Pandas DataFrame DataFrame.assign() 함수
Suraj Joshi
2023년1월30일
Pandas
Pandas DataFrame
-
pandas.DataFrame.assign()
의 구문: -
예제 코드: 단일 열을 할당하는
DataFrame.assign()
메서드 -
예제 코드: 여러 열을 할당하는
DataFrame.assign()
메서드
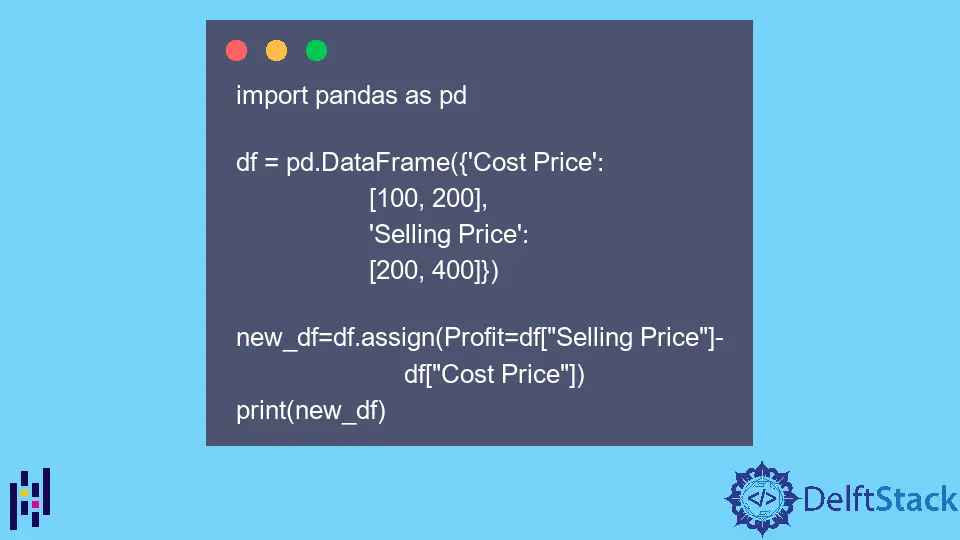
Python Pandas DataFrame.assign()
함수는DataFrame
에 새 열을 할당합니다.
pandas.DataFrame.assign()
의 구문:
DataFrame.assign(**kwargs)
매개 변수
**kwargs |
assign() 함수에 대한 키워드 인수. DataFrame 에 할당 할 열 이름은 키워드 인수로 전달됩니다. |
반환
기존 열과 함께 새 열이 할당 된DataFrame
개체를 반환합니다.
예제 코드: 단일 열을 할당하는DataFrame.assign()
메서드
import pandas as pd
df = pd.DataFrame({'Cost Price':
[100, 200],
'Selling Price':
[200, 400]})
new_df=df.assign(Profit=df["Selling Price"]-
df["Cost Price"])
print(new_df)
호출자DataFrame
은
Cost Price Selling Price
0 100 200
1 200 400
출력:
Cost Price Selling Price Profit
0 100 200 100
1 200 400 200
Selling Price
와Cost Price
열의 차이에 해당하는Dataframe
에 새 열Profit
을 할당합니다.
호출 가능한 객체에lambda
함수를 사용하여df
에 새 열을 할당 할 수도 있습니다.
import pandas as pd
df = pd.DataFrame({'Cost_Price':
[100, 200],
'Selling_Price':
[200, 400]})
new_df=df.assign(Profit=lambda x:
x.Selling_Price-
x.Cost_Price)
print(new_df)
호출자DataFrame
은
Cost Price Selling Price
0 100 200
1 200 400
출력:
Cost_Price Selling_Price Profit
0 100 200 100
1 200 400 200
예제 코드: 여러 열을 할당하는DataFrame.assign()
메서드
import pandas as pd
df = pd.DataFrame({'Cost_Price':
[100, 200],
'Selling_Price':
[200, 400]})
new_df=df.assign(Cost_Price_Euro =
df['Cost_Price']*1.11,
Selling_Price_Euro =
df['Selling_Price']*1.11)
print(new_df)
호출자DataFrame
은
Cost Price Selling Price
0 100 200
1 200 400
출력:
Cost_Price Selling_Price Cost_Price_Euro Selling_Price_Euro
0 100 200 111.0 222.0
1 200 400 222.0 444.0
기존 열Cost_Price
및Selling_Price
에서 파생 된df
에 각각 두 개의 새 열Cost_Price_Euro
및Selling_Price_Euro
를 할당합니다.
호출 가능한 객체에 대해lambda
함수를 사용하여df
에 여러 열을 할당 할 수도 있습니다.
import pandas as pd
df = pd.DataFrame({'Cost_Price':
[100, 200],
'Selling_Price':
[200, 400]})
new_df=df.assign(Cost_Price_Euro =
lambda x: x.Cost_Price*1.11,
Selling_Price_Euro =
lambda x: x.Selling_Price*1.11)
print(new_df)
호출자DataFrame
은
Cost Price Selling Price
0 100 200
1 200 400
출력:
Cost_Price Selling_Price Cost_Price_Euro Selling_Price_Euro
0 100 200 111.0 222.0
1 200 400 222.0 444.0
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
작가: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn