['Cost_Price']*1.11,
Selling_Price_Euro =
df['Selling_Price']*1.11)
print(new_df)
The caller DataFrame
is
Cost Price Selling Price
0 100 200
1 200 400
Output:
Cost_Price Selling_Price Cost_Price_Euro Selling_Price_Euro
0 100 200 111.0 222.0
1 200 400 222.0 444.0
It assigns two new columns Cost_Price_Euro
and Selling_Price_Euro
to df
which are derived from existing columns Cost_Price
and Selling_Price
respectively.
We can also assign multiple columns to df
using the lambda
function for callable objects.
import pandas as pd
df = pd.DataFrame({'Cost_Price':
[100, 200],
'Selling_Price':
[200, 400]})
new_df=df.assign(Cost_Price_Euro =
lambda x: x.Cost_Price*1.11,
Selling_Price_Euro =
lambda x: x.Selling_Price*1.11)
print(new_df)
The caller DataFrame
is
Cost Price Selling Price
0 100 200
1 200 400
Output:
Cost_Price Selling_Price Cost_Price_Euro Selling_Price_Euro
0 100 200 111.0 222.0
1 200 400 222.0 444.0
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn